编写简单的python程序存储学生信息。多次输入学号、姓名后,将信息写入 文本文件保存。后续可进行添加。同时可读取文件内容,进行格式化的输出。 要求: 一、尽量定义模块函数(如写函数、读函数、输入函数、输出函数) 二、同时需编写一个主程序循环,提
时间: 2024-03-27 17:40:02 浏览: 26
供给用户选择功能。
好的,这是一个关于Python学生信息存储的问题,我可以帮您解决。以下是代码示例:
```python
# 定义模块函数
def input_student_info():
# 输入学生信息,返回学号和姓名
student_id = input("请输入学生学号:")
student_name = input("请输入学生姓名:")
return student_id, student_name
def write_student_info(student_list, file_path):
# 将学生信息写入文本文件
with open(file_path, "a") as f:
for student in student_list:
f.write(student[0] + "\t" + student[1] + "\n")
def read_student_info(file_path):
# 从文本文件中读取学生信息
student_list = []
with open(file_path, "r") as f:
for line in f.readlines():
student_info = line.strip().split("\t")
student_list.append(student_info)
return student_list
def output_student_info(student_list):
# 格式化输出学生信息
print("{:<10}{:<10}".format("学号", "姓名"))
for student in student_list:
print("{:<10}{:<10}".format(student[0], student[1]))
# 编写主程序循环
file_path = "student_info.txt"
while True:
print("请选择功能:")
print("1. 添加学生信息")
print("2. 查看学生信息")
print("3. 退出程序")
choice = input("请输入选项:")
if choice == "1":
student_list = []
while True:
student_info = input_student_info()
student_list.append(student_info)
add_another = input("是否继续添加?(y/n)")
if add_another.lower() != "y":
break
write_student_info(student_list, file_path)
print("学生信息已添加")
elif choice == "2":
student_list = read_student_info(file_path)
output_student_info(student_list)
elif choice == "3":
print("程序已退出")
break
else:
print("输入有误,请重新输入")
```
以上代码实现了输入、写入、读取、输出学生信息的功能,并提供了一个主程序循环供用户选择功能。用户可以选择添加学生信息、查看学生信息或退出程序。程序会将学生信息写入文本文件并格式化输出。
相关推荐
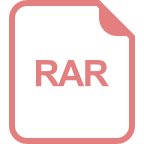
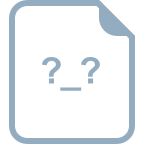
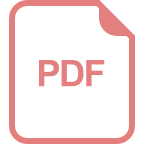














