如何用adt设计xml序列化
时间: 2024-10-24 11:05:48 浏览: 8
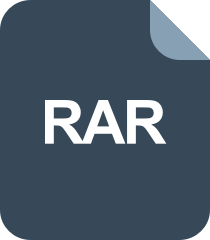
android 二进制传输 序列化

在Eclipse ADT (Android Development Tools) 中,设计XML序列化通常涉及到将数据结构映射到XML格式以便于存储和交互。Android支持通过`Serializable`、`Parcelable`接口以及`Gson`库等来进行对象到XML的转换。这里我们以`Serializable`为例简单讲解:
1. **创建Serializable类**:
首先,你需要创建一个实现了`Serializable`接口的类,这个类就是你要序列化的对象。比如有一个简单的用户模型(User):
```java
public class User implements Serializable {
private String name;
private int age;
// getters and setters...
}
```
2. **编写XML布局文件**:
创建一个XML布局文件,如`User.xml`,用于描述对象的数据结构:
```xml
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content">
<TextView
android:id="@+id/user_name"
android:layout_width="wrap_content"
android:layout_height="wrap_content" />
<TextView
android:id="@+id/user_age"
android:layout_width="wrap_content"
android:layout_height="wrap_content" />
</LinearLayout>
```
3. **序列化和反序列化**:
当需要保存User对象到XML时,你可以将其转换成`OutputStream`然后写入文件:
```java
User user = new User("张三", 25);
// 序列化
ObjectOutputStream out = new ObjectOutputStream(new FileOutputStream("users.xml"));
out.writeObject(user);
out.close();
```
要从XML加载回User对象,使用`ObjectInputStream`读取并实例化:
```java
ObjectInputStream in = new ObjectInputStream(new FileInputStream("users.xml"));
User restoredUser = (User) in.readObject();
in.close();
```
注意,这种方式虽然简单,但在实际应用中可能会遇到性能问题,因为`Serializable`默认不是线程安全的。如果需要处理大量数据或者分布式环境,推荐使用`Parcelables`或者第三方库如Gson。
阅读全文
相关推荐
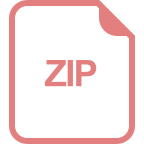
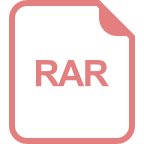
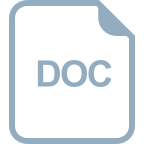
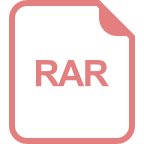
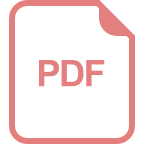
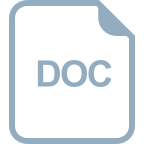
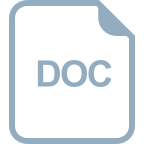
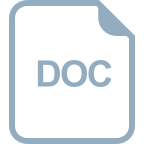
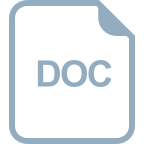
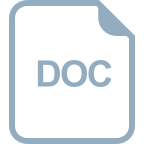

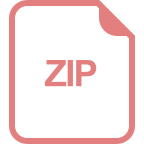
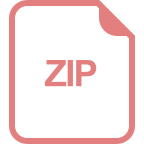