<androidx.recyclerview.widget.RecyclerView android:id="@+id/city_recycler" android:layout_width="match_parent" android:layout_height="match_parent" android:layout_below="@id/city_line" android:divider="@null"></androidx.recyclerview.widget.RecyclerView>
时间: 2024-03-15 13:42:50 浏览: 137
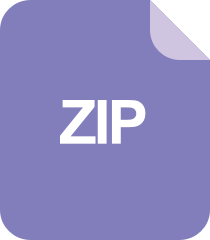
android-RecyclerView-master.zip_android_recyclerview
这是一个 Android 布局文件中的代码片段,其中定义了一个 RecyclerView 控件,它的 ID 是 city_recycler,宽度和高度都设置为 match_parent,表示填满父容器。android:layout_below="@id/city_line" 表示该控件在布局中的位置应该在 ID 为 city_line 的控件下方。android:divider="@null" 表示该 RecyclerView 不应该显示分割线。RecyclerView 是一个高度可定制化的控件,它可以用来展示列表数据,并且支持各种不同的布局方式和交互方式。
阅读全文
相关推荐
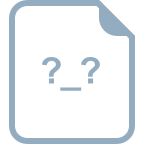





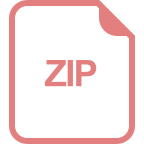
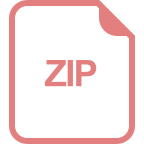
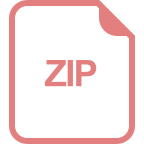
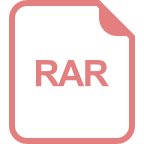
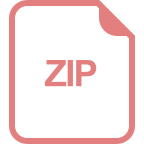





