RuntimeError: Working outside of request context.
时间: 2024-03-13 12:44:30 浏览: 108
这个错误是在 Flask 中使用某些功能时出现的常见错误,通常是因为您在请求上下文之外使用了某些函数或对象。
解决这个问题的方法是确保您的代码在请求上下文之内运行。可以使用 Flask 提供的 `current_app` 和 `g` 对象来确保代码在请求上下文之内运行。
例如,如果您想使用 Flask 中的数据库模块 `flask_sqlalchemy`,您可以在请求处理函数中这样使用:
```
from flask import current_app, g
from flask_sqlalchemy import SQLAlchemy
db = SQLAlchemy()
def my_request_handler():
# make sure we're in a request context
app = current_app._get_current_object()
if not hasattr(g, 'db'):
g.db = db.create_scoped_session()
# use the db object
# ...
```
在这里,我们使用了 Flask 的 `current_app` 对象获取当前应用程序实例,并使用 `g` 对象来存储数据库会话对象。这样可以确保我们的代码在请求上下文之内运行,并且可以安全地使用 Flask 的数据库模块。
相关问题
RuntimeError: Working outside of application context.
This error occurs when you are trying to access or modify data that is tied to the Flask application context, but you are not currently within that context.
For example, if you try to access a Flask extension or request data outside of a Flask route function, you may encounter this error.
To resolve this error, you need to make sure that you are operating within the Flask application context. This can be achieved by wrapping your code within a Flask route function or by using the `with app.app_context()` block.
Here's an example of using `with app.app_context()`:
```python
from flask import Flask
app = Flask(__name__)
with app.app_context():
# your code that requires the Flask application context here
```
Alternatively, you can also use the `current_app` proxy object from the `flask` module to access the Flask application context from within your code:
```python
from flask import current_app
# your code that requires the Flask application context here
current_app.some_extension.do_something()
```
Remember to always make sure you are within the Flask application context before accessing or modifying any data related to your Flask application.
flask raise RuntimeError(unbound_message) from None RuntimeError: Working outside of application context.
这个错误通常发生在 Flask 应用程序上下文之外的代码中尝试访问 Flask 应用程序上下文时。在 Flask 中,应用程序上下文是 Flask 应用程序运行时的环境。为了解决这个问题,你需要在访问 Flask 应用程序上下文之前,先激活应用程序上下文。可以通过将你的代码放在 Flask 应用程序的请求上下文中来实现这一点,或者使用 Flask 提供的上下文管理器,例如 `app.app_context()`。具体实现方式可以参考 Flask 官方文档中的相关章节。
阅读全文
相关推荐
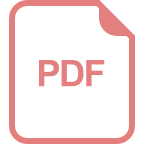
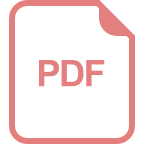

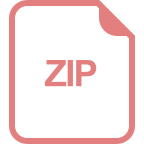
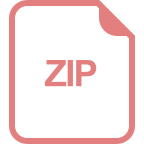
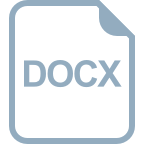
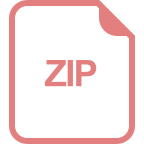
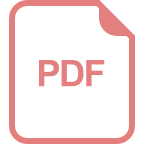
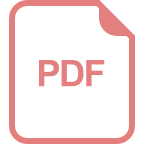
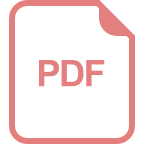
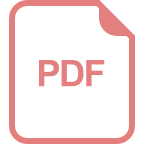
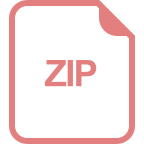
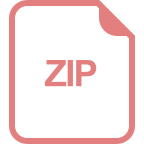
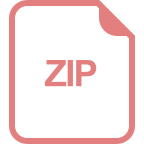