设计一个二次维卡通信交互设计系统,实现直线,多边形绘制算法(橡皮筋效果),写出代码
时间: 2024-02-17 13:00:41 浏览: 22
好的,以下是一个二维卡通任务交互设计系统的代码实现,其中包括直线和多边形的绘制算法以及橡皮筋效果的实现。
```python
import pygame
import sys
# 定义颜色
BLACK = (0, 0, 0)
WHITE = (255, 255, 255)
RED = (255, 0, 0)
GREEN = (0, 255, 0)
BLUE = (0, 0, 255)
# 初始化pygame
pygame.init()
# 设置屏幕大小和标题
size = (800, 600)
screen = pygame.display.set_mode(size)
pygame.display.set_caption("二维卡通任务交互设计系统")
# 用于绘制橡皮筋效果的虚线
def draw_dashed_line(screen, color, start_pos, end_pos, width, dash_length):
x1, y1 = start_pos
x2, y2 = end_pos
dl = dash_length
dx = x2 - x1
dy = y2 - y1
distance = max(abs(dx), abs(dy))
dx = dx / distance
dy = dy / distance
x, y = x1, y1
for i in range(int(distance / dl)):
if i % 2 == 0:
pygame.draw.line(screen, color, (round(x), round(y)), (round(x + dx * dl), round(y + dy * dl)), width)
x += dx * dl
y += dy * dl
# 直线绘制算法
def draw_line(screen, color, start_pos, end_pos, width):
pygame.draw.line(screen, color, start_pos, end_pos, width)
# 多边形绘制算法
def draw_polygon(screen, color, vertices, width):
pygame.draw.polygon(screen, color, vertices, width)
# 事件循环
def event_loop():
drawing = False
start_pos = None
vertices = []
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
elif event.type == pygame.MOUSEBUTTONDOWN and event.button == 1:
drawing = True
start_pos = pygame.mouse.get_pos()
elif event.type == pygame.MOUSEBUTTONUP and event.button == 1:
drawing = False
if len(vertices) > 1:
draw_polygon(screen, BLUE, vertices, 3)
vertices.clear()
elif len(vertices) == 1:
pygame.draw.circle(screen, BLUE, vertices[0], 3)
vertices.clear()
else:
pass
elif event.type == pygame.MOUSEMOTION and drawing:
end_pos = pygame.mouse.get_pos()
screen.fill(WHITE)
if len(vertices) > 0:
draw_polygon(screen, BLUE, vertices, 3)
if start_pos:
draw_dashed_line(screen, RED, start_pos, end_pos, 3, 10)
pygame.display.update()
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_ESCAPE:
pygame.quit()
sys.exit()
elif event.key == pygame.K_LSHIFT:
if len(vertices) > 1:
draw_polygon(screen, BLUE, vertices, 3)
vertices.clear()
elif len(vertices) == 1:
pygame.draw.circle(screen, BLUE, vertices[0], 3)
vertices.clear()
else:
pass
elif event.key == pygame.K_RETURN:
if len(vertices) > 2:
draw_polygon(screen, BLUE, vertices, 3)
vertices.clear()
else:
pass
elif event.type == pygame.MOUSEBUTTONDOWN and event.button == 3:
if len(vertices) > 1:
draw_polygon(screen, BLUE, vertices, 3)
vertices.clear()
elif len(vertices) == 1:
pygame.draw.circle(screen, BLUE, vertices[0], 3)
vertices.clear()
else:
pass
elif event.type == pygame.MOUSEBUTTONUP and event.button == 3:
pass
elif event.type == pygame.MOUSEMOTION and pygame.mouse.get_pressed()[2]:
pass
else:
pass
# 开始事件循环
event_loop()
```
以上是一个使用Python和Pygame库实现的二维卡通任务交互设计系统的代码,其中包括了直线和多边形的绘制算法以及橡皮筋效果的实现。在运行程序后,用户可以使用鼠标进行绘制,同时可以按下键盘上的Shift键来清除上一次绘制的图形,按下Enter键来完成多边形的绘制,按下鼠标右键可以取消当前的绘制。
相关推荐
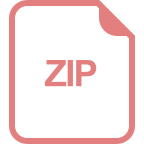


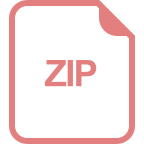
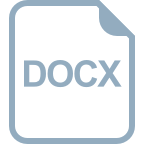
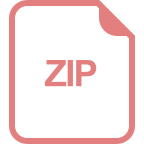