C语言实现BF匹配算法,即逐个字符比较主串和模式串,若不匹配则主串指针后移一位继续比较,直到找到匹配位置或主串遍历完毕。C语言代码
时间: 2024-11-20 09:45:14 浏览: 18
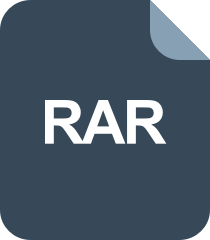
基于BF和KMP的串模式匹配算法设计与实现(C语言).rar-综合文档

BF(Brute Force)匹配算法,也被称为简单暴力匹配,是一种基础的字符串查找算法。在C语言中,我们可以用循环结构来实现这个过程。以下是简单的BF匹配算法在C语言中的代码示例:
```c
#include <stdio.h>
#include <string.h>
// 定义函数用于暴力匹配
int bruteForceMatch(char *text, char *pattern) {
int text_len = strlen(text);
int pattern_len = strlen(pattern);
// 遍历主串
for (int i = 0; i <= text_len - pattern_len; i++) {
// 比较每个字符是否匹配
for (int j = 0; j < pattern_len; j++) {
if (text[i + j] != pattern[j]) {
// 如果不匹配,则跳到下一个位置
break;
}
// 所有字符都匹配则返回当前索引
else if (j == pattern_len - 1)
return i;
}
}
// 如果没有找到匹配,返回-1表示未找到
return -1;
}
int main() {
char text[] = "Hello, world!";
char pattern[] = "world";
int result = bruteForceMatch(text, pattern);
if (result != -1)
printf("Pattern found at position %d\n", result);
else
printf("Pattern not found in the text.\n");
return 0;
}
```
在这个例子中,`bruteForceMatch`函数接收两个字符串参数,分别为主串和模式串。它首先计算它们的长度,然后从头开始逐位比较。如果遇到不匹配的字符,就移动主串指针;如果所有字符都匹配了,说明找到了匹配的位置。
阅读全文
相关推荐
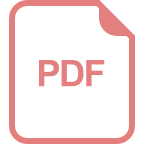
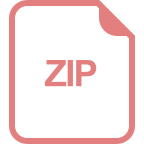
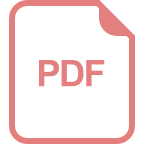
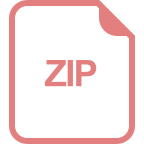
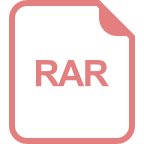
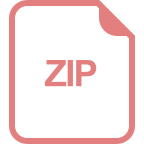
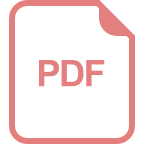
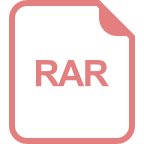
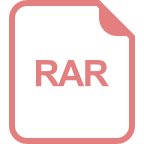
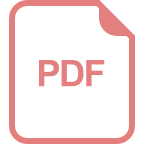
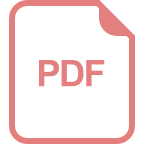
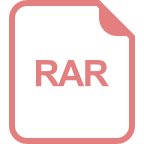
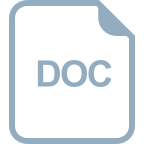
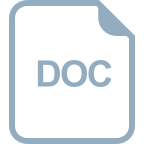