String xmlData=<?xml version="1.0"?> <Results xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:xsd="http://www.w3.org/2001/XMLSchema"> <Code>0</Code> <Message>返回值内容</Message> <Data xsi:type="BillHeadResponse"> <InvtHead> <EportListNo/> <SeqNo/> </InvtHead> <ListDetail> <InvtList> <BillListFullEntity> <ListGNo>1</ListGNo> <GNo>813</GNo> </BillListFullEntity> <BillListFullEntity> <ListGNo>1</ListGNo> <GNo>813</GNo> </BillListFullEntity> </InvtList> <InvtDtList> <BillListFullEntity> <Unit>054</Unit> <Unit1>035</Unit1> <Unit2>054</Unit2> </BillListFullEntity> </InvtDtList> </ListDetail> <InOutList> <InOutStoreEntity> <SasStockNo>xxxxxxx</SasStockNo> <PoNo></PoNo> </InOutStoreEntity> </InOutList> </Data> </Results> 将xmlData转换成对象
时间: 2024-02-16 11:26:21 浏览: 17
您可以使用Java中的JAXB(Java Architecture for XML Binding)来将XML数据转换为Java对象。首先,您需要创建一个Java类,该类的属性应该与XML数据中的元素名称和属性名称相匹配。例如,在这个例子中,您需要创建一个名为Results的类,包含Code、Message和Data属性,Data属性应该是一个名为BillHeadResponse的类,包含InvtHead、ListDetail和InOutList属性。然后,使用JAXBContext和Unmarshaller来将XML数据转换为Java对象,如下所示:
```java
// 创建JAXBContext对象
JAXBContext jaxbContext = JAXBContext.newInstance(Results.class);
// 创建Unmarshaller对象
Unmarshaller unmarshaller = jaxbContext.createUnmarshaller();
// 将XML数据转换为Java对象
Results results = (Results) unmarshaller.unmarshal(new StringReader(xmlData));
```
在这个例子中,Results是您创建的Java类的名称,xmlData是包含XML数据的字符串。转换后,您可以使用Java对象来访问XML数据中的元素和属性。
相关问题
<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>org.example</groupId> <artifactId>maven_test</artifactId> <version>1.0-SNAPSHOT</version> <dependency> <groupId>org.python</groupId> <artifactId>jython-standalone</artifactId> <version>2.7.0</version> </dependency> </project>
根据您提供的 pom.xml 文件内容,我注意到您的依赖项标签没有被正确地闭合。为了解决这个问题,您需要在每个依赖项的开始和结束标签之间添加一个空格或换行符。以下是您修正后的 pom.xml 文件内容:
```xml
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org.example</groupId>
<artifactId>maven_test</artifactId>
<version>1.0-SNAPSHOT</version>
<dependencies>
<dependency>
<groupId>org.python</groupId>
<artifactId>jython-standalone</artifactId>
<version>2.7.0</version>
</dependency>
</dependencies>
</project>
```
请注意,我添加了一个 `<dependencies>` 标签来包含所有的依赖项。这样,每个依赖项都被包含在 `<dependency>` 标签中,并且正确地闭合。保存并重新运行 Maven 命令,应该就能成功解析和使用这个依赖项了。
vb.net 实现如下请求,应该怎么写? POST /RTService/service.asmx HTTP/1.1 Host: 10.168.1.137 Content-Type: text/xml; charset=utf-8 Content-Length: length SOAPAction: "http://tempuri.org/SpcCheck" <?xml version="1.0" encoding="utf-8"?> <soap:Envelope xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:xsd="http://www.w3.org/2001/XMLSchema" xmlns:soap="http://schemas.xmlsoap.org/soap/envelope/"> <soap:Body> <SpcCheck xmlns="http://tempuri.org/"> <dcs> <DataCollection> <BatchIDs>string</BatchIDs> <WaferIDs>string</WaferIDs></DataCollection> </dcs> </SpcCheck> </soap:Body> </soap:Envelope>
你可以使用VB.Net中的HttpWebRequest和HttpWebResponse类来实现该请求。具体实现过程如下:
```vb.net
Dim request As HttpWebRequest = CType(WebRequest.Create("http://10.168.1.137/RTService/service.asmx"), HttpWebRequest)
request.Method = "POST"
request.ContentType = "text/xml; charset=utf-8"
request.Headers.Add("SOAPAction", "http://tempuri.org/SpcCheck")
Dim xml As String = "<?xml version=""1.0"" encoding=""utf-8""?>" & _
"<soap:Envelope xmlns:xsi=""http://www.w3.org/2001/XMLSchema-instance"" " & _
"xmlns:xsd=""http://www.w3.org/2001/XMLSchema"" " & _
"xmlns:soap=""http://schemas.xmlsoap.org/soap/envelope/"">" & _
"<soap:Body>" & _
"<SpcCheck xmlns=""http://tempuri.org/"">" & _
"<dcs>" & _
"<DataCollection>" & _
"<BatchIDs>string</BatchIDs>" & _
"<WaferIDs>string</WaferIDs>" & _
"</DataCollection>" & _
"</dcs>" & _
"</SpcCheck>" & _
"</soap:Body>" & _
"</soap:Envelope>"
Dim data As Byte() = Encoding.UTF8.GetBytes(xml)
request.ContentLength = data.Length
Using stream As Stream = request.GetRequestStream()
stream.Write(data, 0, data.Length)
End Using
Dim response As HttpWebResponse = CType(request.GetResponse(), HttpWebResponse)
Dim reader As StreamReader = New StreamReader(response.GetResponseStream())
Dim result As String = reader.ReadToEnd()
Console.WriteLine(result)
```
这段代码发送了一个POST请求,将请求体设置为xml变量中的内容,然后从响应流中读取响应并输出。你需要将`10.168.1.137`替换为实际的IP地址,以及将`string`替换为实际的数据。
相关推荐
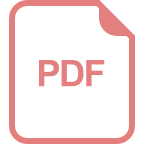
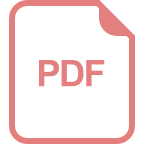












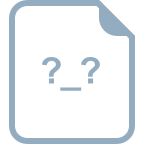