我需要用react+ts写一个递归调用,递归到没有这个对象属性后跳到上一层返回所有id为1的对象数组
时间: 2024-03-02 11:53:18 浏览: 22
好的,您可以参考以下代码实现一个递归函数,该函数可以递归遍历一个对象的属性,当遍历到没有某个属性时,返回包含所有 id 为 1 的对象的数组。这里假设您的数据结构为:
```typescript
interface Node {
id: number;
children?: Node[];
}
```
代码如下:
```typescript
function getAllNodesWithIdOne(node: Node, result: Node[] = []): Node[] {
if (node?.id === 1) {
result.push(node);
}
if (!node.children || !node.children.length) {
return result;
}
for (const child of node.children) {
getAllNodesWithIdOne(child, result);
}
return result;
}
```
在这个函数中,我们首先判断当前节点的 id 是否为 1,如果是,则将该节点加入结果数组中。然后判断该节点是否有子节点,如果没有,则返回结果数组;否则,对每个子节点递归调用该函数。最终返回结果数组。
您可以将该函数作为一个组件的方法,在组件中使用。例如:
```typescript
interface MyComponentProps {
node: Node;
}
interface MyComponentState {
nodesWithIdOne: Node[];
}
class MyComponent extends React.Component<MyComponentProps, MyComponentState> {
constructor(props: MyComponentProps) {
super(props);
this.state = {
nodesWithIdOne: [],
};
}
componentDidMount() {
const { node } = this.props;
const nodesWithIdOne = getAllNodesWithIdOne(node);
this.setState({ nodesWithIdOne });
}
render() {
const { nodesWithIdOne } = this.state;
return (
<div>
<h2>Nodes with id=1:</h2>
<ul>
{nodesWithIdOne.map((node) => (
<li key={node.id}>{node.id}</li>
))}
</ul>
</div>
);
}
}
```
在该组件的 `componentDidMount` 生命周期方法中调用 `getAllNodesWithIdOne` 函数获取所有 id 为 1 的节点,然后将结果保存在组件的状态中。在组件的 `render` 方法中,我们将结果渲染为一个无序列表。
相关推荐
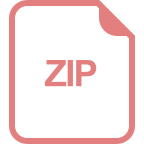
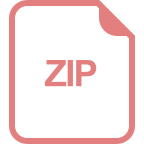














