application/json vue传值
时间: 2023-09-26 10:04:28 浏览: 183
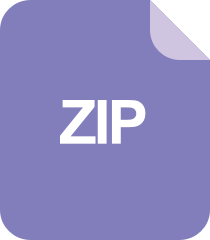
谷歌插件 vue和json美化
在 Vue 中传递 JSON 数据可以通过 props 属性或事件进行。以下是两种常用的方法:
1. 使用 props 属性传递 JSON 数据:
在父组件中,可以通过 props 将 JSON 数据传递给子组件。首先,在父组件中定义一个属性,并将 JSON 数据作为值传递给该属性。然后,在子组件中使用 props 来接收并使用这些数据。
父组件中的示例代码:
```
<template>
<div>
<child-component :jsonData="myJsonData"></child-component>
</div>
</template>
<script>
import ChildComponent from './ChildComponent.vue';
export default {
components: {
ChildComponent
},
data() {
return {
myJsonData: {
name: 'John',
age: 25
}
};
}
};
</script>
```
子组件的示例代码(ChildComponent.vue):
```
<template>
<div>
<p>Name: {{ jsonData.name }}</p>
<p>Age: {{ jsonData.age }}</p>
</div>
</template>
<script>
export default {
props: {
jsonData: {
type: Object,
required: true
}
}
};
</script>
```
2. 使用事件(event)传递 JSON 数据:
在父组件中,可以使用 $emit 方法触发一个自定义事件,并附带 JSON 数据作为参数。然后,在子组件中使用 $on 来监听该事件,并处理接收到的数据。
父组件中的示例代码:
```
<template>
<div>
<button @click="sendJsonData">Send JSON Data</button>
<child-component @json-data-received="handleJsonData"></child-component>
</div>
</template>
<script>
import ChildComponent from './ChildComponent.vue';
export default {
components: {
ChildComponent
},
methods: {
sendJsonData() {
const jsonData = {
name: 'John',
age: 25
};
this.$emit('json-data-received', jsonData);
}, handleJsonData(jsonData) {
console.log(jsonData);
}
}
};
</script>
```
子组件的示例代码(ChildComponent.vue):
```
<template>
<div></div>
</template>
<script>
export default {
mounted() {
this.$parent.$on('json-data-received', this.handleJsonData);
},
beforeUnmount() {
this.$parent.$off('json-data-received', this.handleJsonData);
},
methods: {
handleJsonData(jsonData) {
console.log(jsonData);
// 处理接收到的 JSON 数据
}
}
};
</script>
```
这两种方法可以根据你的需求选择适合的方式来传递和处理 JSON 数据。
阅读全文
相关推荐
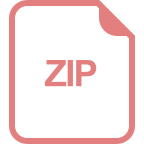















