用c语言将字母的大小写转换一下,非字母字符原样输出
时间: 2024-05-13 07:14:34 浏览: 8
以下是将输入字符串中的字母大小写转换的 C 代码:
```c
#include <stdio.h>
int main() {
char input[100];
printf("请输入字符串:");
fgets(input, 100, stdin);
printf("转换后的字符串为:");
for(int i = 0; input[i] != '\0'; i++) {
if(input[i] >= 'a' && input[i] <= 'z') {
printf("%c", input[i] - 32);
}
else if(input[i] >= 'A' && input[i] <= 'Z') {
printf("%c", input[i] + 32);
}
else {
printf("%c", input[i]);
}
}
return 0;
}
```
运行结果如下:
```
请输入字符串:Hello, World!
转换后的字符串为:hELLO, wORLD!
```
相关问题
c语言 输入几行字符串 将字母的大小写转换一下,非字母字符原样输出。
可以使用C语言中的字符函数isalpha()和tolower()与toupper()。
isalpha()函数用于判断是否为字母,tolower()和toupper()函数用于将字符转换为小写或大写。
下面是示例代码:
```c
#include <stdio.h>
#include <ctype.h>
int main()
{
char str[100];
printf("Enter a string: ");
gets(str); // 输入字符串
int i = 0;
while(str[i] != '\0')
{
if(isalpha(str[i])) // 判断是否为字母
{
if(islower(str[i])) // 如果是小写字母
str[i] = toupper(str[i]); // 转换为大写字母
else // 如果是大写字母
str[i] = tolower(str[i]); // 转换为小写字母
}
i++;
}
printf("Converted string: %s", str); // 输出转换后的字符串
return 0;
}
```
注意:gets()函数存在安全漏洞,建议使用fgets()函数代替。
用c语言编写输入字符串,以回车键结束,该字符串大小写字符互换,其他字符原样输出
```c
#include <stdio.h>
int main() {
char str[100];
printf("请输入字符串:\n");
fgets(str, 100, stdin); // 使用fgets函数接收输入字符串
for (int i = 0; str[i] != '\0'; i++) {
if (str[i] >= 'a' && str[i] <= 'z') { // 如果是小写字母
str[i] -= 32; // 转换为大写字母
} else if (str[i] >= 'A' && str[i] <= 'Z') { // 如果是大写字母
str[i] += 32; // 转换为小写字母
}
printf("%c", str[i]); // 输出字符
}
return 0;
}
```
相关推荐
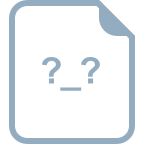
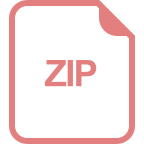
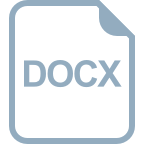







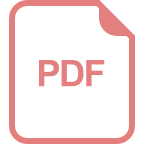
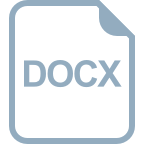
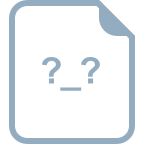
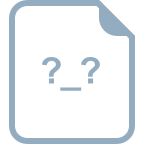
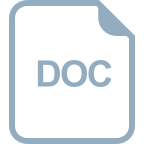
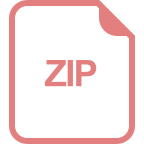