首页如何用C语言简单编写程序来统计字符串中各字符的出现次数?
如何用C语言简单编写程序来统计字符串中各字符的出现次数?
时间: 2024-11-23 12:40:40 浏览: 29
在C语言中,你可以使用数组来存储每个字符及其出现次数。下面是一个简单的示例程序,它会统计给定字符串中每个字符的出现次数:
```c
#include <stdio.h>
#include <string.h>
#define MAX_CHAR 128 // Assuming ASCII characters
// 定义字符计数数组
int char_count[MAX_CHAR] = {0};
void count_chars(char *str) {
while (*str != '\0') { // 遍历字符串直到遇到结束符
if (*str >= 'a' && *str <= 'z') { // 只考虑小写字母(ASCII码)
++char_count[*str]; // 对字母计数++
} else if (*str >= 'A' && *str <= 'Z') { // 同理,考虑大写字母
++char_count[*str - 'A' + 'a']; // 转换大小写并计数
}
str++;
}
}
int main() {
char input[100];
printf("Enter a string: ");
fgets(input, sizeof(input), stdin); // 从用户输入读取字符串
input[strcspn(input, "\n")] = '\0'; // 清除fgets留下的换行符
count_chars(input);
for (int i = 0; i < MAX_CHAR; i++) {
if (char_count[i] > 0) {
printf("'%c': %d\n", i, char_count[i]);
}
}
return 0;
}
```
这个程序首先定义了一个字符计数数组`char_count`,然后通过`count_chars`函数遍历输入的字符串,更新字符计数。最后,在`main`函数里,我们打印出每个非空计数的字符及其出现次数。
阅读全文
CSDN会员
开通CSDN年卡参与万元壕礼抽奖
最新推荐
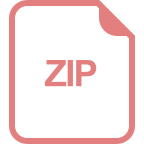
RStudio中集成Connections包以优化数据库连接管理
资源摘要信息:"connections:https"
### 标题解释
标题 "connections:https" 直接指向了数据库连接领域中的一个重要概念,即通过HTTP协议(HTTPS为安全版本)来建立与数据库的连接。在IT行业,特别是数据科学与分析、软件开发等领域,建立安全的数据库连接是日常工作的关键环节。此外,标题可能暗示了一个特定的R语言包或软件包,用于通过HTTP/HTTPS协议实现数据库连接。
### 描述分析
描述中提到的 "connections" 是一个软件包,其主要目标是与R语言的DBI(数据库接口)兼容,并集成到RStudio IDE中。它使得R语言能够连接到数据库,尽管它不直接与RStudio的Connections窗格集成。这表明connections软件包是一个辅助工具,它简化了数据库连接的过程,但并没有改变RStudio的用户界面。
描述还提到connections包能够读取配置,并创建与RStudio的集成。这意味着用户可以在RStudio环境下更加便捷地管理数据库连接。此外,该包提供了将数据库连接和表对象固定为pins的功能,这有助于用户在不同的R会话中持续使用这些资源。
### 功能介绍
connections包中两个主要的功能是 `connection_open()` 和可能被省略的 `c`。`connection_open()` 函数用于打开数据库连接。它提供了一个替代于 `dbConnect()` 函数的方法,但使用完全相同的参数,增加了自动打开RStudio中的Connections窗格的功能。这样的设计使得用户在使用R语言连接数据库时能有更直观和便捷的操作体验。
### 安装说明
描述中还提供了安装connections包的命令。用户需要先安装remotes包,然后通过remotes包的`install_github()`函数安装connections包。由于connections包不在CRAN(综合R档案网络)上,所以需要使用GitHub仓库来安装,这也意味着用户将能够访问到该软件包的最新开发版本。
### 标签解读
标签 "r rstudio pins database-connection connection-pane R" 包含了多个关键词:
- "r" 指代R语言,一种广泛用于统计分析和图形表示的编程语言。
- "rstudio" 指代RStudio,一个流行的R语言开发环境。
- "pins" 指代R包pins,它可能与connections包一同使用,用于固定数据库连接和表对象。
- "database-connection" 指代数据库连接,即软件包要解决的核心问题。
- "connection-pane" 指代RStudio IDE中的Connections窗格,connections包旨在与之集成。
- "R" 代表R语言社区或R语言本身。
### 压缩包文件名称列表分析
文件名称列表 "connections-master" 暗示了一个可能的GitHub仓库名称或文件夹名称。通常 "master" 分支代表了软件包或项目的稳定版或最新版,是大多数用户应该下载和使用的版本。
### 总结
综上所述,connections包是一个专为R语言和RStudio IDE设计的软件包,旨在简化数据库连接过程并提供与Connections窗格的集成。它允许用户以一种更为方便的方式打开和管理数据库连接,而不直接提供与Connections窗格的集成。connections包通过读取配置文件和固定连接对象,增强了用户体验。安装connections包需通过remotes包从GitHub获取最新开发版本。标签信息显示了connections包与R语言、RStudio、数据库连接以及R社区的紧密联系。

管理建模和仿真的文件
管理Boualem Benatallah引用此版本:布阿利姆·贝纳塔拉。管理建模和仿真。约瑟夫-傅立叶大学-格勒诺布尔第一大学,1996年。法语。NNT:电话:00345357HAL ID:电话:00345357https://theses.hal.science/tel-003453572008年12月9日提交HAL是一个多学科的开放存取档案馆,用于存放和传播科学研究论文,无论它们是否被公开。论文可以来自法国或国外的教学和研究机构,也可以来自公共或私人研究中心。L’archive ouverte pluridisciplinaire
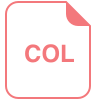
Keil uVision5全面精通指南
# 摘要
Keil uVision5是一个集成开发环境,广泛应用于嵌入式系统的开发。本文从基础入门开始,详细介绍了Keil uVision5的项目管理、配置、编程实践、高级功能及技巧,并通过项目案例分析,深入阐述了其在实际开发中的应用。本篇论文特别注重于项目创建、目标配置、调试环境搭建、性能优化、脚本编程与自动化、高级调试技术,以及通过嵌入式操作系统集成和驱动开发流程的案例分析,展示了Keil uVision5的实用性和高效性。文章还展望了Keil uVision5的未来发展趋势,并提供了社区资源和学习渠道,为读者提供了一个全面掌握Keil uVision5的平台。
# 关键字
Keil u

flink提交给yarn19个全量同步MYsqlCDC的作业,flink的配置参数怎样设置
在Flink中,如果你需要将19个MySQL CDC(Change Data Capture)的全量同步作业提交到YARN上,你需要确保Flink集群和YARN进行了正确的集成,并配置了适当的参数。以下是可能涉及到的一些关键配置:
1. **并行度(Parallelism)**:每个作业的并行度应该设置得足够高,以便充分利用YARN提供的资源。例如,如果你有19个任务,你可以设置总并行度为19或者是一个更大的数,取决于集群规模。
```yaml
parallelism = 19 或者 根据实际资源调整
```
2. **YARN资源配置**:Flink通过`yarn.a
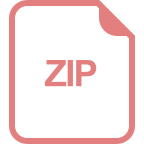
PHP博客旅游的探索之旅
资源摘要信息:"博客旅游"
博客旅游是一个以博客形式分享旅行经验和旅游信息的平台。随着互联网技术的发展和普及,博客作为一种个人在线日志的形式,已经成为人们分享生活点滴、专业知识、旅行体验等的重要途径。博客旅游正是结合了博客的个性化分享特点和旅游的探索性,让旅行爱好者可以记录自己的旅游足迹、分享旅游心得、提供目的地推荐和旅游攻略等。
在博客旅游中,旅行者可以是内容的创造者也可以是内容的消费者。作为创造者,旅行者可以通过博客记录下自己的旅行故事、拍摄的照片和视频、体验和评价各种旅游资源,如酒店、餐馆、景点等,还可以分享旅游小贴士、旅行日程规划等实用信息。作为消费者,其他潜在的旅行者可以通过阅读这些博客内容获得灵感、获取旅行建议,为自己的旅行做准备。
在技术层面,博客平台的构建往往涉及到多种编程语言和技术栈,例如本文件中提到的“PHP”。PHP是一种广泛使用的开源服务器端脚本语言,特别适合于网页开发,并可以嵌入到HTML中使用。使用PHP开发的博客旅游平台可以具有动态内容、用户交互和数据库管理等强大的功能。例如,通过PHP可以实现用户注册登录、博客内容的发布与管理、评论互动、图片和视频上传、博客文章的分类与搜索等功能。
开发一个功能完整的博客旅游平台,可能需要使用到以下几种PHP相关的技术和框架:
1. HTML/CSS/JavaScript:前端页面设计和用户交互的基础技术。
2. 数据库管理:如MySQL,用于存储用户信息、博客文章、评论等数据。
3. MVC框架:如Laravel或CodeIgniter,提供了一种组织代码和应用逻辑的结构化方式。
4. 服务器技术:如Apache或Nginx,作为PHP的运行环境。
5. 安全性考虑:需要实现数据加密、输入验证、防止跨站脚本攻击(XSS)等安全措施。
当创建博客旅游平台时,还需要考虑网站的可扩展性、用户体验、移动端适配、搜索引擎优化(SEO)等多方面因素。一个优质的博客旅游平台,不仅能够提供丰富的内容,还应该注重用户体验,包括页面加载速度、界面设计、内容的易于导航等。
此外,博客旅游平台还可以通过整合社交媒体功能,允许用户通过社交媒体账号登录、分享博客内容到社交网络,从而提升平台的互动性和可见度。
综上所述,博客旅游作为一个结合了旅行分享和在线日志的平台,对于旅行者来说,不仅是一个记录和分享旅行体验的地方,也是一个获取旅行信息、学习旅游知识的重要资源。而对于开发者来说,构建这样一个平台需要运用到多种技术和考虑多个技术细节,确保平台的功能性和用户体验。

"互动学习:行动中的多样性与论文攻读经历"
多样性她- 事实上SCI NCES你的时间表ECOLEDO C Tora SC和NCESPOUR l’Ingén学习互动,互动学习以行动为中心的强化学习学会互动,互动学习,以行动为中心的强化学习计算机科学博士论文于2021年9月28日在Villeneuve d'Asq公开支持马修·瑟林评审团主席法布里斯·勒菲弗尔阿维尼翁大学教授论文指导奥利维尔·皮耶昆谷歌研究教授:智囊团论文联合主任菲利普·普雷教授,大学。里尔/CRISTAL/因里亚报告员奥利维耶·西格德索邦大学报告员卢多维奇·德诺耶教授,Facebook /索邦大学审查员越南圣迈IMT Atlantic高级讲师邀请弗洛里安·斯特鲁布博士,Deepmind对于那些及时看到自己错误的人...3谢谢你首先,我要感谢我的两位博士生导师Olivier和Philippe。奥利维尔,"站在巨人的肩膀上"这句话对你来说完全有意义了。从科学上讲,你知道在这篇论文的(许多)错误中,你是我可以依
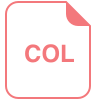
【单片机编程实战】:掌握流水灯与音乐盒同步控制的高级技巧
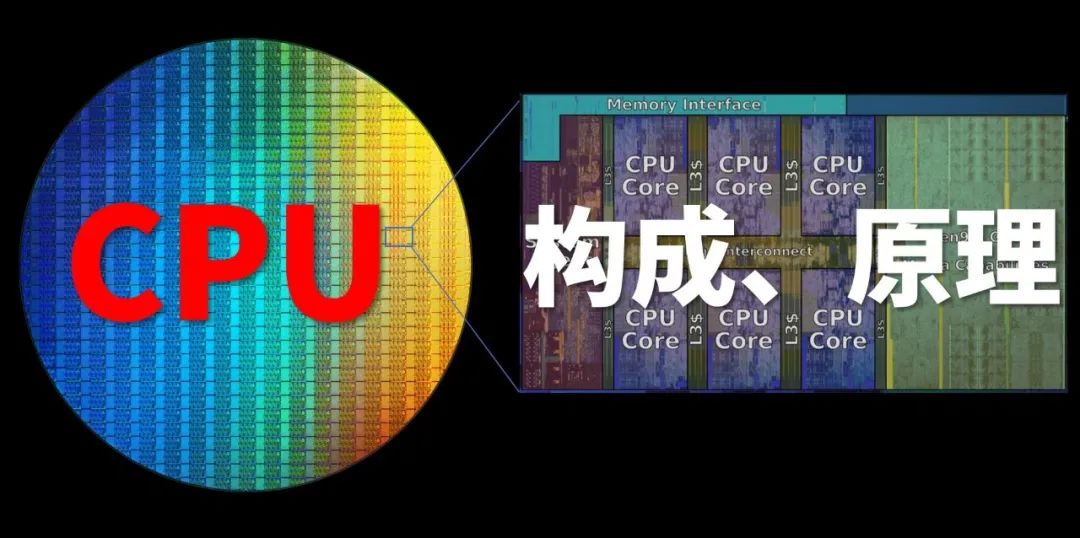
# 摘要
单片机作为电子技术领域的基础组件,广泛应用于各类控制项目。本文从基础开始,详细探讨了单片机在流水灯和音乐盒项目中的应用原理,并分析了如何实现这两个功能的同步控制。通过对硬件和软件层面的深入剖析,本文提供了一系列实践操作和高级技巧,旨在提升流水灯与音乐盒同步控制的性能和效率。通过本研究,读者将能够更好地理解和掌握单片机在复杂控制系统中的应用,进而创新开发更多

java 号码后四位用‘xxxx’脱敏
在Java中,对电话号码后四位进行脱敏通常是为了保护用户隐私。你可以通过字符串截取和替换的方式来实现这个功能。下面是一个简单的示例:
```java
public class Main {
public static void main(String[] args) {
String phoneNumber = "1234567890"; // 假设原始手机号
int startCutOff = phoneNumber.length() - 4; // 要开始切割的位置是后四位的起始位置
String maskedNumber = ph
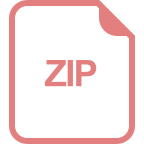
Arachne:实现UDP RIPv2协议的Java路由库
资源摘要信息:"arachne:基于Java的路由库"
知识点详细说明:
1. 知识点一:基于Java的路由库
- Arachne是一个基于Java开发的路由库,它允许开发者在Java环境中实现网络路由功能。
- Java在企业级应用中广泛使用,具有跨平台特性,因此基于Java的路由库能够适应多样的操作系统和硬件环境。
- 该路由库的出现,为Java开发者提供了一种新的网络编程选择,有助于在Java应用中实现复杂的路由逻辑。
2. 知识点二:简单Linux虚拟机上运行
- Arachne能够在资源受限的简单Linux虚拟机上运行,这意味着它对系统资源的要求不高,可以适用于计算能力有限的设备。
- 能够在虚拟机上运行的特性,使得Arachne可以轻松集成到云平台和虚拟化环境中,从而提供网络服务。
3. 知识点三:UDP协议与RIPv2路由协议
- Arachne实现了基于UDP协议的RIPv2(Routing Information Protocol version 2)路由协议。
- RIPv2是一种距离向量路由协议,用于在网络中传播路由信息。它规定了如何交换路由表,并允许路由器了解整个网络的拓扑结构。
- UDP协议具有传输速度快的特点,适用于RIP这种对实时性要求较高的网络协议。Arachne利用UDP协议实现RIPv2,有助于降低路由发现和更新的延迟。
- RIPv2较RIPv1增加了子网掩码和下一跳地址的支持,使其在现代网络中的适用性更强。
4. 知识点四:项目构建与模块组成
- Arachne项目由两个子项目构成,分别是arachne.core和arachne.test。
- arachne.core子项目是核心模块,负责实现路由库的主要功能;arachne.test是测试模块,用于对核心模块的功能进行验证。
- 使用Maven进行项目的构建,通过执行mvn clean package命令来生成相应的构件。
5. 知识点五:虚拟机环境配置
- Arachne在Oracle Virtual Box上的Ubuntu虚拟机环境中进行了测试。
- 虚拟机的配置使用了Vagrant和Ansible的组合,这种自动化配置方法可以简化环境搭建过程。
- 在Windows主机上,需要安装Oracle Virtual Box和Vagrant这两个软件,以支持虚拟机的创建和管理。
- 主机至少需要16 GB的RAM,以确保虚拟机能够得到足够的资源,从而提供最佳性能和稳定运行。
6. 知识点六:Vagrant Box的使用
- 使用Vagrant时需要添加Vagrant Box,这是一个预先配置好的虚拟机镜像文件,代表了特定的操作系统版本,例如ubuntu/trusty64。
- 通过添加Vagrant Box,用户可以快速地在本地环境中部署一个标准化的操作系统环境,这对于开发和测试是十分便利的。
7. 知识点七:Java技术在IT行业中的应用
- Java作为主流的编程语言之一,广泛应用于企业级应用开发,包括网络编程。
- Java的跨平台特性使得基于Java开发的软件具有很好的可移植性,能够在不同的操作系统上运行,无需修改代码。
- Java也具有丰富的网络编程接口,如Java NIO(New Input/Output),它提供了基于缓冲区的、面向块的I/O操作,适合于需要处理大量网络连接的应用程序。
8. 知识点八:网络协议与路由技术
- 理解各种网络协议是网络工程师和开发人员的基本技能之一,RIPv2是其中一种重要协议。
- 路由技术在网络架构设计中占有重要地位,它决定了数据包在网络中的传输路径。
- Arachne库的使用可以加深开发者对路由协议实现和网络架构设计的理解,帮助构建更加稳定和高效的网络系统。
通过上述知识点的介绍,我们可以看出Arachne作为一个基于Java的路由库,在技术实现、项目构建、环境配置以及网络技术等多个方面有着其独特之处,对Java开发者来说,这是一项值得学习和使用的技术资源。

关系数据表示学习
关系数据卢多维奇·多斯桑托斯引用此版本:卢多维奇·多斯桑托斯。关系数据的表示学习机器学习[cs.LG]。皮埃尔和玛丽·居里大学-巴黎第六大学,2017年。英语。NNT:2017PA066480。电话:01803188HAL ID:电话:01803188https://theses.hal.science/tel-01803188提交日期:2018年HAL是一个多学科的开放存取档案馆,用于存放和传播科学研究论文,无论它们是否被公开。论文可以来自法国或国外的教学和研究机构,也可以来自公共或私人研究中心。L’archive ouverte pluridisciplinaireUNIVERSITY PIERRE和 MARIE CURIE计算机科学、电信和电子学博士学院(巴黎)巴黎6号计算机科学实验室D八角形T HESIS关系数据表示学习作者:Ludovic DOS SAntos主管:Patrick GALLINARI联合主管:本杰明·P·伊沃瓦斯基为满足计算机科学博士学位的要求而提交的论文评审团成员:先生蒂埃里·A·退休记者先生尤尼斯·B·恩