python中switch case头文件
时间: 2023-11-22 14:50:55 浏览: 47
很抱歉,Python中并没有switch case头文件。事实上,Python中没有switch case语句,因为Python的设计者认为这种语句容易引起错误,并且可以用其他方式来实现相同的功能。如果你需要实现类似于switch case的功能,可以使用if-elif-else语句或者字典来实现。以下是两种实现方式的示例:
1.使用if-elif-else语句实现类似于switch case的功能:
```python
def switch_case(argument):
switcher = {
0: "zero",
1: "one",
2: "two",
}
return switcher.get(argument, "nothing")
print(switch_case(1)) # 输出:one
```
2.使用字典实现类似于switch case的功能:
```python
def switch_case(argument):
switcher = {
0: "zero",
1: "one",
2: "two",
}
return switcher.get(argument, "nothing")
print(switch_case(1)) # 输出:one
```
相关问题
python中sizeof的头文件
在Python中,没有类似C语言中的sizeof函数。Python中的变量类型和对象大小由解释器自行管理,开发者不需要手动管理内存。因此,在Python中并不存在sizeof头文件。如果你需要获取一个对象的大小,可以使用sys模块中的getsizeof函数来获取。该函数可以返回一个对象占用的内存空间大小,包括对象本身所占用的空间以及对象引用所占用的空间。以下是一个使用示例:
```
import sys
a = [1, 2, 3, 4, 5]
print(sys.getsizeof(a))
```
输出结果为:`120`。
python中读取dicom头文件
在Python中读取DICOM头文件可以使用`pydicom`包。首先需要安装`pydicom`包,可以通过以下命令进行安装:
```
pip install pydicom
```
然后可以使用以下代码读取DICOM头文件:
```python
import pydicom
# 读取DICOM文件
ds = pydicom.dcmread('path/to/dicom/file.dcm')
# 打印DICOM头信息
print(ds)
```
`pydicom`包会将DICOM文件解析为一个`Dataset`对象,该对象包含了所有的DICOM头信息。你可以使用`print(ds)`命令输出所有的DICOM头信息,也可以通过`ds[keyword]`来获取指定的头信息。例如,你可以通过以下代码获取DICOM文件的像素尺寸信息:
```python
# 获取像素尺寸信息
pixel_size = (ds.PixelSpacing[0], ds.PixelSpacing[1], ds.SliceThickness)
print('Pixel size:', pixel_size)
```
这里假设DICOM文件中包含了像素尺寸信息,如果没有该信息则会抛出异常。你可以根据DICOM文件的具体内容来确定需要获取哪些头信息。
相关推荐
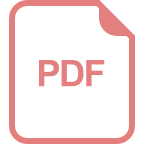
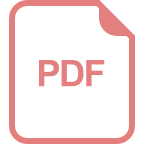












