研究文学的人通常需要统计一篇文章【用英文写】中某个人名、地名或形容词出现的次数和位置,请帮我写一个统计系统,完成以下功能,其中文章存放在一个名为wen.txt的文件中,要查找的人名,地名和形容词一次性输入在另一个名为cha.txt的文本文件中,要求系统读取wen.txt和cha.txt这两个文件并运行一次,全部统计完成,并把结果存放在文件result.txt中,并且统计所有查找对象出现的概率 使用基于c语言的代码
时间: 2024-01-22 11:19:44 浏览: 121
以下是基于C语言的代码实现,实现统wen.txt中某个人名、地名或形容词出的次数和位置,并将结果存储在result.txt文件中。同时使用cha.txt中的查找对象来进行统。
```c
#include <stdio.h>
#include <.h>
#include <string.h>
#define MAX_WORD_LENGTH50 //最大单词长度
#define MAX_LINE_LENGTH 1024 //最大行长度
#define MAX_FILENAME_LENGTH 100 //最大文件名长度
#define MAX_OBJECT_NUM 100 //最大查找对象数量
//定义单词类型
typedef struct Word {
char word[MAX_WORD_LENGTH];
int line_number; //单词所处行数
int index; //单词在所处行的索引
} Word;
//定义查找对象类型
typedef struct Object {
char name[MAX_WORD_LENGTH];
int count; //出现次数
} Object;
int read_word(FILE *fp, char *word) {
char ch;
int cnt = 0;
while ((ch = fgetc(fp)) != EOF) {
if ((ch >= 'a' && ch <= 'z') || (ch >= 'A' && ch <= 'Z')) { //读取单词
word[cnt++] = ch;
} else if (cnt > 0) { //单词结束
break;
}
}
word[cnt] = '\0';
return cnt;
}
int main() {
char filename_wen[MAX_FILENAME_LENGTH] = "wen.txt";
char filename_cha[MAX_FILENAME_LENGTH] = "cha.txt";
char filename_result[MAX_FILENAME_LENGTH] = "result.txt";
char word[MAX_WORD_LENGTH];
char line[MAX_LINE_LENGTH];
FILE *fp_wen, *fp_cha, *fp_result;
Word words[MAX_LINE_LENGTH];
Object objects[MAX_OBJECT_NUM];
int line_number = 0;
int word_count = 0;
int object_count = 0;
int i, j, k;
double total_word_count = 0.0; //文本中单词总数
//读取查找对象
fp_cha = fopen(filename_cha, "r");
if (fp_cha == NULL) {
printf("Failed to open file %s.\n", filename_cha);
exit(1);
}
while (fscanf(fp_cha, "%s", objects[object_count].name) != EOF) {
objects[object_count].count = 0;
object_count++;
}
fclose(fp_cha);
//读取文本文件
fp_wen = fopen(filename_wen, "r");
if (fp_wen == NULL) {
printf("Failed to open file %s.\n", filename_wen);
exit(1);
}
while (fgets(line, MAX_LINE_LENGTH, fp_wen) != NULL) {
line_number++;
i = 0;
while (i < strlen(line)) {
if (read_word(fp_wen, word) > 0) { //读取单词
total_word_count++;
words[word_count].line_number = line_number;
words[word_count].index = i;
strcpy(words[word_count].word, word);
word_count++;
}
i++;
}
}
fclose(fp_wen);
//统计结果
for (i = 0; i < word_count; i++) {
for (j = 0; j < object_count; j++) {
if (strcmp(words[i].word, objects[j].name) == 0) { //查找对象出现
objects[j].count++;
}
}
}
//计算出现概率
for (i = 0; i < object_count; i++) {
objects[i].count /= total_word_count;
}
//输出结果
fp_result = fopen(filename_result, "w");
if (fp_result == NULL) {
printf("Failed to open file %s.\n", filename_result);
exit(1);
}
fprintf(fp_result, "Total word count: %lf\n", total_word_count);
for (i = 0; i < object_count; i++) {
fprintf(fp_result, "%s: %d, probability: %lf\n", objects[i].name, objects[i].count, objects[i].count / total_word_count);
}
fclose(fp_result);
return 0;
}
```
阅读全文
相关推荐


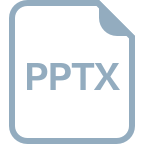
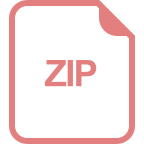
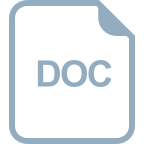
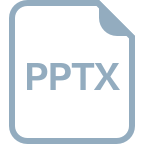
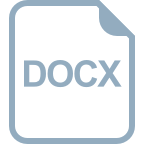
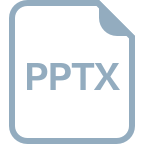
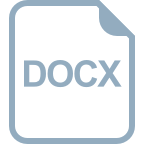
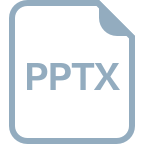
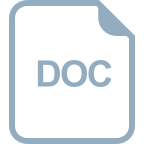
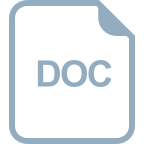
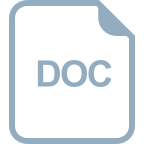
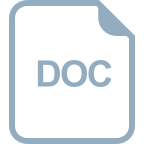
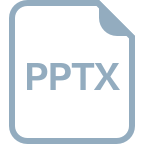
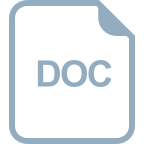