c++把{ "dd": "sD01", "rel": 2, "rfw": 20, "mc": "D123445A79AF", "de": "20230811" } 追加到已经存在的output.json的文件数组中,不存在文件先创建,存在打开文件追加内容,后关闭文件
时间: 2023-08-28 19:04:24 浏览: 85
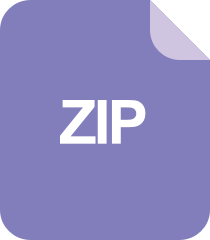
通过C++编程实现文件的创建
要将一个JSON对象追加到已经存在的JSON数组中,你可以读取现有的JSON文件,将其解析为Document对象,然后添加新的JSON对象到数组中,最后将更新后的数组重新写入文件中。以下是一个示例代码:
```cpp
#include <iostream>
#include <fstream>
#include "rapidjson/document.h"
#include "rapidjson/writer.h"
#include "rapidjson/stringbuffer.h"
using namespace rapidjson;
int main() {
// 读取现有的JSON文件
std::ifstream inputFile("output.json");
std::string jsonString((std::istreambuf_iterator<char>(inputFile)), std::istreambuf_iterator<char>());
inputFile.close();
// 解析现有的JSON字符串为Document对象
Document document;
document.Parse(jsonString.c_str());
// 创建要追加的JSON对象
Value object(kObjectType);
object.AddMember("dd", "sD01", document.GetAllocator());
object.AddMember("rel", 2, document.GetAllocator());
object.AddMember("rfw", 20, document.GetAllocator());
object.AddMember("mc", "D123445A79AF", document.GetAllocator());
object.AddMember("de", "20230811", document.GetAllocator());
// 将新的JSON对象添加到现有的数组中
document.PushBack(object, document.GetAllocator());
// 使用Writer将Document转换为字符串
StringBuffer buffer;
Writer<StringBuffer> writer(buffer);
document.Accept(writer);
// 将更新后的JSON字符串写入文件
std::ofstream outputFile("output.json");
outputFile << buffer.GetString();
outputFile.close();
std::cout << "JSON对象已追加到文件中。" << std::endl;
return 0;
}
```
这段代码首先读取现有的JSON文件,并将其解析为一个Document对象。然后,创建要追加的新JSON对象,并将其添加到现有的数组中。接下来,使用Writer将更新后的Document对象转换为字符串,并将其写入同一文件中。
请确保在运行代码之前,已经存在名为"output.json"的JSON文件。如果文件不存在,代码将创建一个新文件。如果文件已存在,代码将打开文件并追加新内容后关闭文件。
阅读全文
相关推荐
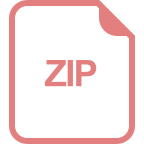
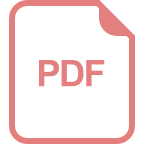
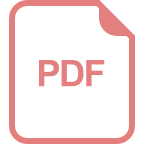
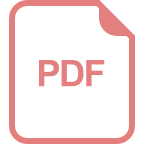
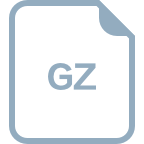
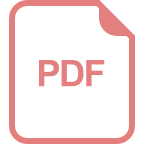
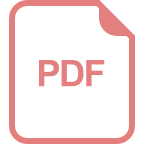
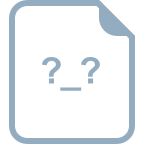
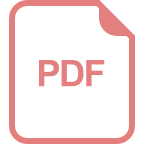
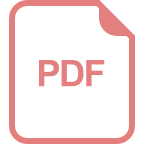
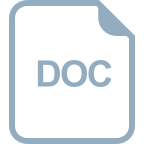
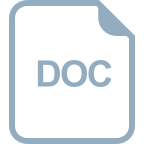
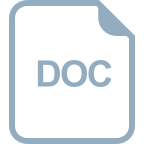
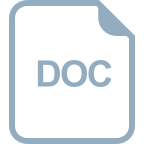