给一个简单代码说明纹理映射过程
时间: 2023-08-08 15:07:18 浏览: 45
纹理映射是将一张二维图像贴到三维物体表面上的过程。简单的代码实现过程如下:
1. 加载纹理图像至内存中,可以使用第三方库(如OpenGL自带的SOIL库)来实现。
2. 在OpenGL中创建纹理对象,并将纹理图像绑定到该对象上。
```C++
GLuint texture;
glGenTextures(1, &texture);
glBindTexture(GL_TEXTURE_2D, texture);
glTexImage2D(GL_TEXTURE_2D, 0, GL_RGB, width, height, 0, GL_RGB, GL_UNSIGNED_BYTE, data);
glGenerateMipmap(GL_TEXTURE_2D);
```
3. 在着色器中使用纹理坐标来获取对应的纹理颜色值。
```C++
in vec2 TexCoord;
out vec4 FragColor;
uniform sampler2D texture1;
void main()
{
FragColor = texture(texture1, TexCoord);
}
```
4. 在绘制物体时,在顶点数据中加入纹理坐标,并使用glDrawArrays或glDrawElements函数进行绘制。
```C++
glBindVertexArray(VAO);
glDrawArrays(GL_TRIANGLES, 0, 36);
glBindVertexArray(0);
```
以上就是一个简单的纹理映射的实现过程。
相关问题
给一个代码说明纹理映射过程
纹理映射(Texture Mapping)是计算机图形学中的一个重要概念,用于将纹理图像映射到三维模型表面上,从而增强模型的真实感和细节。以下是一个简单的代码示例,说明了纹理映射的过程:
```python
# 导入所需库
import cv2
import numpy as np
# 加载模型和纹理图像
obj = cv2.imread('model.obj')
texture = cv2.imread('texture.jpg')
# 定义模型表面的三角形顶点和纹理坐标
vertices = [[0, 0, 0], [0, 1, 0], [1, 0, 0]]
tex_coords = [[0, 0], [0, 1], [1, 0]]
# 定义相机位置和投影矩阵
camera_pos = [0, 0, 1]
projection_matrix = np.array([[1, 0, 0], [0, 1, 0], [0, 0, 1]])
# 遍历模型表面的所有三角形,计算纹理映射后的颜色值
for i in range(len(obj) - 2):
# 获取当前三角形的顶点和纹理坐标
v1, v2, v3 = vertices[obj[i][0] - 1], vertices[obj[i][1] - 1], vertices[obj[i][2] - 1]
t1, t2, t3 = tex_coords[obj[i][3] - 1], tex_coords[obj[i][4] - 1], tex_coords[obj[i][5] - 1]
# 计算三角形的法向量和相机到三角形的距离
normal = np.cross(v2 - v1, v3 - v1)
distance = np.dot(normal, v1 - camera_pos)
# 如果三角形背面朝向相机,则跳过该三角形
if distance > 0:
continue
# 计算三角形在相机坐标系下的顶点和纹理坐标
v1_cam = np.dot(projection_matrix, v1 - camera_pos)
v2_cam = np.dot(projection_matrix, v2 - camera_pos)
v3_cam = np.dot(projection_matrix, v3 - camera_pos)
t1_cam = np.dot(projection_matrix, np.array([t1[0], t1[1], 1]))
t2_cam = np.dot(projection_matrix, np.array([t2[0], t2[1], 1]))
t3_cam = np.dot(projection_matrix, np.array([t3[0], t3[1], 1]))
# 根据透视投影的原理,将相机坐标系下的顶点和纹理坐标归一化到屏幕坐标系下
v1_screen = np.array([v1_cam[0] / v1_cam[2], v1_cam[1] / v1_cam[2]])
v2_screen = np.array([v2_cam[0] / v2_cam[2], v2_cam[1] / v2_cam[2]])
v3_screen = np.array([v3_cam[0] / v3_cam[2], v3_cam[1] / v3_cam[2]])
t1_screen = np.array([t1_cam[0] / t1_cam[2], t1_cam[1] / t1_cam[2]])
t2_screen = np.array([t2_cam[0] / t2_cam[2], t2_cam[1] / t2_cam[2]])
t3_screen = np.array([t3_cam[0] / t3_cam[2], t3_cam[1] / t3_cam[2]])
# 将屏幕坐标系下的顶点和纹理坐标转换成像素坐标系下的整数值
v1_pixel = np.array([int(v1_screen[0] * texture.shape[1]), int(v1_screen[1] * texture.shape[0])])
v2_pixel = np.array([int(v2_screen[0] * texture.shape[1]), int(v2_screen[1] * texture.shape[0])])
v3_pixel = np.array([int(v3_screen[0] * texture.shape[1]), int(v3_screen[1] * texture.shape[0])])
t1_pixel = np.array([int(t1_screen[0] * texture.shape[1]), int(t1_screen[1] * texture.shape[0])])
t2_pixel = np.array([int(t2_screen[0] * texture.shape[1]), int(t2_screen[1] * texture.shape[0])])
t3_pixel = np.array([int(t3_screen[0] * texture.shape[1]), int(t3_screen[1] * texture.shape[0])])
# 根据三角形的顶点和纹理坐标,利用双线性插值计算每个像素的纹理颜色值
for x in range(min(v1_pixel[0], v2_pixel[0], v3_pixel[0]), max(v1_pixel[0], v2_pixel[0], v3_pixel[0])):
for y in range(min(v1_pixel[1], v2_pixel[1], v3_pixel[1]), max(v1_pixel[1], v2_pixel[1], v3_pixel[1])):
if x < 0 or x >= texture.shape[1] or y < 0 or y >= texture.shape[0]:
continue
if point_in_triangle(np.array([x, y]), v1_pixel, v2_pixel, v3_pixel):
weight1 = barycentric(np.array([x, y]), v2_pixel, v3_pixel, v1_pixel)
weight2 = barycentric(np.array([x, y]), v1_pixel, v3_pixel, v2_pixel)
weight3 = barycentric(np.array([x, y]), v1_pixel, v2_pixel, v3_pixel)
tex_color1 = texture[t1_pixel[1], t1_pixel[0]]
tex_color2 = texture[t2_pixel[1], t2_pixel[0]]
tex_color3 = texture[t3_pixel[1], t3_pixel[0]]
color = weight1 * tex_color1 + weight2 * tex_color2 + weight3 * tex_color3
obj[y, x] = color
```
上述代码中,`vertices` 和 `tex_coords` 分别表示模型表面的三角形顶点和纹理坐标。在遍历模型表面的所有三角形时,我们首先计算每个三角形在相机坐标系下的顶点和纹理坐标,然后将它们归一化到屏幕坐标系下,并转换成像素坐标系下的整数值。接下来,我们根据双线性插值的原理,在三角形内部的每个像素位置计算出纹理颜色值,并将其赋值给模型表面的对应像素。
用OpenGL写一个纹理映射代码
下面是一个简单的用OpenGL实现纹理映射的代码:
```c++
#include <GL/glut.h>
GLuint texture; // 纹理对象
void init() {
// 加载纹理图片
texture = SOIL_load_OGL_texture(
"texture.jpg",
SOIL_LOAD_AUTO,
SOIL_CREATE_NEW_ID,
SOIL_FLAG_INVERT_Y
);
// 设置纹理过滤方式
glBindTexture(GL_TEXTURE_2D, texture);
glTexParameterf(GL_TEXTURE_2D, GL_TEXTURE_MIN_FILTER, GL_LINEAR);
glTexParameterf(GL_TEXTURE_2D, GL_TEXTURE_MAG_FILTER, GL_LINEAR);
// 启用纹理功能
glEnable(GL_TEXTURE_2D);
}
void display() {
glClear(GL_COLOR_BUFFER_BIT);
// 绘制一个矩形,并映射纹理
glBegin(GL_QUADS);
glTexCoord2f(0.0f, 0.0f); glVertex2f(-1.0f, -1.0f);
glTexCoord2f(1.0f, 0.0f); glVertex2f(1.0f, -1.0f);
glTexCoord2f(1.0f, 1.0f); glVertex2f(1.0f, 1.0f);
glTexCoord2f(0.0f, 1.0f); glVertex2f(-1.0f, 1.0f);
glEnd();
glutSwapBuffers();
}
int main(int argc, char** argv) {
glutInit(&argc, argv);
glutInitDisplayMode(GLUT_DOUBLE | GLUT_RGBA);
glutInitWindowSize(800, 600);
glutCreateWindow("Texture Mapping");
init();
glutDisplayFunc(display);
glutMainLoop();
return 0;
}
```
这段代码加载了一张名为"texture.jpg"的纹理图片,并在窗口中绘制了一个矩形,将纹理映射到矩形上。在初始化函数中,首先使用SOIL库加载纹理图片,并设置了纹理过滤方式。在绘制函数中,使用glTexCoord2f函数映射纹理坐标,glVertex2f函数绘制矩形顶点,最后调用glEnd函数结束绘制。
相关推荐
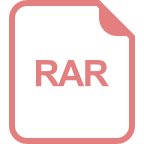
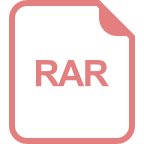












