springboot 异步批量上传文件,并添加关键词,统计上传的文件中关键词出现的次数 代码实现
时间: 2024-05-15 10:18:17 浏览: 88
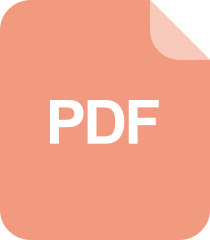
Spring boot 实现单个或批量文件上传功能
好的,关于你的问题,可以使用多线程异步处理文件上传及关键词统计。首先我们需要在SpringBoot中配置对应的异步线程池:
```java
@Configuration
@EnableAsync
public class AsyncConfiguration implements AsyncConfigurer {
@Override
public Executor getAsyncExecutor() {
ThreadPoolTaskExecutor executor = new ThreadPoolTaskExecutor();
executor.setCorePoolSize(10);
executor.setMaxPoolSize(30);
executor.setQueueCapacity(200);
executor.setThreadNamePrefix("async-");
executor.initialize();
return executor;
}
@Override
public AsyncUncaughtExceptionHandler getAsyncUncaughtExceptionHandler() {
return new SimpleAsyncUncaughtExceptionHandler();
}
}
```
接下来,我们可以使用SpringBoot提供的MultipartFile类来处理文件上传:
```java
@Async
public void uploadFile(MultipartFile file) {
// 保存文件到服务器
String filePath = "D:/uploadFile/";
String fileName = file.getOriginalFilename();
File dest = new File(filePath + fileName);
try {
file.transferTo(dest);
} catch (IOException e) {
e.printStackTrace();
}
// 统计文件中关键词出现次数
String[] keywords = {"SpringBoot", "Java", "Python"};
try {
FileInputStream fileInputStream = new FileInputStream(dest);
byte[] bytes = new byte[fileInputStream.available()];
fileInputStream.read(bytes);
String content = new String(bytes);
int[] keywordCount = new int[keywords.length];
for (int i = 0; i < keywords.length; i++) {
String keyword = keywords[i];
int count = StringUtils.countMatches(content, keyword);
keywordCount[i] = count;
}
// TODO: 将文件信息及关键词统计结果保存到数据库中
} catch (IOException e) {
e.printStackTrace();
}
}
```
以上代码示例中,我们首先在uploadFile方法上加上@Async注解,表示该方法为异步方法。在异步方法中,我们先将上传的文件保存到服务器指定的目录中。接着,我们需要统计文件中关键词出现的次数。这里我们定义了一个字符串数组,其中存放了需要统计的关键词。我们使用Java的StringUtils工具类的countMatches方法来统计关键词在文件内容中出现的次数。最后,我们可以将文件信息以及关键词统计结果保存到数据库中。
希望以上代码能对你有所帮助!
阅读全文
相关推荐



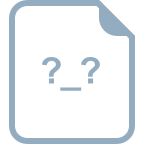

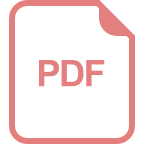
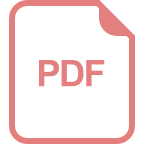
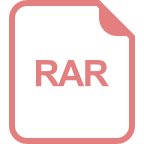
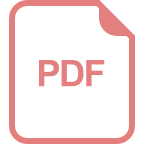
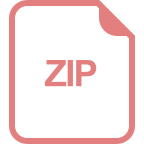
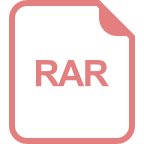
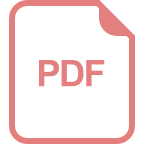
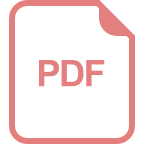
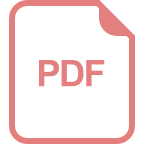
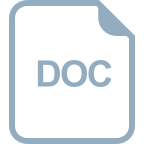
