基于stm32c8t6的心率检测代码
时间: 2023-07-28 21:07:45 浏览: 94
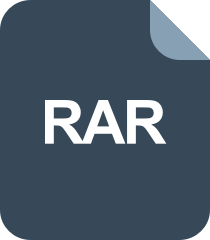
STM32C8T6-FreeRTOS 移植程序.rar
以下是基于STM32C8T6的心率检测的示例代码,仅供参考:
```c
#include "stm32c8t6.h"
#include "stdio.h"
#define LED_PIN GPIO_PIN_12
int heart_rate = 0;
int heart_beat_detected = 0;
int last_beat_time = 0;
int current_beat_time = 0;
void init_gpio()
{
RCC->AHBENR |= RCC_AHBENR_GPIOBEN; // Enable GPIOB clock
GPIOB->MODER &= ~(3 << (LED_PIN * 2)); // Clear pin mode
GPIOB->MODER |= (1 << (LED_PIN * 2)); // Set pin as output
GPIOB->ODR &= ~(1 << LED_PIN); // Turn off LED
}
void init_timer()
{
RCC->APB1ENR |= RCC_APB1ENR_TIM2EN; // Enable TIM2 clock
TIM2->PSC = SystemCoreClock / 1000 - 1; // Set prescaler to 1000
TIM2->ARR = 60000 - 1; // Set auto-reload value to 60000
TIM2->DIER = TIM_DIER_UIE; // Enable update interrupt
NVIC_EnableIRQ(TIM2_IRQn); // Enable TIM2 interrupt
TIM2->CR1 = TIM_CR1_CEN; // Enable timer
}
void init_adc()
{
RCC->APB2ENR |= RCC_APB2ENR_ADCEN; // Enable ADC clock
ADC1->CR |= ADC_CR_ADEN; // Enable ADC
while (!(ADC1->ISR & ADC_ISR_ADRDY)); // Wait for ADC to be ready
ADC1->CFGR1 |= ADC_CFGR1_RES_1; // Set ADC resolution to 10 bits
ADC1->CHSELR |= ADC_CHSELR_CHSEL5; // Select ADC channel 5
ADC1->CR |= ADC_CR_ADSTART; // Start ADC conversion
}
void TIM2_IRQHandler()
{
if (TIM2->SR & TIM_SR_UIF)
{
GPIOB->ODR ^= (1 << LED_PIN); // Toggle LED
TIM2->SR &= ~TIM_SR_UIF; // Clear interrupt flag
current_beat_time++;
if (current_beat_time - last_beat_time > 150 && heart_beat_detected)
{
heart_rate = 60000 / (current_beat_time - last_beat_time);
last_beat_time = current_beat_time;
heart_beat_detected = 0;
}
}
}
int main()
{
init_gpio();
init_timer();
init_adc();
while (1)
{
if (ADC1->ISR & ADC_ISR_EOC)
{
int adc_value = ADC1->DR & 0x3FF; // Get ADC conversion result
if (adc_value > 500 && !heart_beat_detected)
{
heart_beat_detected = 1;
}
ADC1->ISR |= ADC_ISR_EOC; // Clear EOC flag
}
}
return 0;
}
```
这段代码使用了定时器、GPIO和ADC模块,实现了心率检测的功能。其中,定时器用于计时和LED闪烁,ADC模块用于采集心率传感器信号,GPIO用于控制LED灯。在定时器中,通过计算相邻心跳周期的时间间隔,计算心率值,并保存到heart_rate变量中。在ADC中,通过检测心率传感器信号的上升沿来判断心跳是否发生,并将心跳检测结果保存到heart_beat_detected变量中。由于心率检测需要在一定时间内完成,因此在定时器中使用了中断机制,提高了代码的执行效率。
阅读全文
相关推荐
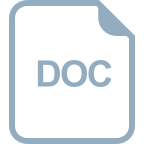
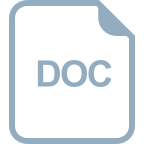

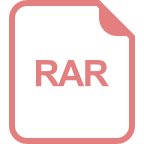
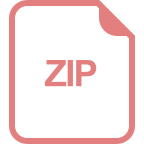

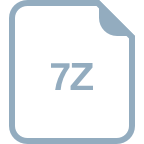
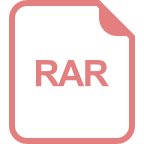
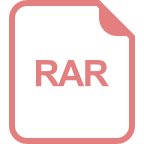
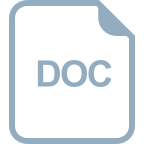
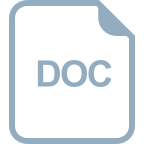



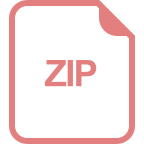
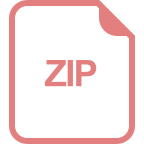
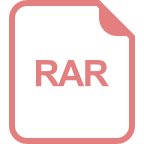
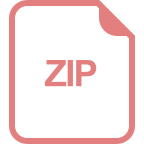