java poi导出设置 字体:宋体, 标题 16号加粗,表头 10号加粗, 内容 10号
时间: 2024-04-14 10:25:54 浏览: 166
您可以使用 Apache POI 库来设置导出的字体样式。下面是一个示例代码,演示如何设置字体为宋体、标题为16号加粗、表头和内容为10号加粗:
```java
import org.apache.poi.ss.usermodel.*;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
import java.io.FileOutputStream;
import java.io.IOException;
public class ExcelExporter {
public static void main(String[] args) {
// 创建工作簿
Workbook workbook = new XSSFWorkbook();
// 创建字体样式
Font titleFont = workbook.createFont();
titleFont.setFontName("宋体");
titleFont.setFontHeightInPoints((short) 16);
titleFont.setBold(true);
Font headerFont = workbook.createFont();
headerFont.setFontName("宋体");
headerFont.setFontHeightInPoints((short) 10);
headerFont.setBold(true);
Font contentFont = workbook.createFont();
contentFont.setFontName("宋体");
contentFont.setFontHeightInPoints((short) 10);
// 创建标题样式
CellStyle titleStyle = workbook.createCellStyle();
titleStyle.setFont(titleFont);
// 创建表头样式
CellStyle headerStyle = workbook.createCellStyle();
headerStyle.setFont(headerFont);
// 创建内容样式
CellStyle contentStyle = workbook.createCellStyle();
contentStyle.setFont(contentFont);
// 创建工作表
Sheet sheet = workbook.createSheet("Sheet1");
// 设置标题
Row titleRow = sheet.createRow(0);
Cell titleCell = titleRow.createCell(0);
titleCell.setCellValue("标题");
titleCell.setCellStyle(titleStyle);
// 设置表头
Row headerRow = sheet.createRow(1);
Cell headerCell = headerRow.createCell(0);
headerCell.setCellValue("表头");
headerCell.setCellStyle(headerStyle);
// 设置内容
Row contentRow = sheet.createRow(2);
Cell contentCell = contentRow.createCell(0);
contentCell.setCellValue("内容");
contentCell.setCellStyle(contentStyle);
// 保存文件
try {
FileOutputStream fileOut = new FileOutputStream("export.xlsx");
workbook.write(fileOut);
fileOut.close();
workbook.close();
System.out.println("导出成功!");
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
请注意,代码中的导出文件名为 "export.xlsx",您可以根据自己的需求修改该名称。另外,此示例使用的是 XSSFWorkbook 类来创建 Excel 文件,如果您需要创建 .xls 格式的文件,可以使用 HSSFWorkbook 类来代替。
希望对您有帮助!如果您有任何其他问题,请随时提问。
阅读全文
相关推荐
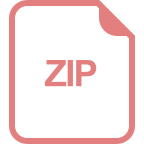
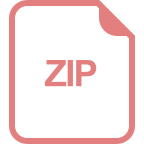
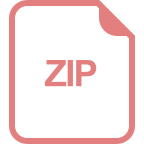














