vue如何在A页面点击按钮后跳转到B页面,同时在B页面打开弹窗
时间: 2024-11-12 09:34:32 浏览: 14
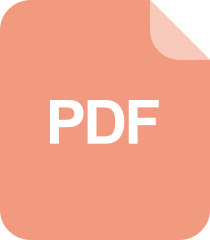
vue 弹窗时 监听手机返回键关闭弹窗功能(页面不跳转)
在Vue中,实现从A页面点击按钮跳转到B页面并打开弹窗,通常涉及两个部分:路由管理和组件通信。
1. **设置路由**:
首先,你需要安装`vue-router`插件,并在项目中配置路由。假设B页面是一个名为`B.vue`的组件,你可以这样做:
```javascript
// router.js 或者 main.js (取决于你的配置)
import Vue from 'vue'
import Router from 'vue-router'
Vue.use(Router)
const routes = [
{ path: '/a', component: AComponent },
{ path: '/b', component: BComponent }
]
export default new Router({
routes
})
```
在A组件里,可以添加一个按钮点击事件,通过导航守卫(`beforeRouteLeave`)来触发跳转:
```html
<template>
<button @click="goToBPage">跳转到B页面</button>
</template>
<script>
export default {
methods: {
goToBPage() {
this.$router.push('/b');
}
},
beforeRouteLeave(to, from, next) {
// 如果需要在离开A页面前执行额外操作,比如关闭弹窗,就在这里添加
next();
}
}
</script>
```
2. **组件间通信**:
在B页面,如果需要显示一个弹窗,你可以在B组件内部使用自定义事件(例如`v-on:`修饰符监听`@openModal`),然后在A页面触发这个事件。例如:
```html (在B.vue)
<!-- 弹窗按钮或其他触发点 -->
<button @click="$emit('openModal')">打开弹窗</button>
...
<template v-slot:default>
<!-- 弹窗内容 -->
</template>
```
```js (在B.vue)
export default {
methods: {
openModal() {
this.$emit('openModal'); // 触发自定义事件
}
}
}
```
在A页面接收并处理这个事件:
```html (在A.vue)
<template>
<div>
<router-link to="/b" @click.prevent="handleRouteAndOpenModal"></router-link>
</div>
</template>
<script>
export default {
methods: {
handleRouteAndOpenModal() {
this.goToBPage();
this.$parent.$on('openModal', () => { /* 在这里处理打开弹窗 */ });
}
},
beforeDestroy() {
this.$parent.$off('openModal'); // 跳转后移除监听
}
}
</script>
```
以上就是基本流程,实际应用中可能需要根据你的项目结构和个人喜好调整细节。如果你有任何关于这方面的疑问,欢迎提问。
阅读全文
相关推荐
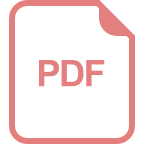
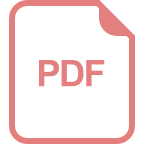

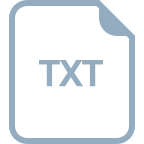
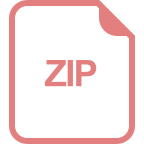
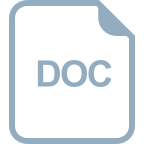










