定义一个类,可以对输入的文章进行统计,要求实现以下几个方法: 1. 统计出各个单词出现的次数和频率 2. 查看出现频率最多的前10个单词 3. 输入单词能够得到单词的出现次数和频率
时间: 2023-03-19 12:25:34 浏览: 143
好的,我明白了。以下是对您的问题的回答:
可以定义一个类来对输入的文章进行统计,实现以下几个方法:
```python
class ArticleStatistics:
def __init__(self):
self.word_count = {}
self.total_word_count = 0
def process_text(self, text):
words = text.split()
for word in words:
self.word_count[word] = self.word_count.get(word, 0) + 1
self.total_word_count += 1
def get_word_count(self, word):
count = self.word_count.get(word, 0)
return count
def get_word_frequency(self, word):
frequency = self.word_count.get(word, 0) / self.total_word_count
return frequency
def get_top_n_words(self, n):
sorted_words = sorted(self.word_count.items(), key=lambda x: x[1], reverse=True)
top_n_words = sorted_words[:n]
return top_n_words
```
上述代码中,我们定义了一个`ArticleStatistics`类,并实现了以下几个方法:
- `__init__(self)`:初始化`word_count`字典和`total_word_count`计数器,用于记录各个单词出现的次数和总共出现的单词数量。
- `process_text(self, text)`:接受一个字符串类型的文章,对其中的单词进行统计,将各个单词出现的次数和总共出现的单词数量记录在`word_count`字典和`total_word_count`计数器中。
- `get_word_count(self, word)`:接受一个单词作为参数,返回该单词在文章中出现的次数。
- `get_word_frequency(self, word)`:接受一个单词作为参数,返回该单词在文章中出现的频率(即出现次数除以总单词数)。
- `get_top_n_words(self, n)`:接受一个整数`n`作为参数,返回出现频率最高的前`n`个单词及其出现次数。
可以使用以下方式来使用该类:
```python
# 创建ArticleStatistics对象
article_stats = ArticleStatistics()
# 处理文章,进行单词统计
article_stats.process_text("This is a test article to demonstrate the ArticleStatistics class.")
# 获取单词的出现次数和频率
print(article_stats.get_word_count("article"))
print(article_stats.get_word_frequency("article"))
# 获取出现频率最高的前10个单词及其出现次数
top_words = article_stats.get_top_n_words(10)
print(top_words)
```
上述代码中,我们首先创建了一个`ArticleStatistics`对象`article_stats`,然后使用`process_text()`方法对文章进行处理,将其中的单词统计出来。接着使用`get_word_count()`和`get_word_frequency()`方法获取单词的出现次数和频率。最后,使用`get_top_n_words()`方法获取出现频率最高的前10个单词及其出现次数。
阅读全文
相关推荐

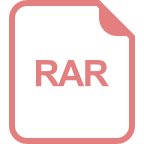















