用c语言写一个表达式求值的程序
时间: 2023-08-31 07:26:25 浏览: 123
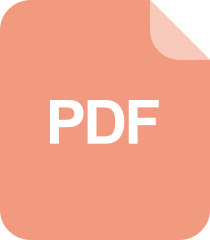
数据结构课程设计-用栈实现表达式求值的方法详解
### 回答1:
好的,我可以回答这个问题。以下是一个用 C 语言编写的表达式求值程序的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#define STACK_SIZE 100
typedef struct {
int top;
int data[STACK_SIZE];
} Stack;
void push(Stack *stack, int value) {
if (stack->top == STACK_SIZE - 1) {
fprintf(stderr, "Stack overflow\n");
exit(1);
}
stack->data[++stack->top] = value;
}
int pop(Stack *stack) {
if (stack->top == -1) {
fprintf(stderr, "Stack underflow\n");
exit(1);
}
return stack->data[stack->top--];
}
int evaluate(char *expr) {
Stack stack = { .top = -1 };
int i, op1, op2;
char ch;
for (i = ; expr[i] != '\'; i++) {
ch = expr[i];
if (ch >= '' && ch <= '9') {
push(&stack, ch - '');
} else if (ch == '+' || ch == '-' || ch == '*' || ch == '/') {
op2 = pop(&stack);
op1 = pop(&stack);
switch (ch) {
case '+': push(&stack, op1 + op2); break;
case '-': push(&stack, op1 - op2); break;
case '*': push(&stack, op1 * op2); break;
case '/': push(&stack, op1 / op2); break;
}
}
}
return pop(&stack);
}
int main() {
char expr[] = "23+4*";
int result = evaluate(expr);
printf("%d\n", result);
return ;
}
```
这个程序可以计算后缀表达式的值,例如上面的表达式 "23+4*" 的值为 10。
### 回答2:
用C语言写一个表达式求值的程序可以借助栈来实现。下面是一种基本的实现方式:
首先,我们需要包含相关的头文件。如下所示:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_SIZE 100
typedef struct {
int data[MAX_SIZE];
int top;
} Stack;
void init_stack(Stack* s) {
s->top = -1;
}
int is_empty(Stack* s) {
return s->top == -1;
}
void push(Stack* s, int item) {
if (s->top >= MAX_SIZE - 1) {
printf("Stack overflow!\n");
return;
}
s->data[++s->top] = item;
}
int pop(Stack* s) {
if (is_empty(s)) {
printf("Stack is empty!\n");
exit(1);
}
return s->data[s->top--];
}
int get_top(Stack* s) {
if (is_empty(s)) {
printf("Stack is empty!\n");
exit(1);
}
return s->data[s->top];
}
int calculate(int operand1, int operand2, char operator) {
switch (operator) {
case '+':
return operand1 + operand2;
case '-':
return operand1 - operand2;
case '*':
return operand1 * operand2;
case '/':
return operand1 / operand2;
default:
printf("Invalid operator!\n");
exit(1);
}
}
int evaluate_expression(char* expression) {
Stack operand_stack;
Stack operator_stack;
init_stack(&operand_stack);
init_stack(&operator_stack);
int i = 0;
while (expression[i] != '\0') {
if (expression[i] >= '0' && expression[i] <= '9') {
int operand = 0;
while (expression[i] >= '0' && expression[i] <= '9') {
operand = operand * 10 + (expression[i] - '0');
i++;
}
push(&operand_stack, operand);
} else if (expression[i] == '+' || expression[i] == '-' || expression[i] == '*' || expression[i] == '/') {
while (!is_empty(&operator_stack) && get_top(&operator_stack) != '(' && ((expression[i] != '*' && expression[i] != '/') || (get_top(&operator_stack) != '+' && get_top(&operator_stack) != '-'))) {
int operand2 = pop(&operand_stack);
int operand1 = pop(&operand_stack);
char operator = pop(&operator_stack);
int result = calculate(operand1, operand2, operator);
push(&operand_stack, result);
}
push(&operator_stack, expression[i]);
i++;
} else if (expression[i] == '(') {
push(&operator_stack, expression[i]);
i++;
} else if (expression[i] == ')') {
while (!is_empty(&operator_stack) && get_top(&operator_stack) != '(') {
int operand2 = pop(&operand_stack);
int operand1 = pop(&operand_stack);
char operator = pop(&operator_stack);
int result = calculate(operand1, operand2, operator);
push(&operand_stack, result);
}
if (!is_empty(&operator_stack) && get_top(&operator_stack) == '(') {
pop(&operator_stack); // 弹出左括号
} else {
printf("Invalid expression!\n");
exit(1);
}
i++;
} else {
printf("Invalid expression!\n");
exit(1);
}
}
while (!is_empty(&operator_stack)) {
int operand2 = pop(&operand_stack);
int operand1 = pop(&operand_stack);
char operator = pop(&operator_stack);
int result = calculate(operand1, operand2, operator);
push(&operand_stack, result);
}
return pop(&operand_stack);
}
int main() {
char expression[100];
printf("请输入表达式:");
scanf("%s", expression);
int result = evaluate_expression(expression);
printf("结果为:%d\n", result);
return 0;
}
```
以上是一个简单的表达式求值程序的实现。通过输入一个中缀表达式,程序会自动计算并给出结果。同时,该程序还可以处理括号、加减乘除运算等情况,并能够处理多位数的操作数。
### 回答3:
使用C语言编写一个表达式求值的程序可以通过递归和栈来实现。
首先,定义一个结构体,用来表示表达式中的操作数和运算符:
```c
struct Node {
int value; // 操作数或运算结果的值
char operator; // 运算符
struct Node *left; // 左子节点
struct Node *right; // 右子节点
};
```
然后,创建一个栈结构用来存储表达式中的操作数和运算符:
```c
struct Stack {
struct Node* data[100];
int top;
};
```
接下来,定义一系列函数来实现表达式求值的操作,包括:
1. 创建一个新的节点:
```c
struct Node* newNode(int value, char operator);
```
2. 检查一个字符是否为运算符:
```c
int isOperator(char c);
```
3. 将一个字符转换为整数:
```c
int charToInt(char c);
```
4. 将中缀表达式转换为逆波兰表达式:
```c
int infixToPostfix(char *infix, char *postfix);
```
5. 根据逆波兰表达式构建表达式树:
```c
struct Node* buildExpressionTree(char *postfix, int length);
```
6. 计算表达式树的值:
```c
int evaluateExpressionTree(struct Node* root);
```
最后,在主函数中,使用以上函数来完成表达式求值的过程:
```c
int main() {
char infix[100], postfix[100];
printf("请输入中缀表达式:");
scanf("%s", infix);
int length = infixToPostfix(infix, postfix);
struct Node* root = buildExpressionTree(postfix, length);
int result = evaluateExpressionTree(root);
printf("结果为:%d\n", result);
return 0;
}
```
以上就是用C语言实现一个表达式求值的简单示例,可以根据实际需求进行进一步的扩展和优化。
阅读全文
相关推荐
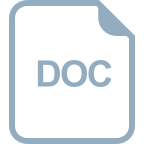
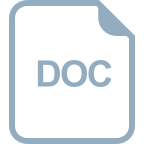
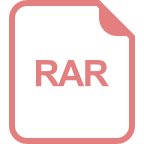

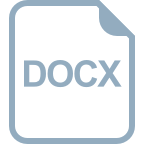
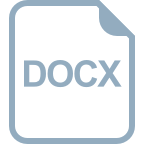
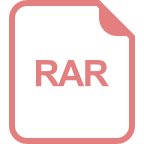
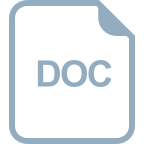
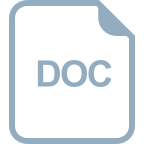
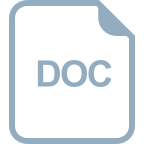
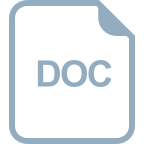
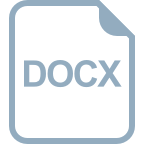
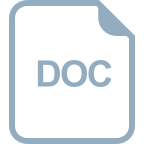
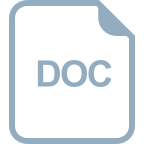
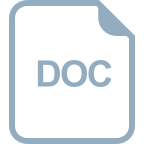