C语言获取uuid对应的rfcomm端口,请提供代码
时间: 2024-03-19 17:44:08 浏览: 81
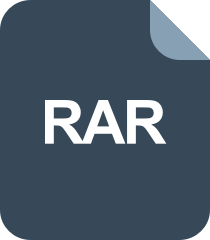
c 代码的UUID生成源码
以下是使用C语言的bluetooth库获取UUID对应的RFCOMM端口的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <bluetooth/bluetooth.h>
#include <bluetooth/sdp.h>
#include <bluetooth/sdp_lib.h>
int main(int argc, char **argv) {
// UUID of the service to search for
uuid_t service_uuid;
sdp_uuid128_create(&service_uuid, 0x12, 0x34, 0x56, 0x78, 0x90, 0xAB, 0xCD, 0xEF, 0xFE, 0xDC, 0xBA, 0x98, 0x76, 0x54, 0x32, 0x10); // Replace with your UUID
// Bluetooth device address to search for
bdaddr_t target_bdaddr = {0, 0, 0, 0, 0, 0}; // Replace with your device address
// Connect to the SDP server on the device
sdp_session_t *session = sdp_connect(BDADDR_ANY, &target_bdaddr, SDP_RETRY_IF_BUSY);
if (!session) {
perror("sdp_connect");
exit(1);
}
// Search for the service with the specified UUID
sdp_list_t *search_list = sdp_list_append(NULL, &service_uuid);
sdp_list_t *response_list = NULL;
uint32_t range = 0x0000ffff;
int err = sdp_service_search_attr_req(session, search_list, SDP_ATTR_REQ_RANGE, &range, &response_list);
if (err) {
perror("sdp_service_search_attr_req");
exit(1);
}
// Get the RFCOMM channel number of the service
uint8_t rfcomm_channel = 0;
sdp_record_t *record = NULL;
for (sdp_list_t *r = response_list; r; r = r->next) {
record = (sdp_record_t *) r->data;
sdp_list_t *proto_list;
if (sdp_get_access_protos(record, &proto_list)) {
continue;
}
for (sdp_list_t *p = proto_list; p; p = p->next) {
sdp_list_t *pds = (sdp_list_t *) p->data;
for (sdp_list_t *d = pds; d; d = d->next) {
sdp_data_t *data = (sdp_data_t *) d->data;
if (data->dtd == SDP_UUID16 || data->dtd == SDP_UUID32 || data->dtd == SDP_UUID128) {
uuid_t uuid;
sdp_uuid16_create(&uuid, data->val.uuid16);
if (sdp_uuid_compare(&uuid, &RFCOMM_UUID) == 0) {
if (sdp_get_rfcomm_channel(record, &rfcomm_channel)) {
perror("sdp_get_rfcomm_channel");
exit(1);
}
}
}
}
sdp_list_free(pds, 0);
}
sdp_list_free(proto_list, 0);
}
// Print the RFCOMM channel number
printf("RFCOMM channel number: %d\n", rfcomm_channel);
// Clean up
sdp_close(session);
sdp_list_free(search_list, 0);
sdp_list_free(response_list, 0);
return 0;
}
```
注意,这段代码仅用于演示目的,实际使用时需要根据自己的需求进行修改和适配。
阅读全文
相关推荐


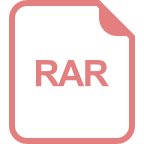
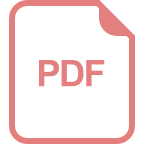
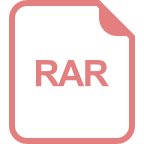
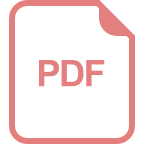
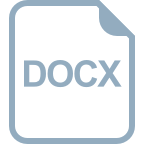
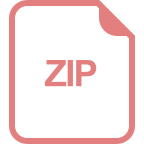
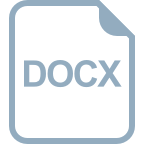
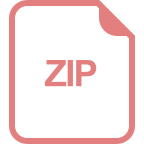
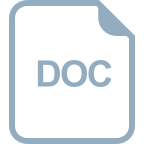

