#include <iostream> #include <string> using namespace std; enum TokenType { BEGIN, IF, THEN, WHILE, DO, END, ID, NUM, ASSIGN, PLUS, MINUS, MUL, DIV, LPAREN, RPAREN, SEMICOLON, LESS, GREATER, NEQ, LESS_EQUAL, GREATER_EQUAL, END_OF_FILE, COLON }; struct Token { TokenType type; string lexeme; }; Token currentToken; void getNextToken() { // 词法分析的代码,略去不写,将结果保存在currentToken中 } void match(TokenType expectedType) { if (currentToken.type == expectedType) { getNextToken(); } else { cout << "error" << endl; exit(1); } } void program(); void stmts(); void stmt(); void exp(); void items(); void item(); void factors(); void factor(); void program() { match(BEGIN); stmts(); match(END); } void stmts() { stmt(); while (currentToken.type == SEMICOLON) { match(SEMICOLON); stmt(); } } void stmt() { if (currentToken.type == ID) { match(ID); match(ASSIGN); exp(); } else { cout << "error" << endl; exit(1); } } void exp() { item(); items(); } void items() { if (currentToken.type == PLUS || currentToken.type == MINUS) { match(currentToken.type); item(); items(); } } void item() { factor(); factors(); } void factors() { if (currentToken.type == MUL || currentToken.type == DIV) { match(currentToken.type); factor(); factors(); } } void factor() { if (currentToken.type == ID || currentToken.type == NUM) { match(currentToken.type); } else if (currentToken.type == LPAREN) { match(LPAREN); exp(); match(RPAREN); } else { cout << "error" << endl; exit(1); } } int main() { // 词法分析部分的代码,略去不写,将结果保存在currentToken中 program(); if (currentToken.type == TokenType::END_OF_FILE) { cout << "success" << endl; } else { cout << "error" << endl; } return 0; }
时间: 2024-01-27 19:05:14 浏览: 166
这段代码中存在一个问题,就是头文件和using namespace std;之间没有加换行符,导致编译器无法识别头文件。
正确的代码应该是:
```cpp
#include <iostream>
#include <string>
using namespace std;
enum TokenType {
BEGIN, IF, THEN, WHILE, DO, END, ID, NUM, ASSIGN, PLUS, MINUS, MUL, DIV, LPAREN, RPAREN, SEMICOLON, LESS, GREATER, NEQ, LESS_EQUAL, GREATER_EQUAL, END_OF_FILE, COLON
};
struct Token {
TokenType type;
string lexeme;
};
Token currentToken;
void getNextToken() {
// 词法分析的代码,略去不写,将结果保存在currentToken中
}
void match(TokenType expectedType) {
if (currentToken.type == expectedType) {
getNextToken();
}
else {
cout << "error" << endl;
exit(1);
}
}
void program();
void stmts();
void stmt();
void exp();
void items();
void item();
void factors();
void factor();
void program() {
match(BEGIN);
stmts();
match(END);
}
void stmts() {
stmt();
while (currentToken.type == SEMICOLON) {
match(SEMICOLON);
stmt();
}
}
void stmt() {
if (currentToken.type == ID) {
match(ID);
match(ASSIGN);
exp();
}
else {
cout << "error" << endl;
exit(1);
}
}
void exp() {
item();
items();
}
void items() {
if (currentToken.type == PLUS || currentToken.type == MINUS) {
match(currentToken.type);
item();
items();
}
}
void item() {
factor();
factors();
}
void factors() {
if (currentToken.type == MUL || currentToken.type == DIV) {
match(currentToken.type);
factor();
factors();
}
}
void factor() {
if (currentToken.type == ID || currentToken.type == NUM) {
match(currentToken.type);
}
else if (currentToken.type == LPAREN) {
match(LPAREN);
exp();
match(RPAREN);
}
else {
cout << "error" << endl;
exit(1);
}
}
int main() {
// 词法分析部分的代码,略去不写,将结果保存在currentToken中
program();
if (currentToken.type == TokenType::END_OF_FILE) {
cout << "success" << endl;
}
else {
cout << "error" << endl;
}
return 0;
}
```
阅读全文
相关推荐










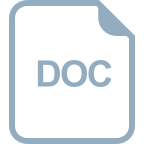

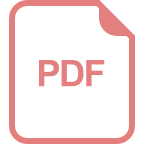




