html 鼠标框选表格
时间: 2023-07-01 19:07:20 浏览: 46
可以通过以下步骤来实现在 HTML 表格中使用鼠标框选:
1. 在表格元素(`<table>`)中添加 `onmousedown`、`onmousemove` 和 `onmouseup` 事件处理程序。
```html
<table onmousedown="startSelection(event)" onmousemove="selectCells(event)" onmouseup="endSelection()">
...
</table>
```
2. 编写 JavaScript 函数来处理事件。
```html
<script>
var table = document.getElementById("myTable");
var startRow, startCol, endRow, endCol;
var selecting = false;
function startSelection(event) {
selecting = true;
startRow = endRow = event.target.parentNode.rowIndex;
startCol = endCol = event.target.cellIndex;
}
function selectCells(event) {
if (!selecting) return;
var targetRow = event.target.parentNode.rowIndex;
var targetCol = event.target.cellIndex;
// 更新结束单元格的行列号
endRow = targetRow;
endCol = targetCol;
// 清除之前选中的单元格
clearSelection();
// 选中新的单元格
for (var r = Math.min(startRow, endRow); r <= Math.max(startRow, endRow); r++) {
for (var c = Math.min(startCol, endCol); c <= Math.max(startCol, endCol); c++) {
table.rows[r].cells[c].classList.add("selected");
}
}
}
function endSelection() {
selecting = false;
}
function clearSelection() {
var selectedCells = table.querySelectorAll(".selected");
for (var i = 0; i < selectedCells.length; i++) {
selectedCells[i].classList.remove("selected");
}
}
</script>
```
3. 在 CSS 样式表中定义选中状态的样式。
```css
.selected {
background-color: #ccc;
}
```
完整示例代码如下:
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Mouse selection in table</title>
<style>
table {
border-collapse: collapse;
}
td {
border: 1px solid #ccc;
padding: 5px;
}
.selected {
background-color: #ccc;
}
</style>
</head>
<body>
<table id="myTable" onmousedown="startSelection(event)" onmousemove="selectCells(event)" onmouseup="endSelection()">
<tr>
<td>1</td>
<td>2</td>
<td>3</td>
</tr>
<tr>
<td>4</td>
<td>5</td>
<td>6</td>
</tr>
<tr>
<td>7</td>
<td>8</td>
<td>9</td>
</tr>
</table>
<script>
var table = document.getElementById("myTable");
var startRow, startCol, endRow, endCol;
var selecting = false;
function startSelection(event) {
selecting = true;
startRow = endRow = event.target.parentNode.rowIndex;
startCol = endCol = event.target.cellIndex;
}
function selectCells(event) {
if (!selecting) return;
var targetRow = event.target.parentNode.rowIndex;
var targetCol = event.target.cellIndex;
// 更新结束单元格的行列号
endRow = targetRow;
endCol = targetCol;
// 清除之前选中的单元格
clearSelection();
// 选中新的单元格
for (var r = Math.min(startRow, endRow); r <= Math.max(startRow, endRow); r++) {
for (var c = Math.min(startCol, endCol); c <= Math.max(startCol, endCol); c++) {
table.rows[r].cells[c].classList.add("selected");
}
}
}
function endSelection() {
selecting = false;
}
function clearSelection() {
var selectedCells = table.querySelectorAll(".selected");
for (var i = 0; i < selectedCells.length; i++) {
selectedCells[i].classList.remove("selected");
}
}
</script>
</body>
</html>
```
相关推荐
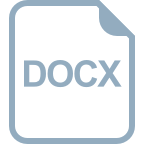
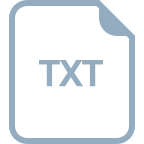
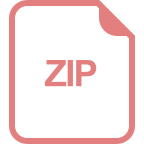











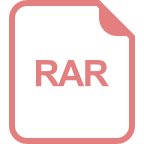
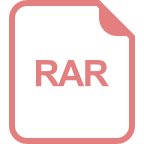
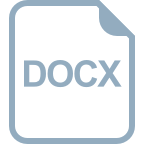
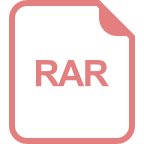