concurrenthashmap putifabsent导致堆栈溢出
时间: 2023-09-15 07:22:52 浏览: 54
可能会有多种原因导致ConcurrentHashMap的putIfAbsent方法导致堆栈溢出,以下是一些可能的原因:
1. 递归调用:如果在putIfAbsent方法中递归调用了自身,就会导致无限递归,最终导致堆栈溢出。
2. 内存泄漏:如果ConcurrentHashMap中已经存在大量的键值对,调用putIfAbsent方法时会导致内存不断增长,最终导致堆栈溢出。
3. 并发问题:ConcurrentHashMap是线程安全的,但是如果多个线程同时调用putIfAbsent方法,可能会导致死锁或者其他并发问题,从而导致堆栈溢出。
针对这些可能的原因,可以通过以下措施来解决问题:
1. 检查代码,确保没有递归调用putIfAbsent方法的情况。
2. 定期清理ConcurrentHashMap中的键值对,避免内存泄漏。
3. 使用合适的并发控制机制,避免多个线程同时调用putIfAbsent方法。
相关问题
ConcurrentHashMap putIfAbsent
ConcurrentHashMap's `putIfAbsent(key, value)` is used to atomically associate the specified key with the given value if it is not already associated with a value in the map. It returns the previous value associated with the key, or `null` if there was no mapping for the key.
Here's an example of how to use `putIfAbsent()`:
```java
ConcurrentHashMap<String, Integer> map = new ConcurrentHashMap<>();
map.put("key1", 1);
// putIfAbsent will only add the mapping if there's no existing mapping for the key
Integer previousValue = map.putIfAbsent("key1", 2);
System.out.println(previousValue); // Output: 1
previousValue = map.putIfAbsent("key2", 2);
System.out.println(previousValue); // Output: null
```
In the first `putIfAbsent()` call, since "key1" already has a value of 1 associated with it, the method does not modify the map and returns the previous value (1).
In the second `putIfAbsent()` call, "key2" has no existing mapping, so the method adds a new mapping with the specified value of 2 and returns `null`.
The `putIfAbsent()` operation is atomic, meaning that multiple threads can safely invoke this method concurrently without running into issues like race conditions.
ConcurrentHashMap的putIfAbsent
ConcurrentHashMap是Java中线程安全的哈希表实现,putIfAbsent是ConcurrentHashMap提供的一个原子操作方法,用于向哈希表中添加键值对,如果该键已经存在,则返回已存在的值,不会进行覆盖操作。如果该键不存在,则添加该键值对并返回null。下面是一个示例代码:
```java
ConcurrentHashMap<String, String> map = new ConcurrentHashMap<>();map.put("key", "value1");
String oldValue = map.putIfAbsent("key", "value2");
System.out.println(oldValue); // 输出:value1
String newValue = map.putIfAbsent("newKey", "value3");
System.out.println(newValue); // 输出:null
```
上述代码中,首先向ConcurrentHashMap中添加了一个键为"key",值为"value1"的键值对。然后使用putIfAbsent方法向ConcurrentHashMap中添加了两个键值对,第一个键为"key",值为"value2",由于该键已经存在,putIfAbsent方法返回了已存在的值"value1";第二个键为"newKey",值为"value3",由于该键不存在,putIfAbsent方法添加了该键值对并返回了null。
相关推荐
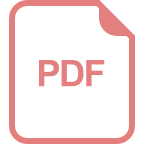
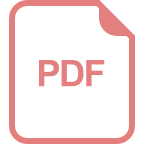
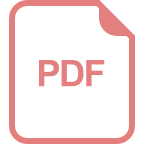








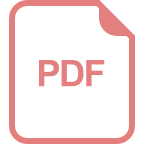
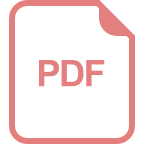
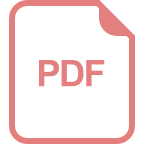
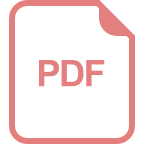
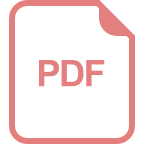
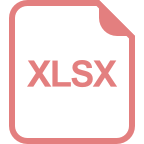