cellvaluechanged c#
时间: 2023-07-30 07:10:56 浏览: 206
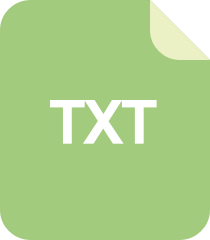
C# 修改注册表值
"CellValueChanged" 是 C# 中 DataGridView 控件事件之一。当 DataGridView 控件中单元格的值被更改时,该事件将被触发。您可以使用该事件来响应用户更改单元格值的行为,例如更新相关数据或执行其他操作。以下是 "CellValueChanged" 事件的基本用法示例:
```csharp
private void dataGridView1_CellValueChanged(object sender, DataGridViewCellEventArgs e)
{
// 获取更改的单元格的行和列索引
int rowIndex = e.RowIndex;
int columnIndex = e.ColumnIndex;
// 获取更改后的单元格值
string newValue = dataGridView1.Rows[rowIndex].Cells[columnIndex].Value.ToString();
// 执行其他操作,例如更新相关数据
}
```
阅读全文
相关推荐
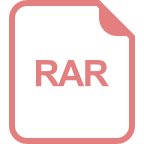
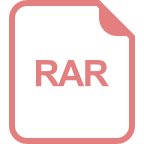
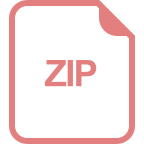
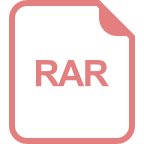
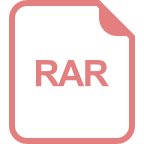
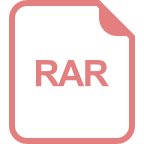
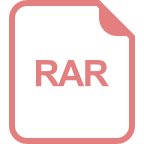
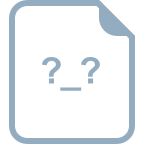
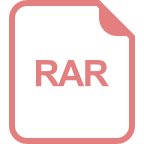
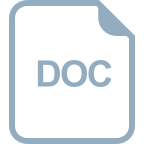
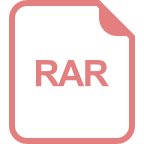
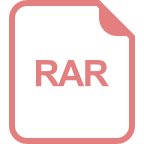
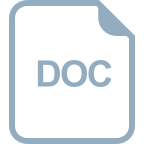
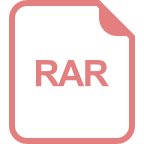


