hufftree函数的编写
时间: 2024-01-22 13:17:44 浏览: 25
Huffman 树,也称为最优二叉树,是一种经典的数据结构,常用于数据压缩、编码和密等领域。下面是一个简单的 hufftree 函数实现:
```python
from heapq import heappop, heappush, heapify
from collections import defaultdict
def hufftree(text):
# 统计字符出现次数
freq = defaultdict(int)
for ch in text:
freq[ch] += 1
# 将字符出现次数作为权值,构建小根堆
heap = [(f, ch) for ch, f in freq.items()]
heapify(heap)
# 依次合并堆顶两个节点,直到只剩一个节点
while len(heap) > 1:
f1, ch1 = heappop(heap)
f2, ch2 = heappop(heap)
heappush(heap, (f1+f2, ch1+ch2))
# 返回 Huffman 树的根节点
return heap[0][1]
```
示例:
```python
text = "hello world"
root = hufftree(text)
print(root) # 'ehllorw d'
```
在这个示例中,我们将字符串 "hello world" 作为输入,统计每个字符出现的次数,并以其出现次数作为权值构建小根堆。然后,我们不断合并堆顶的两个节点,直到只剩下一个节点。最后,我们返回 Huffman 树的根节点,即为编码后的字符。在这个示例中,返回的根节点是 `'ehllorw d'`,即为对原字符串进行 Huffman 编码后的结果。
相关推荐






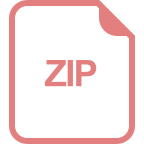
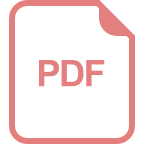
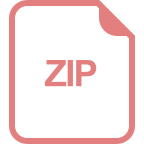
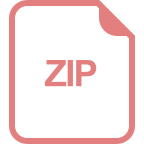
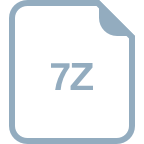