pyqt5 视频文件窗口
时间: 2023-08-26 11:04:23 浏览: 107
您可以使用 PyQt5 中的 QMediaPlayer 和 QVideoWidget 类来创建一个视频文件窗口。下面是一个简单的示例代码:
```python
import sys
from PyQt5.QtCore import QUrl
from PyQt5.QtWidgets import QApplication, QMainWindow, QVBoxLayout, QWidget, QFileDialog
from PyQt5.QtMultimedia import QMediaPlayer, QMediaContent
from PyQt5.QtMultimediaWidgets import QVideoWidget
class VideoPlayerWindow(QMainWindow):
def __init__(self):
super().__init__()
self.setWindowTitle("Video Player")
self.setGeometry(100, 100, 800, 600)
# 创建 QMediaPlayer 和 QVideoWidget 对象
self.media_player = QMediaPlayer(self)
self.video_widget = QVideoWidget(self)
# 设置 QVideoWidget 作为中心窗口部件
layout = QVBoxLayout()
layout.addWidget(self.video_widget)
central_widget = QWidget(self)
central_widget.setLayout(layout)
self.setCentralWidget(central_widget)
# 连接 QMediaPlayer 的信号到相应的槽函数
self.media_player.setVideoOutput(self.video_widget)
def open_file(self):
# 打开视频文件对话框
file_dialog = QFileDialog()
file_dialog.setFileMode(QFileDialog.ExistingFile)
file_dialog.setNameFilters(["Videos (*.mp4 *.avi)"])
if file_dialog.exec_():
file_name = file_dialog.selectedFiles()[0]
media_content = QMediaContent(QUrl.fromLocalFile(file_name))
self.media_player.setMedia(media_content)
self.media_player.play()
if __name__ == "__main__":
app = QApplication(sys.argv)
player_window = VideoPlayerWindow()
player_window.show()
sys.exit(app.exec_())
```
这个示例代码创建了一个名为 "Video Player" 的视频播放器窗口。通过调用 `open_file` 方法,可以打开一个视频文件并将其播放在窗口中。您可以根据需要自定义窗口的大小和其他属性。请确保在运行代码之前已经安装了 PyQt5 和 PyQt5 Multimedia 模块。
阅读全文
相关推荐
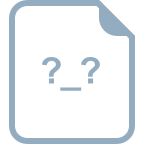
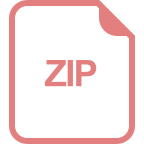














