c#封装继承多态代码演示
时间: 2023-09-11 11:09:38 浏览: 67
封装、继承和多态是面向对象编程中的三个重要概念。下面是一个使用C#语言演示封装、继承和多态的代码示例:
封装示例:
```csharp
using System;
namespace EncapsulationApplication
{
class Employee
{
private string name;
private int age;
public string Name
{
get { return name; }
set { name = value; }
}
public int Age
{
get { return age; }
set { age = value; }
}
public void Display()
{
Console.WriteLine("Name: " + name);
Console.WriteLine("Age: " + age);
}
}
class Program
{
static void Main(string[] args)
{
Employee emp = new Employee();
emp.Name = "John";
emp.Age = 30;
emp.Display();
Console.ReadKey();
}
}
}
```
在这个示例中,Employee类封装了私有字段name和age,并通过公共属性Name和Age提供对它们的访问。Main方法中创建了一个Employee对象emp,并通过属性设置和Display方法展示了封装的效果。
继承示例:
```csharp
using System;
namespace InheritanceApplication
{
class Shape
{
protected int width;
protected int height;
public void setWidth(int w)
{
width = w;
}
public void setHeight(int h)
{
height = h;
}
}
class Rectangle : Shape
{
public int getArea()
{
return width * height;
}
}
class Program
{
static void Main(string[] args)
{
Rectangle rect = new Rectangle();
rect.setWidth(5);
rect.setHeight(7);
Console.WriteLine("Area: " + rect.getArea());
Console.ReadKey();
}
}
}
```
在这个示例中,Shape类作为基类,Rectangle类继承了Shape类。Rectangle类可以访问Shape类的protected字段和方法。Main方法中创建了一个Rectangle对象rect,并通过调用setWidth、setHeight和getArea方法展示了继承的效果。
多态示例:
```csharp
using System;
namespace PolymorphismApplication
{
class Shape
{
public virtual void Draw()
{
Console.WriteLine("Drawing a shape");
}
}
class Circle : Shape
{
public override void Draw()
{
Console.WriteLine("Drawing a circle");
}
}
class Rectangle : Shape
{
public override void Draw()
{
Console.WriteLine("Drawing a rectangle");
}
}
class Program
{
static void Main(string[] args)
{
Shape[] shapes = new Shape[3];
shapes[0] = new Shape();
shapes[1] = new Circle();
shapes[2] = new Rectangle();
foreach (Shape shape in shapes)
{
shape.Draw();
}
Console.ReadKey();
}
}
}
```
在这个示例中,Shape类定义了一个虚拟的Draw方法,Circle类和Rectangle类分别重写了这个方法。Main方法中创建了一个Shape类型的数组,并分别用Shape、Circle和Rectangle的实例填充数组。通过遍历数组并调用Draw方法,展示了多态的效果。
相关推荐
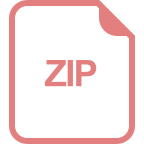
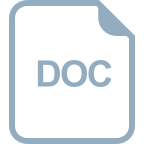
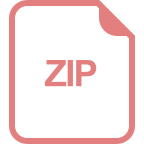














