A uart read/write example without event queue,这个event queue 具体代码什么
时间: 2024-03-03 17:48:19 浏览: 105
Event queue是一种在嵌入式系统中常用的机制,用于处理不同任务之间的通信和协调。在使用UART进行读写时,可以通过event queue机制来处理UART的读写事件,从而实现异步读写。下面是一个不使用event queue的UART读写示例代码:
```c
#include "stdio.h"
#include "stdlib.h"
#include "string.h"
#include "unistd.h"
#include "fcntl.h"
#include "termios.h"
#define BAUDRATE B115200
#define SERIAL_DEVICE "/dev/ttyS1"
int main(int argc, char** argv)
{
int fd;
struct termios options;
fd = open(SERIAL_DEVICE, O_RDWR | O_NOCTTY | O_NDELAY);
if (fd < 0)
{
printf("Failed to open serial port!\n");
return -1;
}
tcgetattr(fd, &options);
cfsetispeed(&options, BAUDRATE);
cfsetospeed(&options, BAUDRATE);
options.c_cflag |= (CLOCAL | CREAD);
options.c_cflag &= ~PARENB;
options.c_cflag &= ~CSTOPB;
options.c_cflag &= ~CSIZE;
options.c_cflag |= CS8;
tcsetattr(fd, TCSANOW, &options);
char buf[255];
int len = read(fd, buf, sizeof(buf));
write(fd, buf, len);
close(fd);
return 0;
}
```
在上面的代码中,我们使用open函数打开串口设备文件,然后使用tcgetattr和tcsetattr函数设置串口的属性。接下来,我们使用read函数读取串口数据,然后使用write函数将读取到的数据写回串口。这些操作都是同步的,没有采用event queue机制。
如果要使用event queue机制来处理UART读写事件,需要在代码中添加一些线程或任务,用于异步读写数据,并通过event queue机制来进行通信和协调。具体的代码实现取决于不同的嵌入式系统和应用场景。
阅读全文
相关推荐
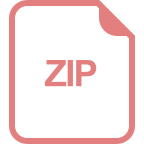
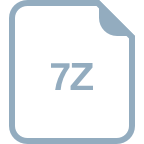
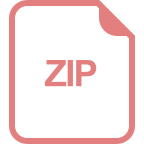
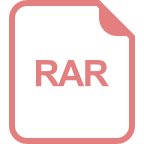
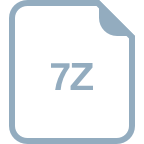
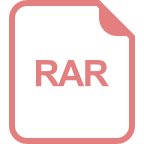
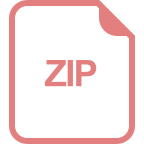
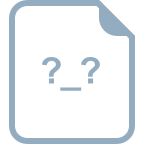
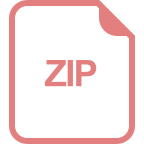
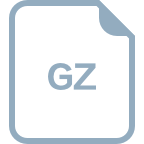
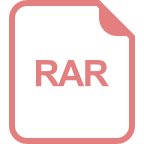
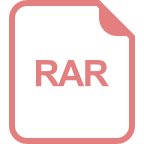
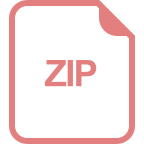
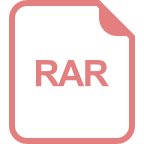
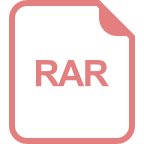
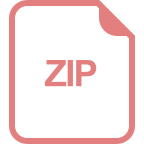
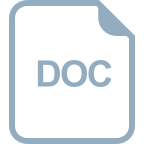
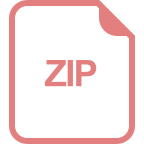