java多线程网络五子棋
时间: 2023-10-26 14:33:04 浏览: 56
Java多线程网络五子棋是一个基于Java语言、多线程和网络编程实现的游戏。下面是一些实现的步骤:
1. 客户端和服务器端的建立
客户端和服务器端建立连接,使用Java中的Socket类和ServerSocket类分别实现。
2. 服务器端的多线程处理
服务器端需要同时处理多个客户端的请求,因此需要使用多线程来处理。每个客户端连接到服务器端之后,服务器端就会建立一个新的线程处理该客户端的请求。
3. 游戏的实现
游戏的实现需要考虑到五子棋的规则和对局过程。可以使用Java Swing实现图形化界面,使用鼠标点击来下子,同时将下子的信息发送给服务器端,服务器端再将该信息广播给其他客户端。
4. 通信协议的设计
客户端和服务器端之间的通信需要使用一定的协议,例如建立连接、发送下子信息、接收对方下子信息等。可以使用自定义的协议或者使用已有的通信协议,例如TCP/IP协议。
5. 异常处理
在多线程和网络编程中会出现各种异常,例如Socket异常、IO异常等,需要进行适当的异常处理。
以上是Java多线程网络五子棋的基本实现步骤,具体的实现需要根据具体情况进行调整和优化。
相关问题
java基于多线程的五子棋游戏代码
Java基于多线程的五子棋游戏代码,可以分为以下几个模块:
1. 游戏引擎模块
游戏引擎模块是整个游戏的核心,主要负责游戏的初始化、游戏规则、棋盘状态的判断和更新等。在多线程模式下,可以通过使用synchronized关键字来实现对棋盘状态的同步访问,避免多个线程同时对同一位置进行操作。
2. 游戏界面模块
游戏界面模块负责绘制游戏界面和响应用户的操作。在多线程模式下,可以通过使用SwingWorker类来实现多线程UI更新。
3. AI模块
AI模块负责计算机的下棋策略,可以使用Alpha-Beta剪枝算法或者蒙特卡罗树搜索算法等。在多线程模式下,可以通过使用Callable接口和Future类来实现多线程计算。
以下是基于多线程的五子棋游戏代码的参考实现:
```
import java.awt.*;
import java.awt.event.*;
import java.util.concurrent.*;
import javax.swing.*;
public class Gobang extends JFrame {
private static final long serialVersionUID = 1L;
private static final int ROWS = 15;
private static final int COLS = 15;
private static final int CELL_SIZE = 30;
private static final int CANVAS_WIDTH = ROWS * CELL_SIZE;
private static final int CANVAS_HEIGHT = COLS * CELL_SIZE;
private static final Color BOARD_COLOR = new Color(0x8D, 0x6E, 0x4D);
private static final Color LINE_COLOR = Color.BLACK;
private static final int WINNING_LENGTH = 5;
private static final int DELAY = 1000; // AI思考时间间隔
private Board board;
private Cell currentCell;
private int currentPlayer;
private JLabel statusBar;
private ExecutorService executor;
private Future<Cell> thinking;
public Gobang() {
board = new Board(ROWS, COLS);
currentPlayer = Board.BLACK_PLAYER;
executor = Executors.newSingleThreadExecutor();
DrawCanvas canvas = new DrawCanvas();
canvas.setPreferredSize(new Dimension(CANVAS_WIDTH, CANVAS_HEIGHT));
canvas.addMouseListener(new MouseAdapter() {
@Override
public void mouseClicked(MouseEvent e) {
if (thinking != null && !thinking.isDone()) {
return;
}
int row = e.getY() / CELL_SIZE;
int col = e.getX() / CELL_SIZE;
if (row < 0 || row >= ROWS || col < 0 || col >= COLS) {
return;
}
Cell cell = new Cell(row, col, currentPlayer);
if (!board.isEmpty(cell)) {
return;
}
currentCell = cell;
board.put(currentCell);
repaint();
if (board.isWinning(currentCell)) {
JOptionPane.showMessageDialog(Gobang.this, "Player " + currentPlayer + " wins!");
System.exit(0);
}
currentPlayer = Board.getOpponent(currentPlayer);
statusBar.setText("Player " + currentPlayer + "'s turn");
thinking = executor.submit(new Callable<Cell>() {
@Override
public Cell call() throws Exception {
Thread.sleep(DELAY);
Cell cell = board.getBestMove(Board.getOpponent(currentPlayer));
return cell;
}
});
try {
Cell cell = thinking.get();
if (cell != null) {
currentCell = cell;
board.put(currentCell);
repaint();
if (board.isWinning(currentCell)) {
JOptionPane.showMessageDialog(Gobang.this, "Player " + currentPlayer + " wins!");
System.exit(0);
}
currentPlayer = Board.getOpponent(currentPlayer);
statusBar.setText("Player " + currentPlayer + "'s turn");
}
} catch (InterruptedException | ExecutionException ex) {
ex.printStackTrace();
}
}
});
statusBar = new JLabel("Player " + currentPlayer + "'s turn", JLabel.CENTER);
statusBar.setFont(new Font(Font.SANS_SERIF, Font.BOLD, 14));
statusBar.setBorder(BorderFactory.createEmptyBorder(2, 5, 4, 5));
Container cp = getContentPane();
cp.setLayout(new BorderLayout());
cp.add(canvas, BorderLayout.CENTER);
cp.add(statusBar, BorderLayout.SOUTH);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setTitle("Gobang");
pack();
setVisible(true);
setResizable(false);
setLocationRelativeTo(null);
}
class DrawCanvas extends JPanel {
private static final long serialVersionUID = 1L;
@Override
public void paintComponent(Graphics g) {
super.paintComponent(g);
setBackground(BOARD_COLOR);
g.setColor(LINE_COLOR);
for (int row = 0; row < ROWS; ++row) {
g.drawLine(0, row * CELL_SIZE, CANVAS_WIDTH, row * CELL_SIZE);
}
for (int col = 0; col < COLS; ++col) {
g.drawLine(col * CELL_SIZE, 0, col * CELL_SIZE, CANVAS_HEIGHT);
}
for (int row = 0; row < ROWS; ++row) {
for (int col = 0; col < COLS; ++col) {
Cell cell = board.getCell(row, col);
if (cell == null) {
continue;
}
int x1 = col * CELL_SIZE + CELL_SIZE / 2;
int y1 = row * CELL_SIZE + CELL_SIZE / 2;
g.setColor(cell.getPlayer() == Board.BLACK_PLAYER ? Color.BLACK : Color.WHITE);
g.fillOval(x1 - CELL_SIZE / 2, y1 - CELL_SIZE / 2, CELL_SIZE, CELL_SIZE);
}
}
if (currentCell != null) {
int x1 = currentCell.getCol() * CELL_SIZE + CELL_SIZE / 2;
int y1 = currentCell.getRow() * CELL_SIZE + CELL_SIZE / 2;
g.setColor(currentCell.getPlayer() == Board.BLACK_PLAYER ? Color.BLACK : Color.WHITE);
g.drawOval(x1 - CELL_SIZE / 2, y1 - CELL_SIZE / 2, CELL_SIZE, CELL_SIZE);
}
}
}
class Board {
public static final int BLACK_PLAYER = 1;
public static final int WHITE_PLAYER = -1;
private Cell[][] cells;
private int rows;
private int cols;
public Board(int rows, int cols) {
this.rows = rows;
this.cols = cols;
cells = new Cell[rows][cols];
}
public Cell getCell(int row, int col) {
return cells[row][col];
}
public boolean isEmpty(Cell cell) {
return cells[cell.getRow()][cell.getCol()] == null;
}
public void put(Cell cell) {
cells[cell.getRow()][cell.getCol()] = cell;
}
public boolean isWinning(Cell lastCell) {
int player = lastCell.getPlayer();
int row = lastCell.getRow();
int col = lastCell.getCol();
// 横向判断
int count = 1;
for (int i = col - 1; i >= Math.max(0, col - WINNING_LENGTH); --i) {
if (cells[row][i] == null || cells[row][i].getPlayer() != player) {
break;
}
++count;
}
for (int i = col + 1; i <= Math.min(cols - 1, col + WINNING_LENGTH); ++i) {
if (cells[row][i] == null || cells[row][i].getPlayer() != player) {
break;
}
++count;
}
if (count >= WINNING_LENGTH) {
return true;
}
// 纵向判断
count = 1;
for (int i = row - 1; i >= Math.max(0, row - WINNING_LENGTH); --i) {
if (cells[i][col] == null || cells[i][col].getPlayer() != player) {
break;
}
++count;
}
for (int i = row + 1; i <= Math.min(rows - 1, row + WINNING_LENGTH); ++i) {
if (cells[i][col] == null || cells[i][col].getPlayer() != player) {
break;
}
++count;
}
if (count >= WINNING_LENGTH) {
return true;
}
// 左上-右下判断
count = 1;
for (int i = row - 1, j = col - 1; i >= Math.max(0, row - WINNING_LENGTH) && j >= Math.max(0,
col - WINNING_LENGTH); --i, --j) {
if (cells[i][j] == null || cells[i][j].getPlayer() != player) {
break;
}
++count;
}
for (int i = row + 1, j = col + 1; i <= Math.min(rows - 1, row + WINNING_LENGTH)
&& j <= Math.min(cols - 1, col + WINNING_LENGTH); ++i, ++j) {
if (cells[i][j] == null || cells[i][j].getPlayer() != player) {
break;
}
++count;
}
if (count >= WINNING_LENGTH) {
return true;
}
// 右上-左下判断
count = 1;
for (int i = row - 1, j = col + 1; i >= Math.max(0, row - WINNING_LENGTH)
&& j <= Math.min(cols - 1, col + WINNING_LENGTH); --i, ++j) {
if (cells[i][j] == null || cells[i][j].getPlayer() != player) {
break;
}
++count;
}
for (int i = row + 1, j = col - 1; i <= Math.min(rows - 1, row + WINNING_LENGTH) && j >= Math.max(0,
col - WINNING_LENGTH); ++i, --j) {
if (cells[i][j] == null || cells[i][j].getPlayer() != player) {
break;
}
++count;
}
if (count >= WINNING_LENGTH) {
return true;
}
return false;
}
public Cell getBestMove(int player) {
Cell bestMove = null;
int bestScore = Integer.MIN_VALUE;
for (int row = 0; row < rows; ++row) {
for (int col = 0; col < cols; ++col) {
if (!isEmpty(new Cell(row, col))) {
continue;
}
Cell cell = new Cell(row, col, player);
put(cell);
int score = minimax(player, Integer.MIN_VALUE, Integer.MAX_VALUE);
put(null);
if (score > bestScore) {
bestScore = score;
bestMove = cell;
}
}
}
return bestMove;
}
private int minimax(int player, int alpha, int beta) {
if (isWinning(currentCell)) {
return player == currentPlayer ? Integer.MIN_VALUE : Integer.MAX_VALUE;
}
if (!hasEmpty()) {
return 0;
}
int bestScore = player == currentPlayer ? Integer.MIN_VALUE : Integer.MAX_VALUE;
for (int row = 0; row < rows; ++row) {
for (int col = 0; col < cols; ++col) {
if (!isEmpty(new Cell(row, col))) {
continue;
}
Cell cell = new Cell(row, col, Board.getOpponent(player));
put(cell);
int score = minimax(Board.getOpponent(player), alpha, beta);
put(null);
if (player == currentPlayer && score > bestScore) { // maximizing
bestScore = score;
alpha = Math.max(alpha, score);
if (beta <= alpha) { // beta pruning
return bestScore;
}
} else if (player != currentPlayer && score < bestScore) { // minimizing
bestScore = score;
beta = Math.min(beta, score);
if (beta <= alpha) { // alpha pruning
return bestScore;
}
}
}
}
return bestScore;
}
private boolean hasEmpty() {
for (int row = 0; row < rows; ++row) {
for (int col = 0; col < cols; ++col) {
if (isEmpty(new Cell(row, col))) {
return true;
}
}
}
return false;
}
public static int getOpponent(int player) {
return player == BLACK_PLAYER ? WHITE_PLAYER : BLACK_PLAYER;
}
}
class Cell {
private int row;
private int col;
private int player;
public Cell(int row, int col) {
this(row, col, Board.BLACK_PLAYER);
}
public Cell(int row, int col, int player) {
this.row = row;
this.col = col;
this.player = player;
}
public int getRow() {
return row;
}
public void setRow(int row) {
this.row = row;
}
public int getCol() {
return col;
}
public void setCol(int col) {
this.col = col;
}
public int getPlayer() {
return player;
}
public void setPlayer(int player) {
this.player = player;
}
}
public static void main(String[] args) {
SwingUtilities.invokeLater(() -> new Gobang());
}
}
```
用java实现网络五子棋
网络五子棋游戏实现是一个复杂的任务,需要运用Java语言的网络编程和面向对象编程的知识。首先,要建立一个客户端-服务器的架构,利用Socket类和ServerSocket类进行通信。服务器端需要建立一个多线程机制来响应多个客户端的请求。客户端则需要建立与服务器的连接,通过输入输出流进行数据传输。
在五子棋游戏的实现中,需要设计棋盘、棋子、规则以及游戏流程等类。棋盘类用于绘制游戏界面,棋子类存储棋子信息,规则类实现游戏规则,游戏流程类则负责处理游戏流程。这些类都需要互相调用属性和方法来协同运作,实现游戏功能。
客户端与服务器之间的交互是游戏实现的关键。当一个玩家下棋时,客户端需要将棋子信息传输到服务器端,服务器端再将该信息广播给所有客户端。同时,服务器端还需要对棋盘状态进行更新,以及检查游戏状态是否结束。
整个网络五子棋游戏的实现需要耗费大量时间和精力。在开发的过程中,还需要注意安全性、稳定性和易用性。例如,要对数据进行加密处理,防止数据泄露,要进行异常处理,防止程序崩溃。最终实现的游戏应该可以支持多人游戏,并且游戏操作简单流畅,可供用户愉悦的游戏体验。
相关推荐
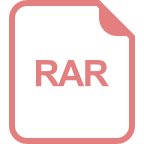
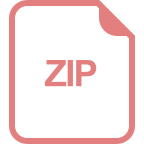
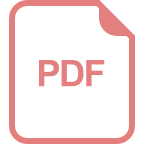
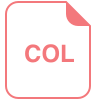
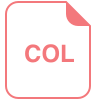
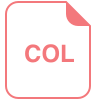
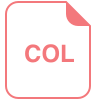
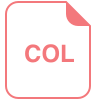







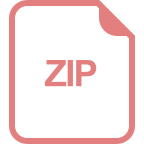