如何在Python中封装MongoDB的关键字操作?
时间: 2024-11-07 17:13:32 浏览: 11
在Python中,你可以通过Pymongo库来封装MongoDB的关键字操作,使其更易于管理和复用。以下是一个简单的例子:
首先,导入必要的库:
```python
from pymongo import MongoClient
from typing import List
```
然后,创建一个连接到MongoDB的函数,并封装查询、插入、更新和删除等操作:
```python
def connect_to_mongodb(uri: str) -> MongoClient:
client = MongoClient(uri)
return client
def get_database(db_name: str, client):
db = client[db_name]
return db
def query_data(collection_name: str, filter_dict: dict, client=None):
if not client:
client = connect_to_mongodb()
collection = get_database("your_database", client)[collection_name]
return collection.find(filter_dict)
def insert_document(collection_name: str, document: dict, client=None):
if not client:
client = connect_to_mongodb()
collection = get_database("your_database", client)[collection_name]
result = collection.insert_one(document)
return result.inserted_id
def update_document(collection_name: str, filter_dict: dict, update_dict: dict, upsert=False, client=None):
if not client:
client = connect_to_mongodb()
collection = get_database("your_database", client)[collection_name]
result = collection.update_one(filter_dict, {"$set": update_dict}, upsert=upsert)
return result.modified_count
def delete_document(collection_name: str, filter_dict: dict, client=None):
if not client:
client = connect_to_mongodb()
collection = get_database("your_database", client)[collection_name]
result = collection.delete_one(filter_dict)
return result.deleted_count
```
现在你可以通过`query_data()`, `insert_document()`, `update_document()`, 或者`delete_document()`这样的封装函数来进行关键字操作。
阅读全文
相关推荐
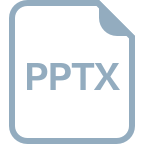
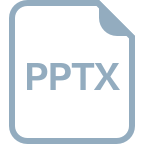
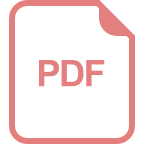
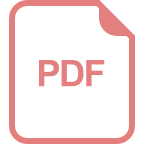
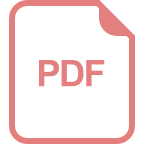
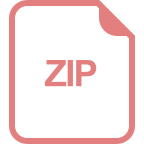
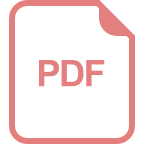
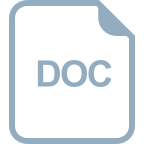
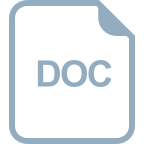
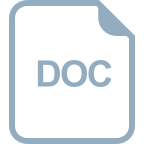
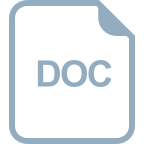
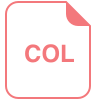
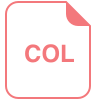
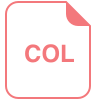
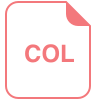
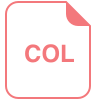
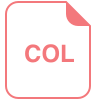