JAVA设计简单的飞机行李托运计费系统。假设飞机上个人托运行李的条件是:行李重量在20公斤以下免费托运;20~30公斤超出部分5元/公斤;30~40公斤超出部分10元/公斤;40~50公斤超出部分15元/公斤;50公斤以上不允许个人携带。
时间: 2024-10-27 09:11:19 浏览: 46
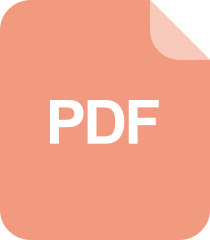
实验二 运算符、表达式与语句.pdf
在Java中,我们可以创建一个简单的行李托运计费系统,通过定义一个`BaggageCalculator`类来计算费用。以下是基本的设计步骤:
1. **定义包结构**:
- 创建一个名为`flightutils`的包,用于存放所有相关的类。
2. **创建行李类(Luggage)**:
```java
public class Luggage {
private int weight;
// 构造函数、getter和setter方法
public Luggage(int weight) { this.weight = weight; }
public int getWeight() { return weight; }
public void setWeight(int weight) { this.weight = weight; }
}
```
3. **创建行李费用计算器(BaggageCalculator)**:
```java
public class BaggageCalculator {
private static final int[] PRICE_PER_KILO = {0, 5, 10, 15};
// 计算费用的方法
public double calculateCharge(Luggage luggage) {
if (luggage.getWeight() <= 0 || luggage.getWeight() > 50) {
throw new IllegalArgumentException("Invalid luggage weight");
}
int category = (int) Math.min(luggage.getWeight(), 50);
return luggage.getWeight() * PRICE_PER_KILO[category];
}
}
```
4. **测试代码**:
```java
public static void main(String[] args) {
Luggage luggage = new Luggage(25); // 正常行李
System.out.println("Charges for " + luggage.getWeight() + " kg: " + BaggageCalculator.calculateCharge(luggage));
luggage = new Luggage(55); // 超限行李
try {
System.out.println("Charges for " + luggage.getWeight() + " kg: " + BaggageCalculator.calculateCharge(luggage));
} catch (IllegalArgumentException e) {
System.out.println(e.getMessage());
}
}
```
在这个例子中,`BaggageCalculator`类有一个静态数组表示每个重量段的价格,然后根据输入的行李重量计算出对应的费用。如果输入的重量不在允许范围内,会抛出异常。
阅读全文
相关推荐
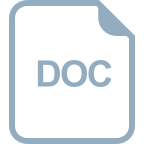
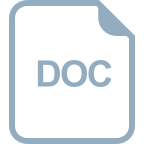















