SemicircleProgressBar 实现渐变色
时间: 2023-06-19 22:06:36 浏览: 170
要实现SemicircleProgressBar的渐变色,可以使用Android自带的GradientDrawable类。GradientDrawable可以创建渐变色和形状的Drawable对象,可以通过设置渐变色的起始颜色和结束颜色、渐变方向等属性来实现。
以下是一个示例代码:
```java
// 创建渐变色
int[] colors = {Color.parseColor("#FF4081"), Color.parseColor("#3F51B5")};
GradientDrawable gradient = new GradientDrawable(GradientDrawable.Orientation.BL_TR, colors);
gradient.setGradientType(GradientDrawable.LINEAR_GRADIENT);
// 设置渐变色为进度条的背景
progressBar.setBackground(gradient);
```
在上述示例代码中,我们首先创建了一个渐变色,其中起始颜色为#FF4081,结束颜色为#3F51B5,渐变方向为从左下到右上。然后,我们将渐变色设置为进度条的背景。
你可以根据需要调整渐变色的颜色和方向,以满足你的需求。
相关问题
SemiCircleProgressBar 实现代码 支持渐变色
以下是一个简单的实现 SemiCircleProgressBar 并支持渐变色的示例代码,使用了 Canvas 绘制。
```java
public class SemiCircleProgressBar extends View {
private static final int DEFAULT_START_COLOR = Color.parseColor("#FFC107");
private static final int DEFAULT_END_COLOR = Color.parseColor("#FF5722");
private static final int DEFAULT_ANIM_DURATION = 1000;
private int startColor;
private int endColor;
private int animDuration;
private float progress;
private Paint paint;
private RectF rectF;
public SemiCircleProgressBar(Context context) {
this(context, null);
}
public SemiCircleProgressBar(Context context, AttributeSet attrs) {
super(context, attrs);
TypedArray ta = context.obtainStyledAttributes(attrs, R.styleable.SemiCircleProgressBar);
startColor = ta.getColor(R.styleable.SemiCircleProgressBar_startColor, DEFAULT_START_COLOR);
endColor = ta.getColor(R.styleable.SemiCircleProgressBar_endColor, DEFAULT_END_COLOR);
animDuration = ta.getInteger(R.styleable.SemiCircleProgressBar_animDuration, DEFAULT_ANIM_DURATION);
ta.recycle();
init();
}
private void init() {
paint = new Paint();
paint.setAntiAlias(true);
paint.setStyle(Paint.Style.STROKE);
paint.setStrokeWidth(10);
rectF = new RectF();
}
public void setProgress(float progress) {
this.progress = progress;
invalidate();
}
@Override
protected void onDraw(Canvas canvas) {
super.onDraw(canvas);
int width = getWidth();
int height = getHeight();
int centerX = width / 2;
int centerY = height / 2;
int radius = Math.min(centerX, centerY) - 20;
rectF.set(centerX - radius, centerY - radius, centerX + radius, centerY + radius);
LinearGradient gradient = new LinearGradient(0, 0, width, 0, startColor, endColor, Shader.TileMode.CLAMP);
paint.setShader(gradient);
canvas.drawArc(rectF, 180, 180 * progress, false, paint);
}
public void startAnim() {
ValueAnimator animator = ValueAnimator.ofFloat(0, 1);
animator.setDuration(animDuration);
animator.addUpdateListener(new ValueAnimator.AnimatorUpdateListener() {
@Override
public void onAnimationUpdate(ValueAnimator animation) {
setProgress((float) animation.getAnimatedValue());
}
});
animator.start();
}
}
```
在布局文件中使用:
```xml
<com.example.SemiCircleProgressBar
android:layout_width="200dp"
android:layout_height="200dp"
app:startColor="#FFC107"
app:endColor="#FF5722"
app:animDuration="1000" />
```
其中,`startColor` 和 `endColor` 分别表示渐变色的起始颜色和结束颜色,`animDuration` 表示动画的时长。可以通过 `setProgress` 方法设置进度,通过 `startAnim` 方法开始动画。
SemiCircleProgressBar 实现并支持渐变
实现一个支持渐变的半圆形进度条可以使用自定义 View 的方式,主要思路如下:
1. 继承 View,重写 onDraw 方法,在方法中绘制半圆形和进度条。
2. 在 onDraw 方法中使用 Paint 绘制半圆形和进度条。绘制半圆形可以使用 drawArc 方法,绘制渐变进度条可以使用 Shader。
3. 创建一个 SweepGradient 对象,设置渐变的颜色和位置。
4. 将 SweepGradient 对象设置给 Paint 对象的 Shader 属性,使得进度条使用渐变颜色绘制。
下面是一个简单的实现示例:
```java
public class SemiCircleProgressBar extends View {
private static final int DEFAULT_START_ANGLE = 180;
private static final int DEFAULT_SWEEP_ANGLE = 180;
private Paint mBackgroundPaint;
private Paint mProgressPaint;
private int mStartAngle = DEFAULT_START_ANGLE;
private int mSweepAngle = DEFAULT_SWEEP_ANGLE;
private int mProgressColor = Color.RED;
private int mBackgroundColor = Color.GRAY;
public SemiCircleProgressBar(Context context) {
super(context);
init();
}
public SemiCircleProgressBar(Context context, AttributeSet attrs) {
super(context, attrs);
init();
}
public SemiCircleProgressBar(Context context, AttributeSet attrs, int defStyleAttr) {
super(context, attrs, defStyleAttr);
init();
}
private void init() {
mBackgroundPaint = new Paint();
mBackgroundPaint.setAntiAlias(true);
mBackgroundPaint.setColor(mBackgroundColor);
mBackgroundPaint.setStyle(Paint.Style.STROKE);
mProgressPaint = new Paint();
mProgressPaint.setAntiAlias(true);
mProgressPaint.setStyle(Paint.Style.STROKE);
mProgressPaint.setStrokeCap(Paint.Cap.ROUND);
}
@Override
protected void onDraw(Canvas canvas) {
super.onDraw(canvas);
int width = getWidth();
int height = getHeight();
int strokeWidth = Math.min(width, height) / 10;
mBackgroundPaint.setStrokeWidth(strokeWidth);
mProgressPaint.setStrokeWidth(strokeWidth);
RectF rectF = new RectF(strokeWidth / 2, strokeWidth / 2,
width - strokeWidth / 2, height - strokeWidth / 2);
// 绘制半圆形背景
canvas.drawArc(rectF, mStartAngle, mSweepAngle, false, mBackgroundPaint);
// 绘制渐变进度条
int[] colors = {Color.GREEN, Color.YELLOW, Color.RED};
float[] positions = {0f, 0.5f, 1f};
SweepGradient sweepGradient = new SweepGradient(width / 2, height / 2,
colors, positions);
mProgressPaint.setShader(sweepGradient);
canvas.drawArc(rectF, mStartAngle, mSweepAngle, false, mProgressPaint);
}
public void setProgressColor(int progressColor) {
mProgressColor = progressColor;
mProgressPaint.setColor(mProgressColor);
invalidate();
}
public void setBackgroundColor(int backgroundColor) {
mBackgroundColor = backgroundColor;
mBackgroundPaint.setColor(mBackgroundColor);
invalidate();
}
public void setStartAngle(int startAngle) {
mStartAngle = startAngle;
invalidate();
}
public void setSweepAngle(int sweepAngle) {
mSweepAngle = sweepAngle;
invalidate();
}
}
```
在代码中,我们使用了 SweepGradient 实现渐变,设置了颜色和位置,然后将 SweepGradient 对象设置给进度条的 Paint 对象的 Shader 属性。最终我们可以得到一个支持渐变的半圆形进度条。
阅读全文
相关推荐
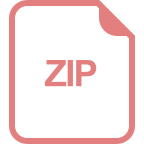
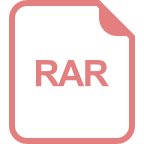
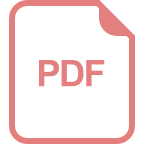
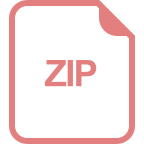
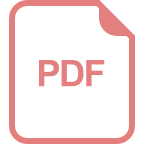
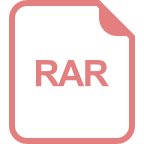
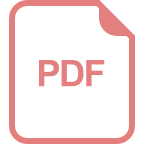
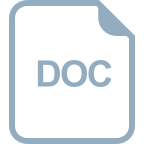
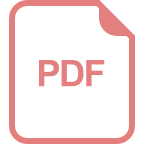
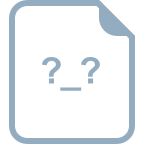
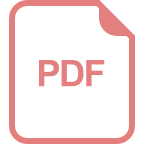
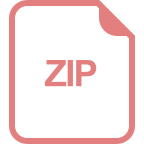