本关任务:编写一个能实现双色球选号的小程序。 相关知识 为了完成本关任务,你需要掌握:1.条件语句,2.列表操作,3.循环语句,4函数定义及调用。 编程要求 根据提示,在右侧编辑器补充代码,编写一个能实现双色球选号的小程序。 双色球选号由7个数字组成y,其中有6个红球,其号码的取值范围为[1,33],一个蓝球的取值范围为[1,16],要求6个红球从小到大排列,蓝球在最后输出。其输出格式为09 12 16 20 30 33 | 03。(注意,如双色球号码为3,则必须输出03) 测试说明 平台会对你编写的代码进行测试: 测试输入:7; 输入说明:选7注 预期输出: 09 12 16 20 30 33 | 03 01 07 08 09 18 31 | 16 05 08 21 26 28 31 | 05 01 03 06 22 25 33 | 02 02 09 16 20 27 28 | 13 15 19 24 26 28 32 | 05 02 05 07 16 24 32 | 09 输出说明:生成7注双色球号。|后面的数字为蓝球的号码 提示:等概率的生成一组无重复的数,可以采用sample函数,其案例如下 如要在1-10中等概率抽取6位数,代码如下 from random import sample t = [x for x in range(1, 11)] print(sample(t,6))
时间: 2023-06-11 14:07:45 浏览: 1228
```python
from random import sample
def select_nums():
red_balls = sorted(sample(range(1, 34), 6))
blue_ball = sample(range(1, 17), 1)[0]
return red_balls + [blue_ball]
def print_nums(n):
for i in range(n):
nums = select_nums()
print(" ".join(f"{num:0>2}" for num in nums[:6]), end=" ")
print("|", end=" ")
print(f"{nums[-1]:0>2}")
n = int(input())
print_nums(n)
```
相关问题
python编写一个能实现双色球选号的小程序。
在Python中编写一个简单的双色球选号小程序,你可以创建一个函数,让用户输入他们想要选择的红球和蓝球号码。这里是一个基础示例:
```python
import random
def generate_lottery_numbers():
# 红球范围从1到33
red_balls = list(range(1, 34))
# 蓝球范围从1到16
blue_ball = list(range(1, 17))
def select_numbers(num):
return random.sample(red_balls, num)
# 用户可以选择6个红球和1个蓝球
print("请输入你要选择的6个红球(用逗号分隔):")
red_selection = input().split(",")
red_numbers = [int(n) for n in red_selection]
print("请输入你要选择的蓝球号码:")
blue_number = int(input())
# 验证并生成蓝球
while blue_number < 1 or blue_number > 16:
print("蓝球号码需要在1到16之间,请重新输入:")
blue_number = int(input())
selected_numbers = {
"红球": red_numbers,
"蓝球": blue_number
}
return selected_numbers
# 使用函数并打印结果
selected = generate_lottery_numbers()
print(f"你选择了:红球{selected['红球']}, 蓝球 {selected['蓝球']}")
根据提示,在右侧编辑器补充代码,编写一个能实现双色球选号的小程序。 双色球选号由7个数字组成y,其中有6个红球,其号码的取值范围为[1,33],一个蓝球的取值范围为[1,16],要求6个红球从小到大排列,蓝球在最后输出。其输出格式为09 12 16 20 30 33 | 03。(注意,如双色球号码为3,则必须输出03)
```python
import random
# 生成红球号码
red_balls = random.sample(range(1, 34), 6)
red_balls.sort()
# 生成蓝球号码
blue_ball = random.randint(1, 16)
# 格式化输出双色球号码
red_balls_str = ' '.join(['{:02d}'.format(ball) for ball in red_balls])
blue_ball_str = '{:02d}'.format(blue_ball)
print(red_balls_str, '|', blue_ball_str)
```
代码说明:
1. 使用 random.sample 函数生成 6 个不重复的红球号码,取值范围为 [1, 33]。
2. 使用列表的 sort 方法将红球号码从小到大排序。
3. 使用 random.randint 函数生成一个蓝球号码,取值范围为 [1, 16]。
4. 使用列表推导式和字符串的 join 方法,将红球号码转换为字符串,并在数字前加上 0 补齐成两位数的格式。
5. 使用字符串的 format 方法,将蓝球号码转换为字符串,并在数字前加上 0 补齐成两位数的格式。
6. 使用 print 函数输出双色球号码,格式为 “红球1 红球2 红球3 红球4 红球5 红球6 | 蓝球”。
阅读全文
相关推荐






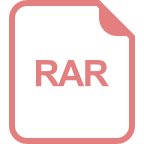
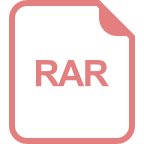

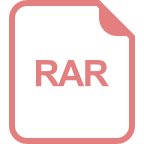
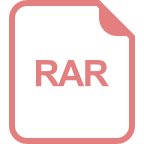


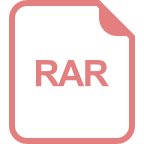
