esp32作为客户端基于ESPIDF通过tcp协议传输二维整型数组
时间: 2023-05-18 08:06:08 浏览: 58
可以使用以下代码实现:
```c
#include <stdio.h>
#include <string.h>
#include <sys/socket.h>
#include <netdb.h>
#include <esp_wifi.h>
#include <esp_event_loop.h>
#include <esp_log.h>
#include <esp_system.h>
#include <nvs_flash.h>
#define HOST_IP_ADDR "192.168.1.100"
#define PORT 8080
static const char *TAG = "TCP_CLIENT";
void tcp_client_task(void *pvParameters)
{
int addr_family = 0;
int ip_protocol = 0;
struct sockaddr_in dest_addr;
dest_addr.sin_addr.s_addr = inet_addr(HOST_IP_ADDR);
dest_addr.sin_family = AF_INET;
dest_addr.sin_port = htons(PORT);
addr_family = AF_INET;
ip_protocol = IPPROTO_IP;
int sock = socket(addr_family, SOCK_STREAM, ip_protocol);
if (sock < 0) {
ESP_LOGE(TAG, "Unable to create socket: errno %d", errno);
vTaskDelete(NULL);
return;
}
ESP_LOGI(TAG, "Socket created, connecting to %s:%d", HOST_IP_ADDR, PORT);
int err = connect(sock, (struct sockaddr *)&dest_addr, sizeof(dest_addr));
if (err != 0) {
ESP_LOGE(TAG, "Socket unable to connect: errno %d", errno);
vTaskDelete(NULL);
return;
}
ESP_LOGI(TAG, "Successfully connected");
int array[2][2] = {{1, 2}, {3, 4}};
char buffer[4 * sizeof(int)];
memcpy(buffer, array, sizeof(array));
int err_send = send(sock, buffer, sizeof(buffer), 0);
if (err_send < 0) {
ESP_LOGE(TAG, "Error occurred during sending: errno %d", errno);
}
ESP_LOGI(TAG, "Message sent");
vTaskDelete(NULL);
}
void app_main()
{
nvs_flash_init();
tcpip_adapter_init();
ESP_ERROR_CHECK(esp_event_loop_create_default());
wifi_init_config_t cfg = WIFI_INIT_CONFIG_DEFAULT();
ESP_ERROR_CHECK(esp_wifi_init(&cfg));
ESP_ERROR_CHECK(esp_wifi_set_storage(WIFI_STORAGE_RAM));
ESP_ERROR_CHECK(esp_wifi_set_mode(WIFI_MODE_STA));
wifi_config_t wifi_config = {
.sta = {
.ssid = "your_wifi_ssid",
.password = "your_wifi_password",
},
};
ESP_ERROR_CHECK(esp_wifi_set_config(ESP_IF_WIFI_STA, &wifi_config));
ESP_ERROR_CHECK(esp_wifi_start());
ESP_ERROR_CHECK(esp_wifi_connect());
xTaskCreate(tcp_client_task, "tcp_client", 4096, NULL, 5, NULL);
}
```
这段代码实现了一个 ESP32 客户端,通过 TCP 协议向指定 IP 地址和端口号的服务器发送一个二维整型数组。在这个例子中,数组的值为 {{1, 2}, {3, 4}},可以根据实际需求修改。
相关推荐
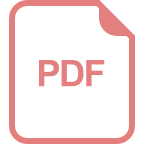














