python tkinter listbox
时间: 2023-04-22 07:03:48 浏览: 190
Tkinter 是 Python 标准库中的一个图形用户界面 (GUI) 工具包,Listbox 是 Tkinter 中的一个组件,可用于在 GUI 中显示列表。它提供了一个可滚动的列表框,可用于显示项目列表。
Listbox 组件可用于创建一个列表框,在其中可以显示项目列表。可以使用 insert() 方法将项目插入列表中,使用 delete() 方法删除项目,使用 get() 方法获取列表中的项目。
示例代码:
```
from tkinter import *
root = Tk()
listbox = Listbox(root)
listbox.pack()
listbox.insert(END, "item1")
listbox.insert(END, "item2")
root.mainloop()
```
通过这段简单的代码可以展示一个窗口,里面有两个列表项 "item1" "item2"
相关问题
python tkinter Listbox
Python的Tkinter库是一个用于创建图形用户界面(GUI)的标准库。其中的Listbox是Tkinter提供的一个控件,用于显示一个可选项列表。
Listbox控件可以用于显示一列选项,并允许用户从中选择一个或多个选项。它可以用于展示一组数据、菜单选项、文件列表等。
在Tkinter中创建一个Listbox控件的步骤如下:
1. 导入Tkinter库:`import tkinter as tk`
2. 创建主窗口:`root = tk.Tk()`
3. 创建Listbox控件:`listbox = tk.Listbox(root)`
4. 添加选项到Listbox:`listbox.insert(tk.END, "Option 1")`
5. 显示Listbox:`listbox.pack()`
6. 运行主循环:`root.mainloop()`
可以通过调用Listbox的方法来操作和管理Listbox控件,例如:
- `listbox.insert(index, item)`:在指定位置插入一个选项。
- `listbox.delete(first, last=None)`:删除指定范围内的选项。
- `listbox.get(index)`:获取指定位置的选项。
- `listbox.curselection()`:获取当前选中的选项的索引。
- `listbox.selection_set(first, last=None)`:设置选中的选项。
- `listbox.selection_clear(first, last=None)`:取消选中的选项。
注意,Listbox控件默认只能单选,如果需要多选,可以设置`selectmode`属性为`tk.MULTIPLE`。
Python tkinter Listbox
The Listbox widget in tkinter is used to display a list of items. It allows the user to select one or more items from the list. The following code creates a Listbox widget and adds items to it:
```
import tkinter as tk
root = tk.Tk()
# Create a Listbox widget
listbox = tk.Listbox(root)
# Add items to the Listbox
listbox.insert(0, "Item 1")
listbox.insert(1, "Item 2")
listbox.insert(2, "Item 3")
# Pack the Listbox widget
listbox.pack()
root.mainloop()
```
In the above code, we first import the tkinter module and create a new instance of the Tk class. We then create a Listbox widget and add items to it using the `insert` method. Finally, we pack the Listbox widget using the `pack` method and start the main event loop using the `mainloop` method of the root window.
We can also bind a function to the Listbox widget to be executed when an item is selected. The following code demonstrates this:
```
import tkinter as tk
root = tk.Tk()
# Define a function to be called when an item is selected
def on_select(event):
selected_item = event.widget.get(event.widget.curselection())
print("Selected item:", selected_item)
# Create a Listbox widget
listbox = tk.Listbox(root)
# Add items to the Listbox
listbox.insert(0, "Item 1")
listbox.insert(1, "Item 2")
listbox.insert(2, "Item 3")
# Bind the on_select function to the Listbox widget
listbox.bind('<<ListboxSelect>>', on_select)
# Pack the Listbox widget
listbox.pack()
root.mainloop()
```
In the above code, we define a function `on_select` that is called when an item is selected. This function retrieves the selected item using the `curselection` method of the Listbox widget and prints it to the console. We then bind this function to the Listbox widget using the `bind` method and the `<<ListboxSelect>>` event. When an item is selected, the `on_select` function is called and the selected item is printed to the console.
阅读全文
相关推荐





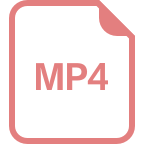







