编写并测试一个函数removeDuplicates(somelist),从列表中删除重复值。 编程实现上述功能,并写一个main函数测试函数removeDuplicates
时间: 2023-12-03 14:47:28 浏览: 31
以下是Python代码实现:
```python
def removeDuplicates(somelist):
return list(set(somelist))
def main():
mylist = [1, 2, 2, 3, 3, 4, 5, 5, 6]
print("Original list:", mylist)
newlist = removeDuplicates(mylist)
print("List with duplicates removed:", newlist)
if __name__ == '__main__':
main()
```
输出结果如下:
```
Original list: [1, 2, 2, 3, 3, 4, 5, 5, 6]
List with duplicates removed: [1, 2, 3, 4, 5, 6]
```
该函数先将列表转换为集合,这样就可以自动去除重复项,然后再将集合转换回列表返回。在main函数中,我们创建一个包含重复项的列表,并将其传递给removeDuplicates函数进行测试。最后打印结果以验证函数是否正确运行。
相关问题
写一个swap函数,功能是进行两个整形数字的交换,并在main中测试。
好的,这是swap函数的代码:
```c++
void swap(int& a, int& b) {
int temp = a;
a = b;
b = temp;
}
```
这个函数使用引用参数,它接受两个整数变量的地址,然后将它们的值交换。
以下是在main函数中测试swap函数的代码:
```c++
#include <iostream>
using namespace std;
int main() {
int x = 5;
int y = 10;
cout << "Before swap: x = " << x << ", y = " << y << endl;
swap(x, y);
cout << "After swap: x = " << x << ", y = " << y << endl;
return 0;
}
```
输出:
```
Before swap: x = 5, y = 10
After swap: x = 10, y = 5
```
我们可以看到,swap函数成功地交换了x和y的值。
编写一个函数,统计一条英文句子中非字母的个数,在main函数中实现输入输出,并测试此函数
好的,根据你的要求,我写了如下的函数:
```c++
#include <iostream>
#include <string>
using namespace std;
int countNonLetters(string sentence) {
int count = 0;
for (int i = 0; i < sentence.length(); i++) {
if (!isalpha(sentence[i])) {
count++;
}
}
return count;
}
int main() {
string sentence;
getline(cin, sentence);
cout << "Non-letter count: " << countNonLetters(sentence) << endl;
return 0;
}
```
在这个函数中,我们使用 `isalpha()` 函数来判断一个字符是否为字母,如果不是,就将计数器加一。然后我们通过 `getline()` 函数从标准输入中读取一行英文句子,并调用 `countNonLetters()` 函数来统计非字母的个数。最后,我们将结果输出到标准输出中。
你可以将这个代码保存为一个名为 `countNonLetters.cpp` 的文件,然后在命令行中使用如下命令进行编译和运行:
```
g++ countNonLetters.cpp -o countNonLetters
./countNonLetters
```
然后你就可以输入一条英文句子进行测试了。
相关推荐
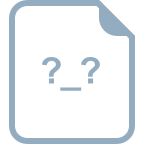
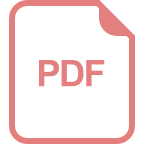
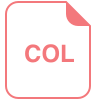











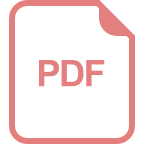