制作一个沙盒冒险生存游戏
时间: 2023-10-19 16:05:23 浏览: 45
要制作一个沙盒冒险生存游戏,需要考虑以下几个方面:
1. 游戏世界的设定和背景故事:游戏的世界观和故事情节能够吸引玩家,让他们投入游戏中。
2. 游戏玩法的设计:沙盒游戏的核心是自由度,需要为玩家提供丰富的玩法,例如建造、探索、收集、制作等。
3. 游戏的艺术风格和音效设计:良好的艺术风格和音效能够增强游戏的代入感和沉浸感。
4. 游戏的优化和稳定性:游戏的稳定性和流畅度是玩家体验的关键因素,需要注重游戏的优化。
5. 游戏的社交和互动功能:多人游戏的社交和互动功能能够增加游戏的趣味性和互动性。
以上是制作沙盒冒险生存游戏需要考虑的几个方面,如果你需要更详细的信息或者有其他问题,请告诉我。
相关问题
我需要制作一个沙盒冒险生存游戏的代码
制作沙盒冒险生存游戏的代码需要考虑游戏的功能和玩法,这里提供一些常见的游戏功能和对应的代码:
1. 玩家移动和操作
```python
# 玩家移动
def move_player(direction):
if direction == 'up':
player_y -= 1
elif direction == 'down':
player_y += 1
elif direction == 'left':
player_x -= 1
elif direction == 'right':
player_x += 1
# 玩家操作
def player_action(action):
if action == 'collect':
collect_resource(player_x, player_y)
elif action == 'build':
build_structure(player_x, player_y)
elif action == 'attack':
attack_enemy(player_x, player_y)
```
2. 资源采集和制作
```python
# 资源采集
def collect_resource(x, y):
resource = get_resource(x, y)
if resource:
player_inventory.add_resource(resource)
# 制作物品
def craft_item(item):
for recipe in item_recipes:
if recipe['item'] == item and recipe['resources'] in player_inventory:
player_inventory.remove_resources(recipe['resources'])
player_inventory.add_item(item)
break
```
3. 建造和拆除结构
```python
# 建造结构
def build_structure(x, y, structure_type):
if player_inventory.has_resources(structure_type.resources):
player_inventory.remove_resources(structure_type.resources)
structures.add(Structure(x, y, structure_type))
# 拆除结构
def destroy_structure(x, y):
for structure in structures:
if structure.x == x and structure.y == y:
player_inventory.add_resources(structure.resources)
structures.remove(structure)
break
```
4. 敌人生成和AI
```python
# 敌人生成
def spawn_enemy():
enemy_type = random.choice(enemy_types)
x, y = get_random_location()
enemies.add(Enemy(x, y, enemy_type))
# 敌人AI
def enemy_ai(enemy):
if player_distance(enemy) < enemy_type.attack_range:
attack_player(enemy)
else:
move_enemy_towards_player(enemy)
```
5. 多人游戏和网络通信
```python
# 网络通信
def send_data(data):
connection.sendall(data.encode())
def receive_data():
data = connection.recv(1024).decode()
return data
# 多人游戏
def multiplayer_game():
while True:
data = receive_data()
# 处理游戏数据
send_data(game_state)
```
以上是一些常见的游戏功能和对应的代码,你可以根据自己的需求进行修改和扩展。注意,在实际开发中,还需要考虑代码的组织结构、错误处理、调试和测试等方面。
Dev c++怎么创造一个沙盒游戏
要创建一个沙盒游戏,您需要遵循以下步骤:
1.确定游戏类型:沙盒游戏可以是建造游戏、模拟游戏或冒险游戏等。您需要确定您的游戏类型,并根据需要为其设计游戏机制和规则。
2.设计游戏世界:您需要设计游戏世界的环境、地形和生物群落。您可以使用3D建模软件来创建游戏中的物体和场景。
3.添加游戏元素:您需要添加各种游戏元素,例如角色、物品、任务和技能等。您还需要确定这些元素之间的关系和交互方式。
4.编写游戏代码:使用Dev C++等编程软件编写游戏代码。您需要使用游戏引擎或框架来简化游戏开发过程。
5.测试游戏:测试您的游戏以检查其是否运行良好。您需要修复任何错误和漏洞,并进行性能测试以确保游戏流畅。
6.发布游戏:最后,您需要将游戏发布到适当的平台上,例如Steam、App Store或Google Play等。
请注意,创建一个沙盒游戏需要大量的时间和努力,因此请确保您有足够的资源和经验来完成它。
相关推荐
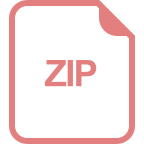












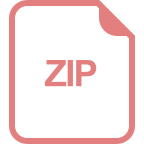