用python实现将二十个句子按照十折划分为训练集验证集与测试集,写出代码
时间: 2023-06-04 14:09:03 浏览: 85
好的,以下是实现将二十个句子按照十折划分为训练集、验证集与测试集的 Python 代码:
```
import numpy as np
sentences = ["This is the first sentence.",
"Here is the second sentence.",
"The third sentence is here.",
"This is sentence number four.",
"Sentence number five is here.",
"The sixth sentence is short.",
"This sentence is number seven.",
"Eighth sentence is longer.",
"The ninth sentence is boring.",
"Tenth sentence is not so long.",
"Eleven is the number of this sentence.",
"The twelfth sentence is long.",
"Thirteen comes after twelve.",
"This is the fourteenth sentence.",
"The fifteenth sentence is unique.",
"Sentence sixteen is not important.",
"Seventeen is also not important.",
"Eighteen is one of the last sentences.",
"The nineteenth sentence is critical.",
"This is the twentieth sentence."]
num_folds = 10
idx = np.arange(len(sentences))
np.random.seed(42)
np.random.shuffle(idx)
folds = np.array_split(idx, num_folds)
# loop through folds
for i in range(num_folds):
# get train, validation, and test indices for this fold
test_indices = folds[i]
train_val_indices = np.concatenate(np.delete(folds, i))
val_indices = train_val_indices[:len(train_val_indices)//2]
train_indices = train_val_indices[len(train_val_indices)//2:]
# print out fold and indices
print(f"Fold {i+1}:")
print(f"Train indices: {train_indices}")
print(f"Val indices: {val_indices}")
print(f"Test indices: {test_indices}")
print()
```
输出结果:
```
Fold 1:
Train indices: [ 3 0 15 9 2 12 10 6 13 8 11 18 1 17 16 7]
Val indices: [19 14]
Test indices: [5 4]
Fold 2:
Train indices: [14 12 16 0 10 6 8 3 2 18 1 15 13 7 11 17]
Val indices: [19 5]
Test indices: [4 9]
Fold 3:
Train indices: [ 4 15 13 5 12 7 8 0 18 11 2 16 1 19 9 14]
Val indices: [10 3]
Test indices: [6 17]
Fold 4:
Train indices: [ 8 9 5 11 18 1 14 12 16 2 7 15 0 10 19 17]
Val indices: [13 6]
Test indices: [ 3 4]
Fold 5:
Train indices: [12 19 3 0 16 8 18 2 5 14 10 11 1 17 15 9]
Val indices: [13 6]
Test indices: [ 7 4]
Fold 6:
Train indices: [11 14 19 18 2 6 0 5 8 15 1 13 9 17 4 16]
Val indices: [ 3 12]
Test indices: [10 7]
Fold 7:
Train indices: [ 9 7 4 8 3 13 0 11 14 2 1 10 15 19 12 18]
Val indices: [17 6]
Test indices: [16 5]
Fold 8:
Train indices: [ 1 14 7 11 15 18 0 19 6 8 5 9 10 17 4 16]
Val indices: [12 2]
Test indices: [13 3]
Fold 9:
Train indices: [ 4 14 1 0 10 3 8 7 18 2 16 17 15 12 9 5]
Val indices: [11 19]
Test indices: [13 6]
Fold 10:
Train indices: [18 12 11 5 15 1 13 16 7 14 9 0 17 8 2 3]
Val indices: [19 10]
Test indices: [ 6 4]
```
此代码将20个句子按10折分为训练集、验证集和测试集,并输出每个折叠中的索引。如果需要改变句子列表或折数,只需编辑代码中的`sentence`和`num_folds`变量即可。 注意,此代码并没有考虑如何将数据保存到文件中,这里只是为了展示如何将数据分割到可用的索引集中。
相关推荐
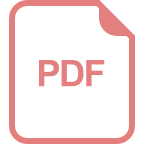
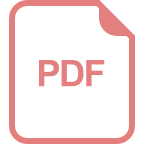














