基于java的科学计算器设计
时间: 2023-12-01 21:04:27 浏览: 31
以下是一个基于Java的科学计算器的设计示例代码,包括用户界面、表达式解析、计算功能和错误处理:
```java
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
public class ScientificCalculator extends JFrame implements ActionListener {
private JTextField inputField;
private JLabel outputLabel;
public ScientificCalculator() {
setTitle("Scientific Calculator");
setLayout(new FlowLayout());
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
inputField = new JTextField(20);
add(inputField);
JButton calculateButton = new JButton("Calculate");
calculateButton.addActionListener(this);
add(calculateButton);
outputLabel = new JLabel("Result: ");
add(outputLabel);
pack();
setLocationRelativeTo(null); // Center the window on the screen
}
@Override
public void actionPerformed(ActionEvent e) {
String input = inputField.getText();
try {
double result = evaluate(input);
outputLabel.setText("Result: " + result);
} catch (Exception ex) {
outputLabel.setText("Error: " + ex.getMessage());
}
}
private double evaluate(String input) throws Exception {
// Parse the input expression
String[] tokens = input.split(" ");
Stack<Double> operands = new Stack<>();
Stack<String> operators = new Stack<>();
for (String token : tokens) {
if (token.matches("\\d+\\.?\\d*")) {
operands.push(Double.parseDouble(token));
} else if (token.matches("[+\\-*/^]")) {
while (!operators.isEmpty() && precedence(operators.peek()) >= precedence(token)) {
evaluate(operands, operators);
}
operators.push(token);
} else if (token.equals("(")) {
operators.push(token);
} else if (token.equals(")")) {
while (!operators.peek().equals("(")) {
evaluate(operands, operators);
}
operators.pop();
} else {
throw new Exception("Invalid token: " + token);
}
}
while (!operators.isEmpty()) {
evaluate(operands, operators);
}
if (operands.size() != 1) {
throw new Exception("Invalid expression");
}
return operands.pop();
}
private void evaluate(Stack<Double> operands, Stack<String> operators) {
double b = operands.pop();
double a = operands.pop();
String operator = operators.pop();
switch (operator) {
case "+":
operands.push(a + b);
break;
case "-":
operands.push(a - b);
break;
case "*":
operands.push(a * b);
break;
case "/":
if (b == 0) {
throw new ArithmeticException("Division by zero");
}
operands.push(a / b);
break;
case "^":
operands.push(Math.pow(a, b));
break;
}
}
private int precedence(String operator) {
switch (operator) {
case "^":
return 3;
case "*":
case "/":
return 2;
case "+":
case "-":
return 1;
default:
return 0;
}
}
public static void main(String[] args) {
ScientificCalculator calculator = new ScientificCalculator();
calculator.setVisible(true);
}
}
```
这个代码实现了一个带有文本框、计算按钮和输出标签的GUI界面,当用户点击计算按钮时,程序将会调用 `evaluate` 方法来计算用户输入的表达式,并将结果显示在输出标签中。`evaluate` 方法使用了逆波兰表达式算法来解析和计算表达式,同时处理了除数为零等错误情况。
相关推荐
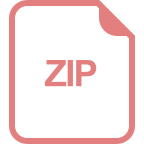
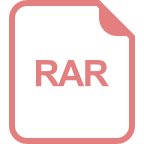














