如何通过c++编程语言实现一个超市销售管理系统
时间: 2023-11-03 18:04:41 浏览: 40
实现一个超市销售管理系统需要以下几个步骤:
1. 定义数据结构:定义商品、订单、顾客等数据结构,包括它们之间的关系。
2. 实现基本功能:定义添加商品、查询商品、添加顾客、查询顾客、创建订单、查询订单等基本功能。
3. 实现交互界面:使用图形界面或者命令行界面与用户进行交互,提供用户友好的操作界面。
4. 数据存储:实现将数据存储到文件中,以便下次启动程序时可以恢复数据。
下面是一个简单的C++代码示例:
```c++
#include <iostream>
#include <fstream>
#include <string>
#include <vector>
using namespace std;
struct Product {
int id;
string name;
double price;
int stock;
};
struct Order {
int id;
int customer_id;
vector<Product> products;
};
struct Customer {
int id;
string name;
string phone;
};
vector<Product> products;
vector<Customer> customers;
vector<Order> orders;
void add_product() {
Product product;
cout << "Enter product id: ";
cin >> product.id;
cout << "Enter product name: ";
cin >> product.name;
cout << "Enter product price: ";
cin >> product.price;
cout << "Enter product stock: ";
cin >> product.stock;
products.push_back(product);
}
void list_products() {
for (auto product : products) {
cout << "Product id: " << product.id << endl;
cout << "Product name: " << product.name << endl;
cout << "Product price: " << product.price << endl;
cout << "Product stock: " << product.stock << endl;
}
}
void add_customer() {
Customer customer;
cout << "Enter customer id: ";
cin >> customer.id;
cout << "Enter customer name: ";
cin >> customer.name;
cout << "Enter customer phone: ";
cin >> customer.phone;
customers.push_back(customer);
}
void list_customers() {
for (auto customer : customers) {
cout << "Customer id: " << customer.id << endl;
cout << "Customer name: " << customer.name << endl;
cout << "Customer phone: " << customer.phone << endl;
}
}
void create_order() {
Order order;
cout << "Enter order id: ";
cin >> order.id;
cout << "Enter customer id: ";
cin >> order.customer_id;
while (true) {
int product_id;
cout << "Enter product id (0 to finish): ";
cin >> product_id;
if (product_id == 0) {
break;
}
for (auto product : products) {
if (product.id == product_id) {
order.products.push_back(product);
break;
}
}
}
orders.push_back(order);
}
void list_orders() {
for (auto order : orders) {
cout << "Order id: " << order.id << endl;
cout << "Customer id: " << order.customer_id << endl;
for (auto product : order.products) {
cout << "Product id: " << product.id << endl;
cout << "Product name: " << product.name << endl;
cout << "Product price: " << product.price << endl;
cout << "Product stock: " << product.stock << endl;
}
}
}
void save_data() {
ofstream file("data.txt");
file << products.size() << endl;
for (auto product : products) {
file << product.id << " " << product.name << " " << product.price << " " << product.stock << endl;
}
file << customers.size() << endl;
for (auto customer : customers) {
file << customer.id << " " << customer.name << " " << customer.phone << endl;
}
file << orders.size() << endl;
for (auto order : orders) {
file << order.id << " " << order.customer_id << " " << order.products.size() << endl;
for (auto product : order.products) {
file << product.id << " " << product.name << " " << product.price << " " << product.stock << endl;
}
}
file.close();
}
void load_data() {
ifstream file("data.txt");
int num_products;
file >> num_products;
for (int i = 0; i < num_products; i++) {
Product product;
file >> product.id >> product.name >> product.price >> product.stock;
products.push_back(product);
}
int num_customers;
file >> num_customers;
for (int i = 0; i < num_customers; i++) {
Customer customer;
file >> customer.id >> customer.name >> customer.phone;
customers.push_back(customer);
}
int num_orders;
file >> num_orders;
for (int i = 0; i < num_orders; i++) {
Order order;
file >> order.id >> order.customer_id;
int num_products;
file >> num_products;
for (int j = 0; j < num_products; j++) {
Product product;
file >> product.id >> product.name >> product.price >> product.stock;
order.products.push_back(product);
}
orders.push_back(order);
}
file.close();
}
int main() {
load_data();
while (true) {
cout << "1. Add product" << endl;
cout << "2. List products" << endl;
cout << "3. Add customer" << endl;
cout << "4. List customers" << endl;
cout << "5. Create order" << endl;
cout << "6. List orders" << endl;
cout << "7. Save data" << endl;
cout << "8. Exit" << endl;
int choice;
cin >> choice;
switch (choice) {
case 1:
add_product();
break;
case 2:
list_products();
break;
case 3:
add_customer();
break;
case 4:
list_customers();
break;
case 5:
create_order();
break;
case 6:
list_orders();
break;
case 7:
save_data();
break;
case 8:
return 0;
default:
cout << "Invalid choice" << endl;
break;
}
}
}
```
注意,这只是一个简单的示例代码,实际的超市销售管理系统需要更加完善和复杂的功能。
相关推荐
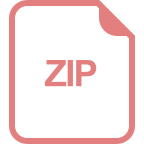











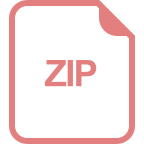