vue typescript 父组件调用子组件方法
时间: 2023-11-19 11:54:30 浏览: 60
在Vue TypeScript中,父组件可以通过ref引用子组件,并调用子组件中的方法。具体步骤如下:
1. 在子组件中定义需要调用的方法。
2. 在父组件中使用ref引用子组件,并在方法中通过ref调用子组件中的方法。
需要注意的是,在使用ref引用子组件时,需要在子组件的定义中添加ref属性,例如:`<son-com ref="sonRef"/>`。此外,如果需要在TS中使用ref,可以使用`const sonRef = ref<null | HTMLElement>(null);`进行定义。
另外,子组件也可以通过$emit将需要传递的数据传递给父组件,从而实现子传父。具体步骤可以参考引用中的方法1和方法2。
相关问题
vue ts父组件调用子组件方法,但是声明的类型没有
在Vue中,如果父组件需要调用子组件的方法,可以通过在父组件中使用`$refs`来获取对子组件的引用,然后通过这个引用来调用子组件的方法。在这个过程中,需要在子组件中声明一个公共的方法,并且在父组件中定义一个类型,来匹配子组件中的方法。
如果父组件调用子组件方法声明的类型没有,则需要检查子组件中的方法是否与声明的类型一致。如果不一致,需要修改子组件中的方法,使其与声明的类型匹配。
在使用TypeScript开发Vue应用时,可以通过在Vue组件中定义`$refs`属性的类型来匹配子组件的类型,例如:
```typescript
import { Component, Vue } from 'vue-property-decorator';
import ChildComponent from '@/components/ChildComponent.vue';
@Component({
components: { ChildComponent },
})
export default class ParentComponent extends Vue {
// 定义$refs属性的类型
$refs!: {
childComponent: ChildComponent;
};
// 在父组件中调用子组件方法
handleClick() {
this.$refs.childComponent.methodInChildComponent();
}
}
```
需要注意的是,在使用`$refs`时需要确保子组件已经被挂载,否则引用会返回`undefined`。可以在生命周期函数`mounted()`中调用子组件方法,或者在需要使用子组件方法的时候通过`$nextTick()`来确保子组件已经被挂载。
vue3 ts 子组件调用父组件方法
在Vue3中,子组件调用父组件的方法可以通过使用`$emit`来实现。下面是一个示例:
在父组件中,定义一个方法:
```typescript
methods: {
parentMethod() {
console.log("父组件方法被调用");
}
}
```
在子组件中,通过 `$emit`触发父组件的方法:
```typescript
methods: {
childMethod() {
this.$emit("methodName");
}
}
```
在模板中,将子组件的方法和父组件的方法绑定:
```html
<template>
<div>
<button @click="childMethod">调用父组件方法</button>
</div>
</template>
```
这样当子组件的方法被调用时,会触发父组件的方法。
相关推荐
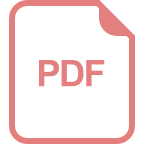
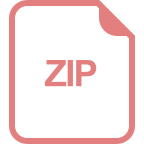
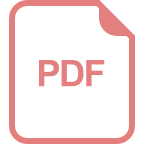
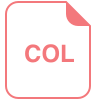
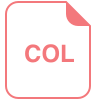
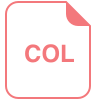









