将上面代码用C#语言实现
时间: 2024-12-22 15:29:59 浏览: 8
在C#中,你可以使用`System.Collections.Generic.PriorityQueue<T>`来创建优先队列,并通过提供一个`IComparer<T>`或`Comparator<T>`实现来定制比较规则。以下是将上述C++代码转换为C#的基本版本:
```csharp
using System;
using System.Collections.Generic;
class Program
{
public class Node
{
// 假设Node有一个名为f的属性
public int f { get; set; }
// 其他必要的属性和构造方法...
}
public static bool CompareNodes(Node a, Node b)
{
return a.f > b.f; // 这里同样比较的是f值
}
private static void Main()
{
List<Node> open_list = new List<Node>(); // 或使用PriorityQueue如果你需要堆结构
// 初始化open_list...
// 对于优先队列,你可以这样做:
var priorityQueue = new PriorityQueue<Node>(CompareNodes);
// 或者,如果你想基于List:
priorityQueue.Enqueue(open_list[0]); // 开始时插入第一个元素
// 接着每次添加新元素时,使用Enqueue并传递新元素和之前的根节点
// 如果你想更新堆排序,可以使用heapify方法:
// heapify(priorityQueue);
// 使用pop_heap等同于从优先队列顶部移除并返回最大值:
// Node topElement = priorityQueue.Peek(); // 返回最大值
// if (priorityQueue.Count > 0) priorityQueue.Dequeue(); // 移除最大值
}
}
```
阅读全文
相关推荐




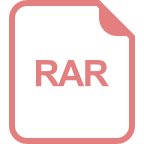
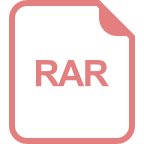
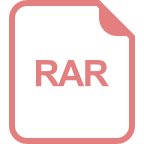
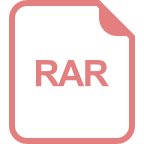



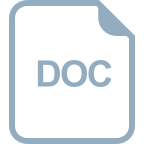






