车辆调度问题matlab
时间: 2023-11-02 19:54:06 浏览: 40
车辆调度问题是一个复杂的优化问题,可以使用MATLAB进行求解。一般来说,车辆调度问题分为两个部分:路径规划和任务分配。路径规划是确定每个车辆应该走哪条路径,任务分配是将任务分配给不同的车辆。
在MATLAB中,可以使用线性规划、整数规划、遗传算法等方法来求解车辆调度问题。其中,线性规划和整数规划是基于数学模型的方法,可以求解较小规模的问题;而遗传算法是基于进化算法的方法,适用于求解较大规模的问题。
如果你想深入研究车辆调度问题,建议你先了解相关的数学模型和算法,然后在MATLAB中实现求解。同时,也可以考虑使用一些专业的求解软件,如CPLEX、Gurobi等。
相关问题
车辆调度问题matlab程序
车辆调度问题是一个经典的优化问题,其主要目标是将一组车辆分配到一系列任务中,使得任务完成时间最短、车辆的使用最优。针对这个问题,可以使用各种不同的优化算法进行求解,比如遗传算法、粒子群算法、蚁群算法等等。
以下是一个基于遗传算法的车辆调度问题的 MATLAB 程序:
```matlab
% 车辆调度问题遗传算法求解程序
% 车辆和任务的数量
num_vehicles = 5;
num_tasks = 10;
% 距离矩阵,表示任意两个任务之间的距离
dist_matrix = rand(num_tasks, num_tasks);
% 初始化种群
pop_size = 50;
pop = zeros(pop_size, num_tasks);
for i = 1:pop_size
pop(i, :) = randperm(num_tasks);
end
% 迭代次数和交叉率、变异率
num_generations = 100;
crossover_rate = 0.8;
mutation_rate = 0.2;
% 开始迭代
for i = 1:num_generations
% 计算适应度,即每个个体的完成时间
fitness = zeros(pop_size, 1);
for j = 1:pop_size
fitness(j) = calculate_fitness(pop(j, :), dist_matrix, num_vehicles);
end
% 选择操作,使用轮盘赌选择算法
selected_pop = zeros(pop_size, num_tasks);
for j = 1:pop_size
selected_pop(j, :) = pop(roulette_wheel_selection(fitness), :);
end
% 交叉操作,使用基于顺序的交叉算子
crossover_pop = zeros(pop_size, num_tasks);
for j = 1:2:pop_size
if rand() < crossover_rate
[c1, c2] = sequential_crossover(selected_pop(j, :), selected_pop(j+1, :));
crossover_pop(j, :) = c1;
crossover_pop(j+1, :) = c2;
else
crossover_pop(j, :) = selected_pop(j, :);
crossover_pop(j+1, :) = selected_pop(j+1, :);
end
end
% 变异操作,使用交换变异算子
mutate_pop = zeros(pop_size, num_tasks);
for j = 1:pop_size
if rand() < mutation_rate
mutate_pop(j, :) = swap_mutation(crossover_pop(j, :));
else
mutate_pop(j, :) = crossover_pop(j, :);
end
end
% 新种群
pop = mutate_pop;
end
% 计算每个任务的完成时间
function completion_time = calculate_completion_time(schedule, dist_matrix)
num_vehicles = size(schedule, 1);
num_tasks = size(schedule, 2);
current_time = zeros(num_vehicles, 1);
completion_time = zeros(num_tasks, 1);
for i = 1:num_tasks
min_time = Inf;
min_vehicle = 0;
for j = 1:num_vehicles
if current_time(j) + dist_matrix(schedule(j, i), i) < min_time
min_time = current_time(j) + dist_matrix(schedule(j, i), i);
min_vehicle = j;
end
end
current_time(min_vehicle) = min_time;
completion_time(i) = min_time;
end
end
% 计算适应度,即完成时间
function fitness = calculate_fitness(schedule, dist_matrix, num_vehicles)
completion_time = calculate_completion_time(reshape(schedule, [], num_vehicles)', dist_matrix);
fitness = max(completion_time);
end
% 轮盘赌选择算法
function index = roulette_wheel_selection(fitness)
total_fitness = sum(fitness);
normalized_fitness = fitness / total_fitness;
cumulative_fitness = cumsum(normalized_fitness);
r = rand();
index = find(cumulative_fitness >= r, 1);
end
% 基于顺序的交叉算子
function [child1, child2] = sequential_crossover(parent1, parent2)
num_tasks = length(parent1);
start = randi([1,num_tasks-1]);
stop = randi([start+1,num_tasks]);
child1 = zeros(1, num_tasks);
child2 = zeros(1, num_tasks);
child1(start:stop) = parent1(start:stop);
child2(start:stop) = parent2(start:stop);
index1 = stop + 1;
index2 = stop + 1;
while true
if index1 > num_tasks
index1 = 1;
end
if index2 > num_tasks
index2 = 1;
end
if ~ismember(parent2(index1), child1)
child1(index2) = parent2(index1);
index2 = index2 + 1;
end
if ~ismember(parent1(index2), child2)
child2(index1) = parent1(index2);
index1 = index1 + 1;
end
if isequal(sort(child1), 1:num_tasks) && isequal(sort(child2), 1:num_tasks)
break;
end
end
end
% 交换变异算子
function mutated_schedule = swap_mutation(schedule)
num_tasks = length(schedule);
indices = randperm(num_tasks, 2);
mutated_schedule = schedule;
mutated_schedule(indices(1)) = schedule(indices(2));
mutated_schedule(indices(2)) = schedule(indices(1));
end
```
这个程序使用遗传算法求解车辆调度问题,其中包括了适应度函数、选择操作、交叉操作和变异操作等。具体来说,适应度函数计算每个个体的完成时间;选择操作使用轮盘赌选择算法;交叉操作使用基于顺序的交叉算子;变异操作使用交换变异算子。通过不断迭代,最终得到一组车辆分配方案,使得任务完成时间最短、车辆的使用最优。
matlab车辆调度问题
车辆调度问题是指在一定的约束条件下,将一组车辆分配到不同的任务中,以最优的方式完成任务。在Matlab中,可以使用优化工具箱来解决车辆调度问题。
一种常见的车辆调度问题是旅行商问题(Traveling Salesman Problem,TSP),它要求在给定一组城市和每对城市之间的距离或成本时,找到一个最短的路径,使得每个城市都被访问且只被访问一次。在车辆调度问题中,可以将这个问题扩展为多车辆TSP,即每个车辆需要遍历所有城市一次,并且车辆之间不能重复访问同一个城市。
在Matlab中,可以使用遗传算法、粒子群优化算法等优化算法来求解车辆调度问题。首先,需要定义目标函数,即车辆行驶路径的总长度。然后,使用优化工具箱中的函数(如ga、particleswarm等)来求解最优解。
具体的实现步骤如下:
1. 定义目标函数:计算车辆行驶路径的总长度。
2. 定义约束条件:包括每个城市只能被访问一次、每个车辆需要遍历所有城市一次等约束条件。
3. 调用优化函数:使用优化工具箱中的函数(如ga、particleswarm等)来求解最优解。
4. 分析结果:根据求解的最优解,得到车辆的调度方案。
需要注意的是,车辆调度问题是一个NP-hard问题,因此在实际应用中可能需要考虑问题规模的大小和时间复杂度的限制。对于大规模的问题,可能需要使用启发式算法或近似算法来求解。
相关推荐
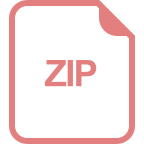
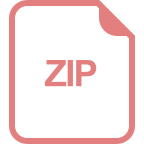












