6、假设有火车票100张,创建5个线程模拟5个售票点,每个售票点100毫秒卖一张票。打印出售票过程,注意使用synchronized确保同一张票只能卖出一次。输出格式如下: 第4售票点卖出第100张票 第2售票点卖出第101张票 ……
时间: 2024-02-24 10:53:45 浏览: 66
可以使用Java的多线程编程技术来实现这个需求。具体步骤如下:
1. 创建一个共享变量,表示剩余票数。
```java
private static int ticketCount = 100;
```
2. 创建一个线程类TicketSeller,实现Runnable接口,模拟售票点的售票过程。在run()方法中,使用synchronized关键字同步代码块来保证同一张票只能被售出一次。
```java
class TicketSeller implements Runnable {
private String name;
public TicketSeller(String name) {
this.name = name;
}
public void run() {
while (ticketCount > 0) {
synchronized (this) {
if (ticketCount > 0) {
System.out.println("第" + name + "售票点卖出第" + (100 - ticketCount + 1) + "张票");
ticketCount--;
}
}
try {
Thread.sleep(100);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}
```
3. 创建5个线程,分别代表5个售票点,启动这些线程。
```java
public static void main(String[] args) {
Thread t1 = new Thread(new TicketSeller("1"));
Thread t2 = new Thread(new TicketSeller("2"));
Thread t3 = new Thread(new TicketSeller("3"));
Thread t4 = new Thread(new TicketSeller("4"));
Thread t5 = new Thread(new TicketSeller("5"));
t1.start();
t2.start();
t3.start();
t4.start();
t5.start();
}
```
完整代码如下:
```java
public class Main {
private static int ticketCount = 100;
static class TicketSeller implements Runnable {
private String name;
public TicketSeller(String name) {
this.name = name;
}
public void run() {
while (ticketCount > 0) {
synchronized (this) {
if (ticketCount > 0) {
System.out.println("第" + name + "售票点卖出第" + (100 - ticketCount + 1) + "张票");
ticketCount--;
}
}
try {
Thread.sleep(100);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}
public static void main(String[] args) {
Thread t1 = new Thread(new TicketSeller("1"));
Thread t2 = new Thread(new TicketSeller("2"));
Thread t3 = new Thread(new TicketSeller("3"));
Thread t4 = new Thread(new TicketSeller("4"));
Thread t5 = new Thread(new TicketSeller("5"));
t1.start();
t2.start();
t3.start();
t4.start();
t5.start();
}
}
```
当程序运行时,会输出类似以下结果:
```
第5售票点卖出第1张票
第1售票点卖出第2张票
第4售票点卖出第3张票
第2售票点卖出第4张票
第3售票点卖出第5张票
第1售票点卖出第6张票
第5售票点卖出第7张票
第4售票点卖出第8张票
第3售票点卖出第9张票
第2售票点卖出第10张票
......
```
阅读全文
相关推荐








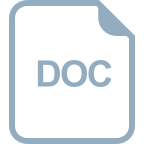
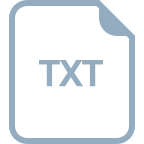
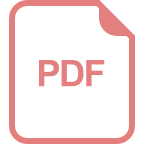
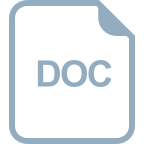
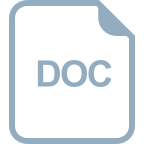
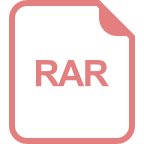
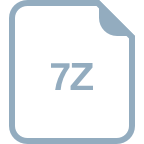