从信源编码技术:哈夫曼编码、菲诺编码、香农编码技术中选择一种,用代码实现其编码过程。
时间: 2024-10-24 19:09:08 浏览: 28
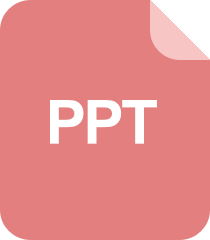
xi p(xi) 缩减信源 码字 li-信息论课件
让我们以哈夫曼编码为例,这是一种自适应的前缀编码,常用于数据压缩。它的基本思想是给频率较高的字符分配较短的编码,反之则较长,以此达到最优的数据压缩比。
以下是使用Python实现的简单哈夫曼编码过程:
首先,我们需要构建一个字典,存储每个字符及其对应的频率:
```python
from collections import Counter
# 假设我们有以下字符及频率
symbols = ['A', 'B', 'C', 'D', 'E']
freqs = {'A': 10, 'B': 20, 'C': 30, 'D': 40, 'E': 50}
```
然后,我们将频率构建成一个优先队列(堆),并开始构建哈夫曼树:
```python
import heapq
def build_huffman_tree(freqs):
heap = [[weight, [char, ""]] for char, weight in freqs.items()]
heapq.heapify(heap)
while len(heap) > 1:
left = heapq.heappop(heap)
right = heapq.heappop(heap)
combined_freq = left[0] + right[0]
new_node = [combined_freq, []]
new_node[1].append(left[1])
new_node[1].append(right[1])
heapq.heappush(heap, new_node)
return heap[0][1]
huffman_tree = build_huffman_tree(freqs)
```
最后,根据哈夫曼树为每个字符生成编码:
```python
def huffman_encode(symbols, tree):
code_dict = {symbol: prefix for symbol, prefix in zip(symbols, tree)}
encoded_string = "".join(code_dict[char] for char in s)
return encoded_string
encoded_text = huffman_encode(symbols, huffman_tree)
```
现在`encoded_text`就是按照哈夫曼编码压缩后的文本了。
阅读全文
相关推荐
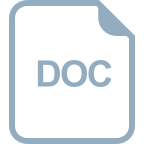
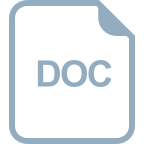
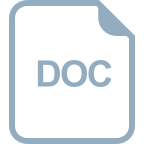
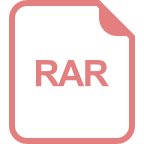
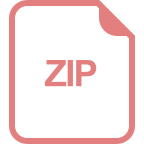
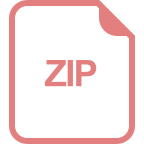
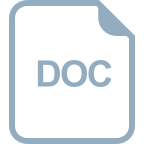
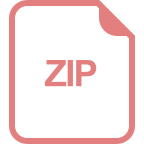
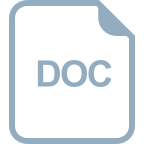
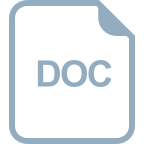
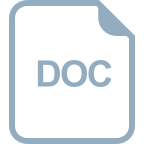
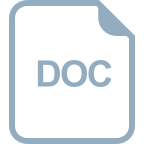
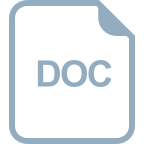
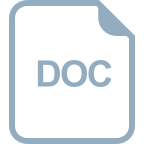
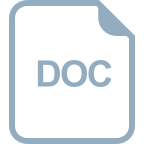
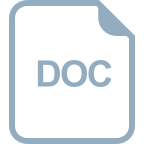
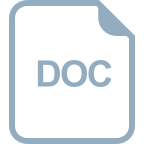
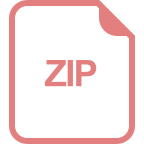