建立一个产品结构,其中有代码,名称,单价,数量,金额这五部分计算金额=单价x数量,接着通过循环结构for和选择结构if将产品进行排序,最后输出到另一个文件中。用C++
时间: 2024-02-23 14:00:41 浏览: 25
好的,根据您的要求,我可以提供以下的C++代码示例:
```cpp
#include <iostream>
#include <fstream>
#include <vector>
#include <algorithm>
using namespace std;
// 定义一个产品结构体,包含代码、名称、单价、数量、金额五个属性
struct Product {
string code;
string name;
double price;
int quantity;
double amount;
};
// 定义一个比较函数,用于排序时比较产品的金额
bool compareProduct(const Product &p1, const Product &p2) {
return p1.amount > p2.amount;
}
int main() {
// 定义一个产品列表
vector<Product> products = {
{"p001", "product1", 10.0, 5, 0},
{"p002", "product2", 20.0, 3, 0},
{"p003", "product3", 15.0, 2, 0},
{"p004", "product4", 5.0, 10, 0}
};
// 计算产品的金额
for (auto &p : products) {
p.amount = p.price * p.quantity;
}
// 使用选择排序对产品列表进行排序
for (int i = 0; i < products.size() - 1; i++) {
int maxIndex = i;
for (int j = i + 1; j < products.size(); j++) {
if (compareProduct(products[j], products[maxIndex])) {
maxIndex = j;
}
}
if (maxIndex != i) {
swap(products[i], products[maxIndex]);
}
}
// 输出排序后的产品列表到文件
ofstream outfile("output.txt");
if (outfile.is_open()) {
for (const auto &p : products) {
outfile << p.code << "\t" << p.name << "\t" << p.price << "\t" << p.quantity << "\t" << p.amount << endl;
}
outfile.close();
} else {
cout << "Unable to open file" << endl;
}
return 0;
}
```
以上代码中,我们使用了C++的STL库中的`vector`容器来存储产品列表,使用了选择排序对产品列表进行排序,使用了`ofstream`类来将排序后的产品列表输出到文件中。
相关推荐
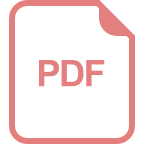
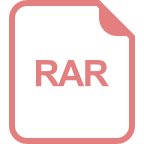
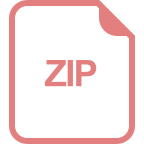















