opency运用python实现HSL二维直方图
时间: 2024-09-02 21:03:16 浏览: 101
OpenCV是一个开源的计算机视觉库,主要用于实时图像处理和计算机视觉任务。如果你想用Python通过OpenCV来实现HSL(色相、饱和度、亮度)色彩空间的二维直方图,你可以按照以下步骤操作:
1. 导入所需模块:
```python
import cv2
import numpy as np
```
2. 读取图片并转换到HSL色彩空间:
```python
img = cv2.imread('image.jpg') # 替换为你要处理的图片路径
hsl_img = cv2.cvtColor(img, cv2.COLOR_BGR2HLS)
```
3. 提取HSL三通道数据:
```python
h_channel = hsl_img[:, :, 0] # 色相
s_channel = hsl_img[:, :, 1] # 饱和度
l_channel = hsl_img[:, :, 2] # 亮度
```
4. 计算每个通道的直方图:
```python
hist_h, _ = np.histogram(h_channel, bins=np.arange(0, 180, 1)) # 对色相分段
hist_s, _ = np.histogram(s_channel, bins=np.linspace(0, 255, 256)) # 对饱和度分段
hist_l, _ = np.histogram(l_channel, bins=np.linspace(0, 255, 256)) # 对亮度分段
```
5. 绘制和显示二维直方图:
```python
hist_2d = np.vstack((hist_h, hist_s, hist_l))
x, y = np.meshgrid(range(len(hist_h)), range(len(hist_s)))
plt.imshow(hist_2d.T, origin='lower', extent=[0, len(hist_h), 0, len(hist_s)], cmap='gray')
plt.xlabel('Hue (Degrees)')
plt.ylabel('Saturation')
plt.colorbar()
plt.show()
```
在这个例子中,`imshow`函数用于绘制直方图,`extent`参数确定了坐标轴的范围。
阅读全文
相关推荐
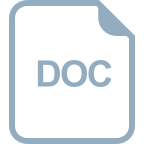
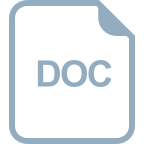
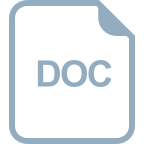
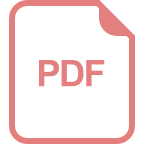
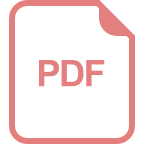
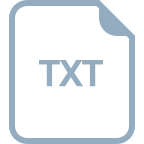
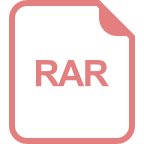
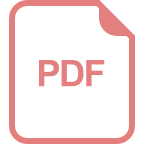
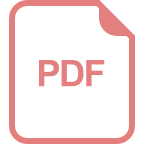
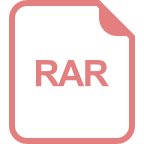
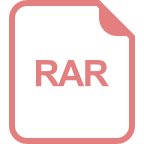
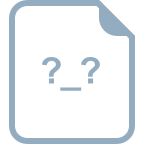
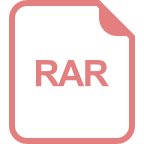
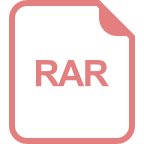
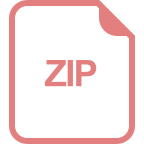
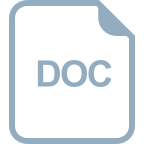
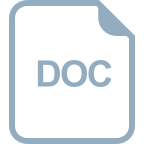

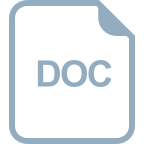