图书管理系统C语言代码
时间: 2023-08-18 11:03:09 浏览: 128
以下是一个简单的图书管理系统的C语言代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_BOOKS 100
struct Book {
char title[100];
char author[100];
char publisher[100];
char ISBN[13];
};
struct BookLibrary {
struct Book books[MAX_BOOKS];
int count;
};
void addBook(struct BookLibrary* library) {
if (library->count >= MAX_BOOKS) {
printf("图书库已满,无法添加新的书籍。\n");
return;
}
struct Book newBook;
printf("请输入书名:");
fgets(newBook.title, 100, stdin);
newBook.title[strcspn(newBook.title, "\n")] = '\0';
printf("请输入作者:");
fgets(newBook.author, 100, stdin);
newBook.author[strcspn(newBook.author, "\n")] = '\0';
printf("请输入出版社:");
fgets(newBook.publisher, 100, stdin);
newBook.publisher[strcspn(newBook.publisher, "\n")] = '\0';
printf("请输入ISBN:");
fgets(newBook.ISBN, 13, stdin);
newBook.ISBN[strcspn(newBook.ISBN, "\n")] = '\0';
library->books[library->count] = newBook;
library->count++;
printf("书籍添加成功!\n");
}
void displayBooks(struct BookLibrary* library) {
if (library->count == 0) {
printf("图书库中没有任何书籍。\n");
return;
}
printf("图书库中的所有书籍:\n");
for (int i = 0; i < library->count; i++) {
printf("书名:%s\n", library->books[i].title);
printf("作者:%s\n", library->books[i].author);
printf("出版社:%s\n", library->books[i].publisher);
printf("ISBN:%s\n", library->books[i].ISBN);
printf("\n");
}
}
int main() {
struct BookLibrary library;
library.count = 0;
int choice;
while (1) {
printf("欢迎使用图书管理系统\n");
printf("1. 添加书籍\n");
printf("2. 显示所有书籍\n");
printf("3. 退出\n");
printf("请输入相应的操作编号:");
scanf("%d", &choice);
getchar(); // 清除输入缓冲区中的换行符
switch (choice) {
case 1:
addBook(&library);
break;
case 2:
displayBooks(&library);
break;
case 3:
printf("感谢使用图书管理系统,再见!\n");
exit(0);
default:
printf("无效的操作编号,请重新输入。\n");
}
}
return 0;
}
```
这段代码实现了一个简单的图书管理系统,用户可以选择添加书籍和显示所有书籍的功能。您可以根据需求自行扩展和修改代码。
希望对您有所帮助!
相关推荐
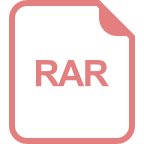
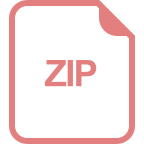











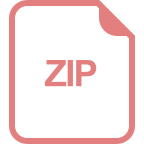
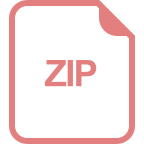